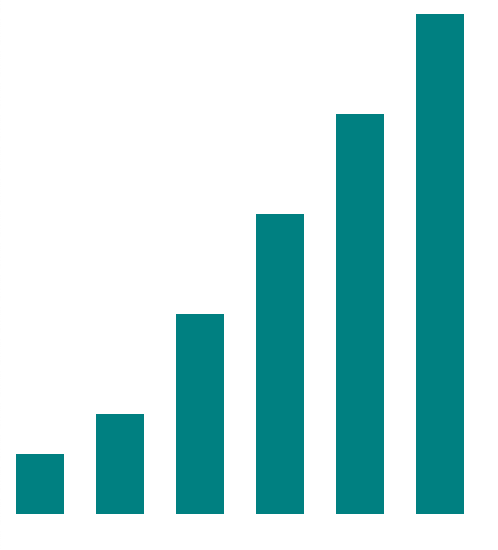
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<title>testD3-3-drawingDivBar</title>
<style>
.box {
border: 1px solid #ddd;
}
.bar {
display: inline-block;
width: 24px;
background-color: teal;
}
</style>
<script src="https://cdn.bootcdn.net/ajax/libs/d3/7.3.0/d3.js"></script>
</head>
<body>
<div class="box"></div>
<script>
const dataset = [3, 5, 10, 15, 20, 25];
const gutter = 16;
d3.select(".box")
.selectAll('.bar')
.data(dataset)
.enter()
.append("div")
.attr("class", "bar")
.style("height", (d) => `${d * 10}px`)
.style('margin-right', `${gutter}px`)
</script>
</body>
</html>
柱状图
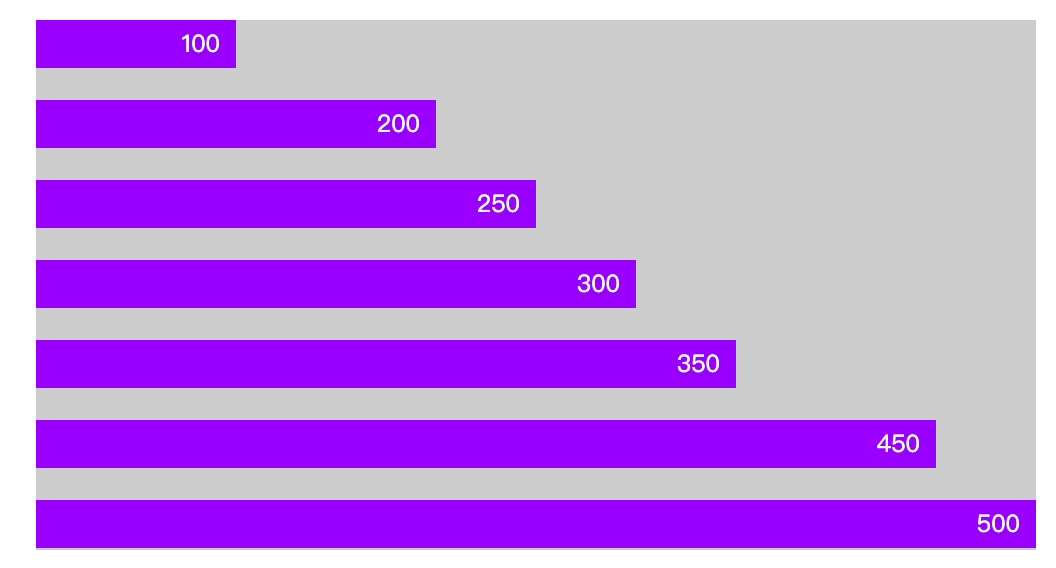
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>d3</title>
<style>
* {
box-sizing: border-box;
}
body {
width: 960px;
margin: 32px auto;
}
.bar {
width: 500px;
height: 265px;
background: rgba(0, 0, 0, 0.2);
position: relative;
}
p {
position: absolute;
margin: 0;
padding-right: 8px;
line-height: 24px;
text-align: right;
color: #fff;
font-size: 12px;
}
</style>
<script src="https://cdn.bootcdn.net/ajax/libs/d3/7.3.0/d3.js"></script>
</head>
<body>
<div class="bar"></div>
<script>
const dataset = [100, 200, 250, 300, 350, 450, 500];
const [width, height] = [16, 24]
const root = d3.select('.bar')
.selectAll('p')
.data(dataset)
.enter().append('p')
.style('left', 0)
.style('top', (d, i) => {
console.log('d', d, i)
return `${i * (width + height)}px`;
})
.style('width', (d, i) => {
return `${d}px`;
})
.style('height', `${height}px`)
.style('background', '#90f')
.text(d => d);
</script>
</body>
</html>