实际上就是渲染了一个 SVG ,自定义的程度会很高
https://github.com/tanem/react-svg
https://www.npmjs.com/package/react-svg
https://zhuanlan.zhihu.com/p/33918621
import React from 'react'
import { ReactSVG } from 'react-svg'
import userSvg from './user.svg'
function App() {
function beforeInjection(error, svg) {
if (error) return;
svg.classList.add('svgStyle')
svg.setAttribute('style', 'fill: red')
}
return (
<div>
<ReactSVG src={userSvg} />
<ReactSVG
src={userSvg}
className="wrapper"
onClick={() => {}}
wrapper={'a'}
beforeInjection={beforeInjection}
/>
</div>
)
}
css修改 svg样式
.wrapper svg {
width: 100px;
height: 100px;
}
.wrapper path {
fill: red;
stroke: red;
width: 80px;
height: 80px;
}
批量导入 svg
webpack的 require.context 函数可以获取一个上下文;
从而实现工程自动化(遍历文件夹的文件,从中获取指定文件,自动导入模块;
如果一个文件夹中的模块,需要频繁引用时可以使用 require.context 一次性引入
import React from 'react'
import { ReactSVG } from 'react-svg'
function SvgIcon(context) {
const files = context.keys();
if (!files.length) return {};
return files
.reduce((prev, path, index) => {
const svgName = path.replace(/\.svg$/, '');
prev[svgName] = (props) => {
const svgPath = files.map(context)[index];
return (
<ReactSvg
src={svgPath}
{...props}
/>
)
}
return prev;
}, {})
}
const paths = require.context('./icons', false, /\.svg$/);
export default SvgIcon(paths)
require.context(dirname, useSubdirectories, RegExp)require.context('./icons', false, /\.svg$/)
dirname | String | 需要读取模块的文件的所在目录 |
---|---|---|
useSubdirectories | Boolean | 是否遍历子目录 |
RegExp | RegExp | 匹配的规则(正则表达式) |
require.context 返回结果
属性 | 类型 | 说明 |
---|---|---|
resolve | Function | 接受一个参数request,request为文件夹下面匹配文件的相对路径, 返回这个匹配文件相对于整个工程的相对路径 |
keys | Function | 返回一个数组,由匹配成功的文件所组成的数组 |
id | String | 执行环境的 id |
字符串导入 svg
缺点:不能修改颜色,其实就是直接用 img 加载了 svg 文件
import logo from './logo.svg';
<img src={logo} />
import 导入 svg
实际上就是渲染了一个 SVG ,自定义的程度会很高,使用限制
- react-scripts 版本要大于 @2.0.0
- react 版本大于 @16.3.0 ```jsx import { ReactComponent as Logo } from ‘./logo.svg’
import { default as logo } from ‘./logo.svg’;
- ReactComponent是必需的魔法字符串
- Logo现在是一个组件
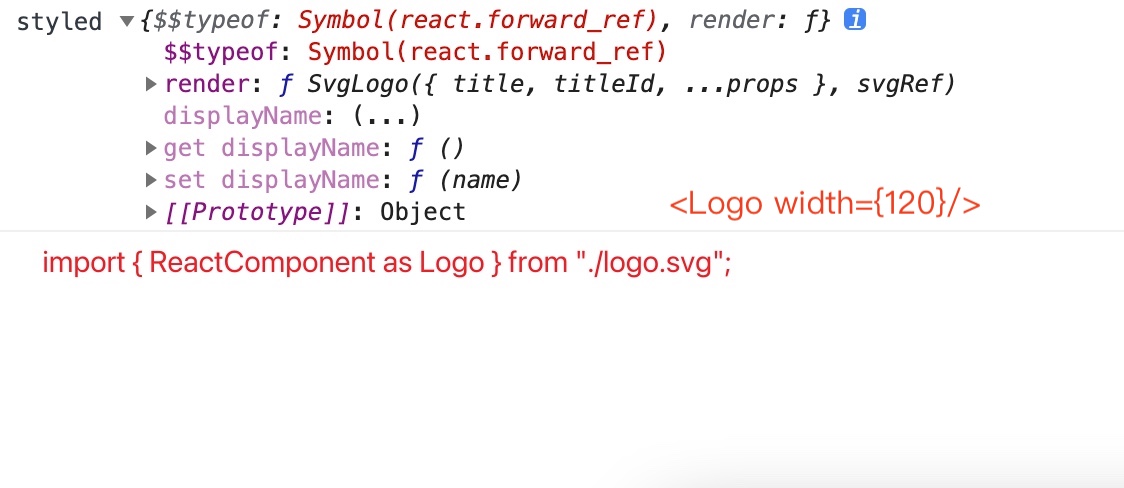
<a name="lA6kC"></a>
## @svgr/webpack
[https://react-svgr.com/docs/webpack/](https://react-svgr.com/docs/webpack/)<br />将 svg图标作为 React组件导入
```bash
yarn add babel-plugin-named-asset-import
yarn add @svgr/webpack --dev
create-react-app 配置 @svgr/webpack
create-react-app 进行 eject , 需要给 webpack 加入配置
[
require.resolve('babel-plugin-named-asset-import'),
{
loaderMap: {
svg: {
ReactComponent: '@svgr/webpack?-svgo,+ref![path]',
},
},
},
],
或
{
test: /\.svg$/,
use: ['@svgr/webpack'],
}
如果svg文件的存放是和jpg以及png等图片同一目录的,
- 则需要在url-loader或file-loader的配置中使用exclude去除svg的匹配,
- 因为如果不去除会导致svg经过hash指纹重命名之后,loader找不到svg文件,进而导致报错
umijs chainWebpack配置
chainWebpack(config) {
config.module
.rule('svg')
.test(/\.svg$/)
.use('svgr')
.loader('@svgr/webpack')
// 图片配置
config.module
.rule('image')
.test(/\.(png|gif|jpe?g|webp)$/)
.use('url-loader')
.loader('url-loader')
.options({
limit: 10000,
name: '[name].[hash:7].[ext]',
})
}
@craco/craco @svgr配置
安装 @svgr/webpack
module.exports = {
plugins: [],
webpack: {
configure: (config, { env, paths }) => {
config.module
.rules
.push({
test: /\.svg$/,
use: ["@svgr/webpack"]
});
return config;
}
}
}
ts项目配置 svg
在项目根目录 加一个 custom.d.ts 文件声明
import * as React from 'react';
declare interface SvgrComponent extends React.StatelessComponent<React.SVGAttributes<SVGElement>> {}
declare module '*.svg' {
const content: SvgrComponent
export default content
}
// 其他语法
declare module '*.svg' {
import React = require('react');
export const ReactComponent: React.FunctionComponent<React.SVGProps<SVGSVGElement>>;
const src: string;
export default src;
}
然后在 tsconfig.json 中加入
"files": ["custom.d.ts"]
https://blog.csdn.net/baidu_39067385/article/details/106311737
https://stackoverflow.com/questions/60074103/adding-svgr-to-create-react-app-via-craco
https://create-react-app.dev/docs/adding-images-fonts-and-files/#adding-svgs
https://www.pluralsight.com/guides/how-to-load-svg-with-react-and-webpack