通常用于SliverAppBar中的可伸展区域,可指定标题、标题间距、背景、折叠模式等。
相关组件
FlexibleSpaceBar基本使用
<br />【title】 : 标题组件 【Widget】<br />【titlePadding】 : 标题间距 【EdgeInsetsGeometry】<br />【collapseMode】 : 折叠模式 【CollapseMode】<br />【stretchModes】 : 延伸模式 【List<StretchMode>】<br />【background】 : 背景组件 【Widget】<br />【centerTitle】 : 是否居中 【bool】<br />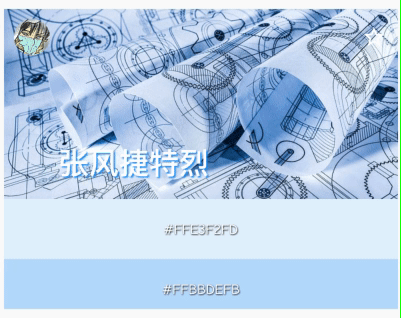
import 'package:flutter/material.dart';
class FlexibleSpaceBarDemo extends StatelessWidget {
final data = <Color>[
Colors.blue[50],
Colors.blue[100],
Colors.blue[200],
Colors.blue[300],
Colors.blue[400],
Colors.blue[500],
Colors.blue[600],
Colors.blue[700],
Colors.blue[800],
Colors.blue[900],
];
@override
Widget build(BuildContext context) {
return
Container(
height: 300,
child: CustomScrollView(
slivers: <Widget>[
_buildSliverAppBar(),
_buildSliverFixedExtentList()
],
),
);
}
Widget _buildSliverAppBar() {
return SliverAppBar(
expandedHeight: 190.0,
leading: _buildLeading(),
actions: _buildActions(),
pinned: true,
backgroundColor: Colors.blue,
flexibleSpace: FlexibleSpaceBar(//伸展处布局
centerTitle: false,
title: Text('张风捷特烈',style: TextStyle(shadows: [
Shadow(color: Colors.blue, offset: Offset(1, 1), blurRadius: 2)
]),),
titlePadding: EdgeInsets.only(left: 55, bottom: 15), //标题边距
collapseMode: CollapseMode.parallax, //视差效果
stretchModes: [StretchMode.blurBackground,StretchMode.zoomBackground],
background: Image.asset(
"assets/images/caver.jpeg",
fit: BoxFit.cover,
),
),
);
}
Widget _buildLeading() => Container(
margin: EdgeInsets.all(10),
child: Image.asset('assets/images/icon_head.png'));
List<Widget> _buildActions() => <Widget>[
IconButton(
onPressed: () {},
icon: Icon(
Icons.star_border,
color: Colors.white,
),
)
];
Widget _buildSliverFixedExtentList() => SliverFixedExtentList(
itemExtent: 60,
delegate: SliverChildBuilderDelegate(
(_, int index) => Container(
alignment: Alignment.center,
width: 100,
height: 50,
color: data[index],
child: Text(
colorString(data[index]),
style: TextStyle(color: Colors.white, shadows: [
Shadow(
color: Colors.black,
offset: Offset(.5, .5),
blurRadius: 2)
]),
),
),
childCount: data.length),
);
String colorString(Color color) =>
"#${color.value.toRadixString(16).padLeft(8, '0').toUpperCase()}";
}