可以进行长按排序的ListView,可指定滑动方向、是否反向、滑动控制器等属性。
相关组件
ReorderableListView基本使用
<br />【children】 : 子组件列表 【List<Widget>】<br />【header】 : 头部组件 【Widget】<br />【padding】 : 内边距 【EdgeInsets】<br />【onReorder】 : 调换时回调 【ReorderCallback】<br />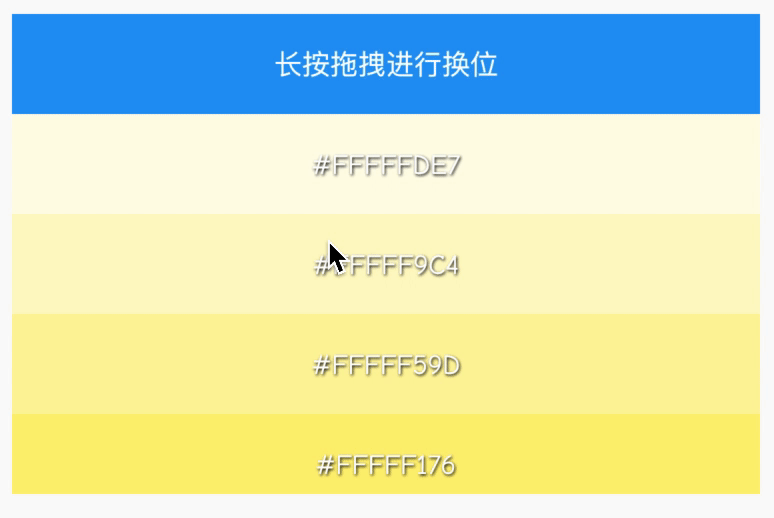
import 'package:flutter/material.dart';
class CustomReorderableListView extends StatefulWidget {
@override
_CustomReorderableListViewState createState() => _CustomReorderableListViewState();
}
class _CustomReorderableListViewState extends State<CustomReorderableListView> {
var data = <Color>[
Colors.yellow[50],
Colors.yellow[100],
Colors.yellow[200],
Colors.yellow[300],
Colors.yellow[400],
Colors.yellow[500],
Colors.yellow[600],
Colors.yellow[700],
Colors.yellow[800],
Colors.yellow[900],
];
@override
Widget build(BuildContext context) {
return Container(
height: 250,
child: ReorderableListView(
padding: EdgeInsets.all(10),
header: Container(
color: Colors.blue,
alignment: Alignment.center,
height: 50,
child: Text('长按拖拽进行换位',style: TextStyle(color: Colors.white),)),
onReorder: _handleReorder,
children: data.map((color) => _buildItem(color)).toList(),
),
);
}
void _handleReorder(int oldIndex, int newIndex) {
if (oldIndex < newIndex) {
newIndex -= 1;
}
setState(() {
final element = data.removeAt(oldIndex);
data.insert(newIndex, element);
});
}
Widget _buildItem(Color color) {
return Container(
key: ValueKey(color) ,
alignment: Alignment.center,
height: 50,
color: color,
child: Text(
colorString(color),
style: TextStyle(color: Colors.white, shadows: [
Shadow(color: Colors.black, offset: Offset(.5, .5), blurRadius: 2)
]),
),
);
}
String colorString(Color color) =>
"#${color.value.toRadixString(16).padLeft(8, '0').toUpperCase()}";
}
ReorderableListView滑动方向
<br />【scrollDirection】 : 滑动方向 【Axis】<br />【reverse】 : 是否反向 【bool】<br />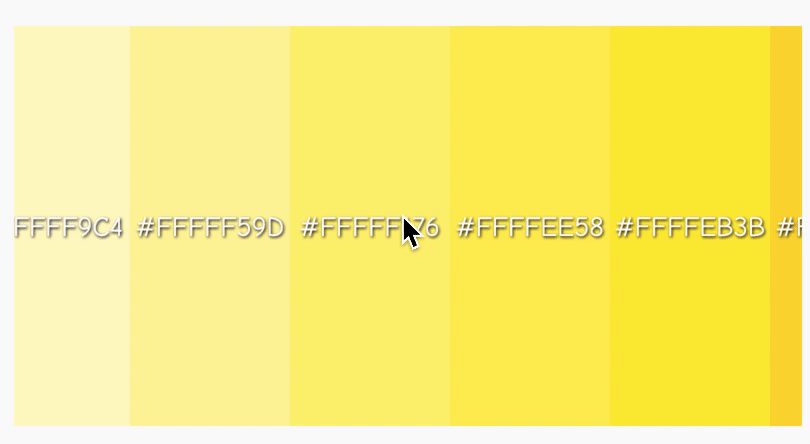
import 'package:flutter/material.dart';
class DirectionReorderableListView extends StatefulWidget {
@override
_DirectionReorderableListViewState createState() => _DirectionReorderableListViewState();
}
class _DirectionReorderableListViewState extends State<DirectionReorderableListView> {
var data = <Color>[
Colors.yellow[50],
Colors.yellow[100],
Colors.yellow[200],
Colors.yellow[300],
Colors.yellow[400],
Colors.yellow[500],
Colors.yellow[600],
Colors.yellow[700],
Colors.yellow[800],
Colors.yellow[900],
];
@override
Widget build(BuildContext context) {
return Container(
height: 200,
child: ReorderableListView(
scrollDirection: Axis.horizontal,
reverse: false,
onReorder: _handleReorder,
children: data.map((color) => _buildItem(color)).toList(),
),
);
}
void _handleReorder(int oldIndex, int newIndex) {
if (oldIndex < newIndex) {
newIndex -= 1;
}
setState(() {
final element = data.removeAt(oldIndex);
data.insert(newIndex, element);
});
}
Widget _buildItem(Color color) {
return Container(
key: ValueKey(color) ,
alignment: Alignment.center,
width: 80,
color: color,
child: Text(
colorString(color),
style: TextStyle(color: Colors.white, shadows: [
Shadow(color: Colors.black, offset: Offset(.5, .5), blurRadius: 2)
]),
),
);
}
String colorString(Color color) =>
"#${color.value.toRadixString(16).padLeft(8, '0').toUpperCase()}";
}