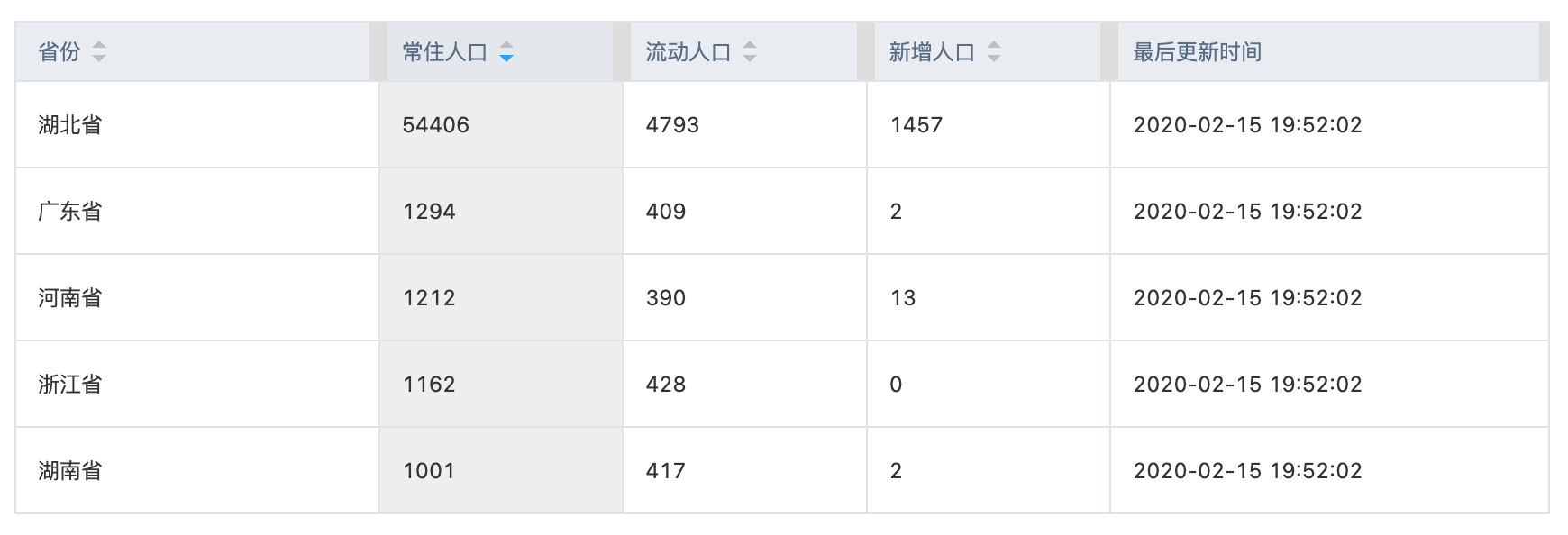
import { BaseTable, useTablePipeline, features } from 'ali-react-table';
import { Table } from 'antd';
const dataSource = [
{ prov: '湖北省', settled: 54406, flow: 4793, add: 1457, t: '2020-02-15 19:52:02' },
{ prov: '广东省', settled: 1294, flow: 409, add: 2, t: '2020-02-15 19:52:02' },
{ prov: '河南省', settled: 1212, flow: 390, add: 13, t: '2020-02-15 19:52:02' },
{ prov: '浙江省', settled: 1162, flow: 428, add: 0, t: '2020-02-15 19:52:02' },
{ prov: '湖南省', settled: 1001, flow: 417, add: 2, t: '2020-02-15 19:52:02' },
];
const columns = [
{ code: 'prov', name: '省份', width: 150, features: { sortable: true } },
{ code: 'confirmed', name: '常住人口', width: 100, features: { sortable: true } },
{ code: 'flow', name: '流动人口', width: 100, features: { sortable: true } },
{ code: 'add', name: '新增人口', width: 100, features: { sortable: true } },
{ code: 't', name: '最后更新时间', width: 180 },
];
function App() {
// const pipeline = useTablePipeline({ components: { Table } })
const pipeline = useTablePipeline()
.input({ dataSource, columns })
.use(
features.sort({
mode: 'single',
// 默认排序的列
defaultSorts: [{ code: 'settled', order: 'desc' }],
highlightColumnWhenActive: true,
})
)
.use(
features.columnResize({
fallbackSize: 120,
handleBackground: '#ddd',
handleHoverBackground: '#aaa',
handleActiveBackground: '#89bff7',
}),
)
return (
<div style={{ padding: 24 }}>
<BaseTable {...pipeline.getProps()} />
</div>
);
}
export default App;
// Table基本用法
<BaseTable dataSource={dataSource} columns={columns} />