分片渲染一万条数据
根据数据大小划分,每次加载固定的条数,例如
- 一万条数据,每次渲染 100条;
- 然后再渲染 100条,递归实现分片渲染;
- 缺点
- 页面DOM会100条,100条的递增,页面会有抖动
- DOM渲染太多,一万条数据,页面滚动卡顿
递归实现分片渲染
const container = document.querySelector('#root')
const total = 10000;
const bufferSize = 100; // 每次渲染 100条
let index = 0;
let id = 0;
let timer = Date.now();
loadDom()
function loadDom() {
index += bufferSize;
if(index > total) return;
// 分片渲染,定时器是一个宏任务,会等待 GUI线程渲染完成后再执行
setTimeout(() => {
for(let i = 0; i< bufferSize; i++) {
const el = document.createElement('p');
el.innerHTML = ++id;
container.appendChild(el);
}
// 10000条数据的执行时间
console.log('JS-render100',Date.now() - timer)
loadDom() // 递归
}, 0)
}
// 同步代码先执行
console.log('JS-render',Date.now() - timer)
// 渲染DOM时间
setTimeout(() => {
console.log('DOM-render',Date.now() - timer)
}, 0)
分片渲染优化
- requestAnimationFrame代替 setTimeout
- document.createDocumentFragment 代替 document.createElement ```jsx const container = document.querySelector(‘#root’) const total = 10000; const bufferSize = 100; let index = 0; let id = 0; let timer = Date.now();
loadDom() function loadDom() { index += bufferSize; if(index > total) return;
const fragment = document.createDocumentFragment(); requestAnimationFrame(() => { for(let i = 0; i< bufferSize; i++) { const el = document.createElement(‘p’); el.innerHTML = ++id; fragment.appendChild(el); } container.appendChild(fragment); // 100条数据的执行时间 console.log(‘JS-render100’,Date.now() - timer) loadDom() // 递归 }) }
// 渲染DOM时间 setTimeout(() => { console.log(‘DOM-render’,Date.now() - timer) }, 0)
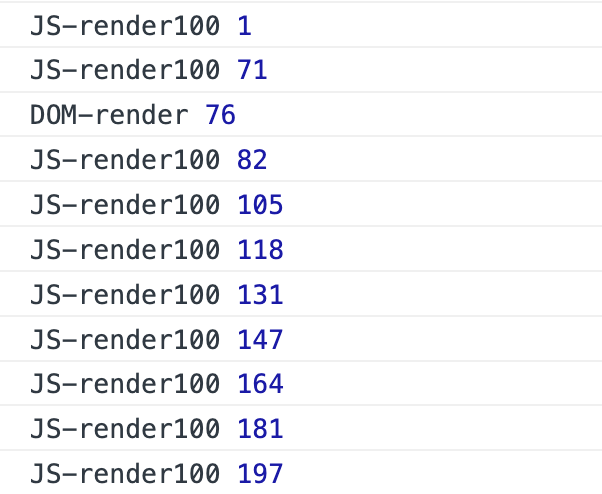
<a name="sfs8N"></a>
## 一次性渲染一万条数据
页面渲染1万条 DOM
- JS渲染生成一万条数据,耗时 95ms
- DOM渲染到页面,耗时 可以646ms
- 缺点
- 页面 loading时间长,白屏时间长
- DOM渲染太多,一万条数据,页面滚动卡顿
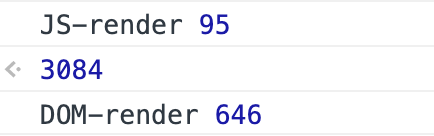
```jsx
const container = document.querySelector('#root')
const total = 10000;
const timer = Date.now();
for(let i = 0; i< total; i++) {
const el = document.createElement('p');
el.innerHTML = i;
container.appendChild(el);
}
// 10000条数据的执行时间
console.log('JS-render', Date.now() - timer)
// 渲染DOM时间
setTimeout(() => {
console.log('DOM-render', Date.now() - timer)
}, 0)