组件事件的监听器,可接受按下、松开、移动、取消等事件。较GestureDetector比较原始,可获取的信息也更多。
相关组件
GestureDetector基本事件
<br />【child】 : 子组件 【Widget】<br />【onTap】 : 点击事件 【Function()】<br />【onDoubleTap】 : 双击事件 【Function()】<br />【onLongPress】 : 长按事件 【Function()】<br />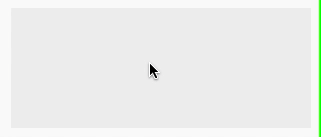
import 'package:flutter/material.dart';
class CustomGestureDetector extends StatefulWidget {
@override
_CustomGestureDetectorState createState() => _CustomGestureDetectorState();
}
class _CustomGestureDetectorState extends State<CustomGestureDetector> {
var _info = '';
@override
Widget build(BuildContext context) {
return GestureDetector(
onTap: () => setState(() => _info = 'onTap'),
onDoubleTap: () => setState(() => _info = 'onDoubleTap'),
onLongPress: () => setState(() => _info = 'onLongPress'),
child: Container(
alignment: Alignment.center,
width: 300,
height: 300 * 0.4,
color: Colors.grey.withAlpha(33),
child: Text(
_info,
style: TextStyle(fontSize: 18, color: Colors.blue),
),
),
);
}
}
GestureDetector详情信息
【onTapDown】 : 按下回调 【Function(TapDownDetails)】
【onTapUp】 : 子组件 【Function(TapUpDetails)】
【onTapCancel】 : 点击取消 【Function()】
import 'package:flutter/material.dart';
class TapGestureDetector extends StatefulWidget {
@override
_TapGestureDetectorState createState() => _TapGestureDetectorState();
}
class _TapGestureDetectorState extends State<TapGestureDetector> {
var _info = '';
@override
Widget build(BuildContext context) {
return GestureDetector(
onTapDown: (detail) => setState(() => _info =
'onTapDown:\n相对落点:${detail.localPosition}\n绝对落点:${detail.globalPosition}'),
onTapUp: (detail) => setState(() => _info =
'onTapUp:\n相对落点:${detail.localPosition}\n绝对落点:${detail.globalPosition}'),
onTapCancel: () => setState(() => _info = 'onTapCancel'),
child: Container(
alignment: Alignment.center,
width: 300,
height: 300 * 0.618,
color: Colors.grey.withAlpha(33),
child: Text(
_info,
style: TextStyle(fontSize: 18, color: Colors.blue),
),
),
);
}
}
GestureDetector的Pan事件
【onPanDown】 : 按下回调 【Function(DragDownDetails)】
【onPanEnd】 : 拖动结束 【Function(DragEndDetails)】
【onPanStart】 : 开始拖动 【Function(DragStartDetails)】
【onPanUpdate】 : 拖动更新 【Function(TapUpDetails)】
【onPanCancel】 : 拖动取消 【Function()】
import 'package:flutter/material.dart';
class PanGestureDetector extends StatefulWidget {
@override
_PanGestureDetectorState createState() => _PanGestureDetectorState();
}
class _PanGestureDetectorState extends State<PanGestureDetector> {
var _info = '';
@override
Widget build(BuildContext context) {
return GestureDetector(
onPanDown: (detail) => setState(() => _info =
'onPanDown:\n相对落点:${detail.localPosition}\n绝对落点:${detail.globalPosition}'),
onPanEnd: (detail) => setState(() => _info =
'onPanEnd:\n初速度:${detail.primaryVelocity}\n最终速度:${detail.velocity}'),
onPanUpdate: (detail) => setState(() => _info =
'onPanUpdate:\n相对落点:${detail.localPosition}\n绝对落点:${detail.globalPosition}'),
onPanStart: (detail) => setState(() => _info =
'onPanStart:\n相对落点:${detail.localPosition}\n绝对落点:${detail.globalPosition}'),
onPanCancel: () => setState(() => _info = 'onTapCancel'),
child: SingleChildScrollView(
child: Container(
alignment: Alignment.center,
width: 300,
height: 300 * 0.618,
color: Colors.grey.withAlpha(33),
child: Text(
_info,
style: TextStyle(fontSize: 18, color: Colors.blue),
),
),
),
);
}
}