可容纳多个组件,按照指定方向依次排布,可以很方便处理孩子的间距,当越界时可以自动换行。拥有主轴和交叉轴的对齐方式,比较灵活。
相关组件
Wrap的基础用法
<br />【children】 : 组件列表 【List<Widget>】<br />【spacing】 : 主轴条目间距 【double】<br />【runSpacing】 : 交叉轴条目间距 【double】<br />【direction】 : 主轴对齐 【Axis】<br />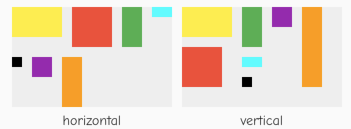
import 'package:flutter/material.dart';
class DirectionWrap extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Wrap(
children: Axis.values
.map((mode) => Column(children: <Widget>[
Container(
margin: EdgeInsets.all(5),
width: 160,
height: 100,
color: Colors.grey.withAlpha(33),
child: _buildItem(mode)),
Text(mode.toString().split('.')[1])
]))
.toList());
}
final yellowBox = Container(
color: Colors.yellow,
height: 30,
width: 50,
);
final redBox = Container(
color: Colors.red,
height: 40,
width: 40,
);
final greenBox = Container(
color: Colors.green,
height: 40,
width: 20,
);
final blackBox = Container(
color: Colors.black,
height: 10,
width: 10,
);
final purpleBox = Container(
color: Colors.purple,
height: 20,
width: 20,
);
final orangeBox = Container(
color: Colors.orange,
height: 80,
width: 20,
);
final cyanBox = Container(
color: Colors.cyanAccent,
height: 10,
width: 20,
);
_buildItem(mode) => Wrap(
direction: mode,
runSpacing: 10,
spacing: 10,
children: <Widget>[
yellowBox, redBox, greenBox, cyanBox,
blackBox, purpleBox, orangeBox,
],
);
}
Wrap的alignment属性
<br />【alignment】 : 主轴对齐 【WrapAlignment】<br />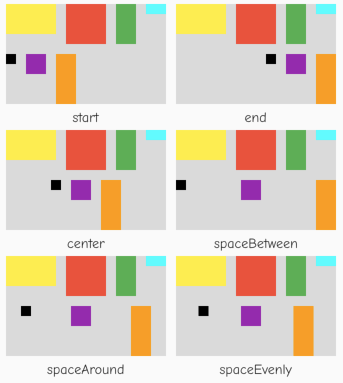
import 'package:flutter/material.dart';
class WrapAlignmentWrap extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Wrap(
children: WrapAlignment.values
.map((mode) => Column(children: <Widget>[
Container(
margin: EdgeInsets.all(5),
width: 160,
height: 100,
color: Colors.grey.withAlpha(88),
child: _buildItem(mode)),
Text(mode.toString().split('.')[1])
]))
.toList());
}
final yellowBox = Container(
color: Colors.yellow,
height: 30,
width: 50,
);
final redBox = Container(
color: Colors.red,
height: 40,
width: 40,
);
final greenBox = Container(
color: Colors.green,
height: 40,
width: 20,
);
final blackBox = Container(
color: Colors.black,
height: 10,
width: 10,
);
final purpleBox = Container(
color: Colors.purple,
height: 20,
width: 20,
);
final orangeBox = Container(
color: Colors.orange,
height: 80,
width: 20,
);
final cyanBox = Container(
color: Colors.cyanAccent,
height: 10,
width: 20,
);
_buildItem(mode) => Wrap(
alignment: mode,
runSpacing: 10,
spacing: 10,
children: <Widget>[
yellowBox, redBox,
greenBox, cyanBox,
blackBox, purpleBox,
orangeBox,
],
);
}
Wrap的crossAxisAlignment属性
<br />【crossAxisAlignment】 : 交叉轴对齐 【CrossAxisAlignment】<br />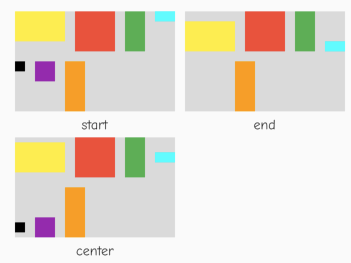
import 'package:flutter/material.dart';
class CrossAxisAlignmentWrap extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Wrap(
children: WrapCrossAlignment.values
.map((mode) => Column(children: <Widget>[
Container(
margin: EdgeInsets.all(5),
width: 160,
height: 100,
color: Colors.grey.withAlpha(88),
child: _buildItem(mode)),
Text(mode.toString().split('.')[1])
]))
.toList());
}
final yellowBox = Container(
color: Colors.yellow,
height: 30,
width: 50,
);
final redBox = Container(
color: Colors.red,
height: 40,
width: 40,
);
final greenBox = Container(
color: Colors.green,
height: 40,
width: 20,
);
final blackBox = Container(
color: Colors.black,
height: 10,
width: 10,
);
final purpleBox = Container(
color: Colors.purple,
height: 20,
width: 20,
);
final orangeBox = Container(
color: Colors.orange,
height: 80,
width: 20,
);
final cyanBox = Container(
color: Colors.cyanAccent,
height: 10,
width: 20,
);
_buildItem(mode) => Wrap(
crossAxisAlignment: mode,
runSpacing: 10,
spacing: 10,
children: <Widget>[
yellowBox, redBox,
greenBox, cyanBox,
blackBox, purpleBox,
orangeBox,
],
);
}
Wrap的textDirection属性
【textDirection】 : 文字方向 【TextDirection】
import 'package:flutter/material.dart';
class TextDirectionWrap extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Wrap(
children: TextDirection.values
.map((mode) => Column(children: <Widget>[
Container(
margin: EdgeInsets.all(5),
width: 160,
height: 100,
color: Colors.grey.withAlpha(88),
child: _buildItem(mode)),
Text(mode.toString().split('.')[1])
]))
.toList());
}
final yellowBox = Container(
color: Colors.yellow,
height: 30,
width: 50,
);
final redBox = Container(
color: Colors.red,
height: 40,
width: 40,
);
final greenBox = Container(
color: Colors.green,
height: 40,
width: 20,
);
final blackBox = Container(
color: Colors.black,
height: 10,
width: 10,
);
final purpleBox = Container(
color: Colors.purple,
height: 20,
width: 20,
);
final orangeBox = Container(
color: Colors.orange,
height: 80,
width: 20,
);
final cyanBox = Container(
color: Colors.cyanAccent,
height: 10,
width: 20,
);
_buildItem(mode) => Wrap(
textDirection: mode,
runSpacing: 10,
spacing: 10,
children: <Widget>[
yellowBox, redBox, greenBox, cyanBox,
blackBox, purpleBox, orangeBox,
],
);
}
Wrap的verticalDirection属性
<br />【verticalDirection】 : 竖直方向 【VerticalDirection】<br />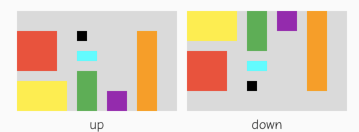
import 'package:flutter/material.dart';
class VerticalDirectionWrap extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Wrap(
children: VerticalDirection.values
.map((mode) => Column(children: <Widget>[
Container(
margin: EdgeInsets.all(5),
width: 160,
height: 100,
color: Colors.grey.withAlpha(88),
child: _buildItem(mode)),
Text(mode.toString().split('.')[1])
]))
.toList());
}
final yellowBox = Container(
color: Colors.yellow,
height: 30,
width: 50,
);
final redBox = Container(
color: Colors.red,
height: 40,
width: 40,
);
final greenBox = Container(
color: Colors.green,
height: 40,
width: 20,
);
final blackBox = Container(
color: Colors.black,
height: 10,
width: 10,
);
final purpleBox = Container(
color: Colors.purple,
height: 20,
width: 20,
);
final orangeBox = Container(
color: Colors.orange,
height: 80,
width: 20,
);
final cyanBox = Container(
color: Colors.cyanAccent,
height: 10,
width: 20,
);
_buildItem(mode) => Wrap(
verticalDirection: mode,
direction: Axis.vertical,
runSpacing: 10,
spacing: 10,
children: <Widget>[
yellowBox, redBox, greenBox, cyanBox,
blackBox, purpleBox, orangeBox,
],
);
}