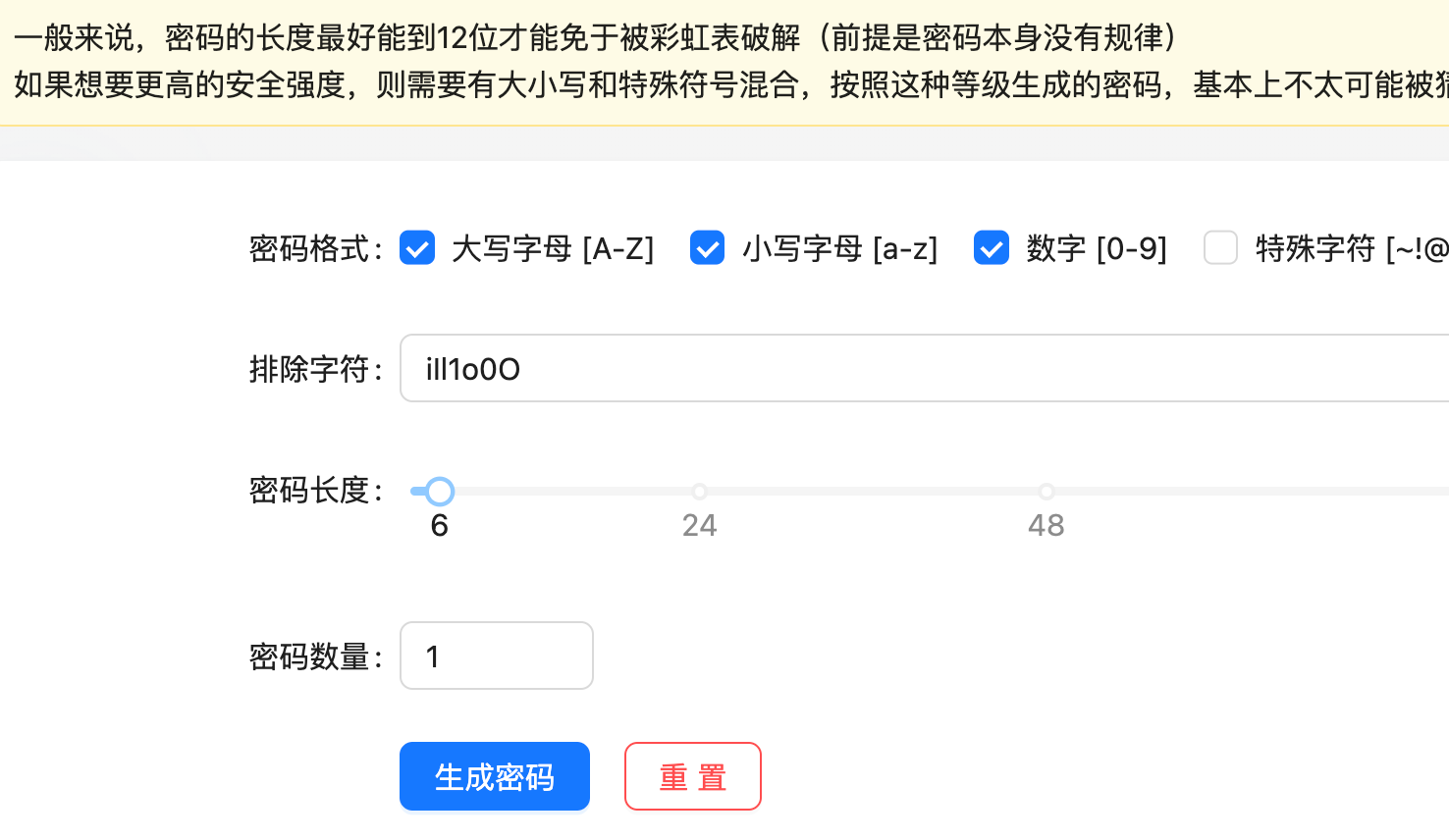
import React, { useState } from 'react';
import { PageContainer } from '@ant-design/pro-components';
import { Alert, Card, Col, Row } from 'antd';
import { FormGroup } from '@/components';
import { generatePassword } from '@/utils'
import List from './List'
const initialValues = {
ignore: 'iIl1o0O',
length: 6,
type: [1, 2, 3],
total: 1,
};
const GeneratePassword: React.FC = () => {
const [state, setState] = useState([]);
const fields = [
{
name: 'type',
label: '密码格式',
component: 'CheckboxGroup',
props: {
options: [
{ label: '大写字母 [A-Z]', value: 1 },
{ label: '小写字母 [a-z]', value: 2 },
{ label: '数字 [0-9]', value: 3 },
],
},
},
{
name: 'ignore',
label: '排除字符',
component: 'Input',
props: {},
},
{
label: ' ',
colon: false,
component: 'Title',
// noStyle: true,
props: { children: '密码强度', level: 5 },
},
{
name: 'length',
label: '密码长度',
component: 'Slider',
props: {
min: 4,
max: 128,
step: 2,
marks: {
6: 6,
24: 24,
48: 48,
80: 80,
128: 128,
}
},
},
{
name: 'total',
label: '密码数量',
component: 'InputNumber',
props: {
defaultValue: 1,
},
},
];
function onFinish(values) {
const passwords = generatePassword(values);
setState(passwords);
console.log(60, values);
}
return (
<PageContainer>
<Card bordered={false}>
<FormGroup
initialValues={initialValues}
fields={fields}
col={24}
style={{ width: '50%' }}
okButtonProps={{
children: '生成密码',
}}
cancelButtonProps={{ danger: true }}
confirmLoading={false}
onFinish={onFinish}
onReset={() => setState([])}
mode='submit'
/>
</Card>
</PageContainer>
);
};
export default GeneratePassword;