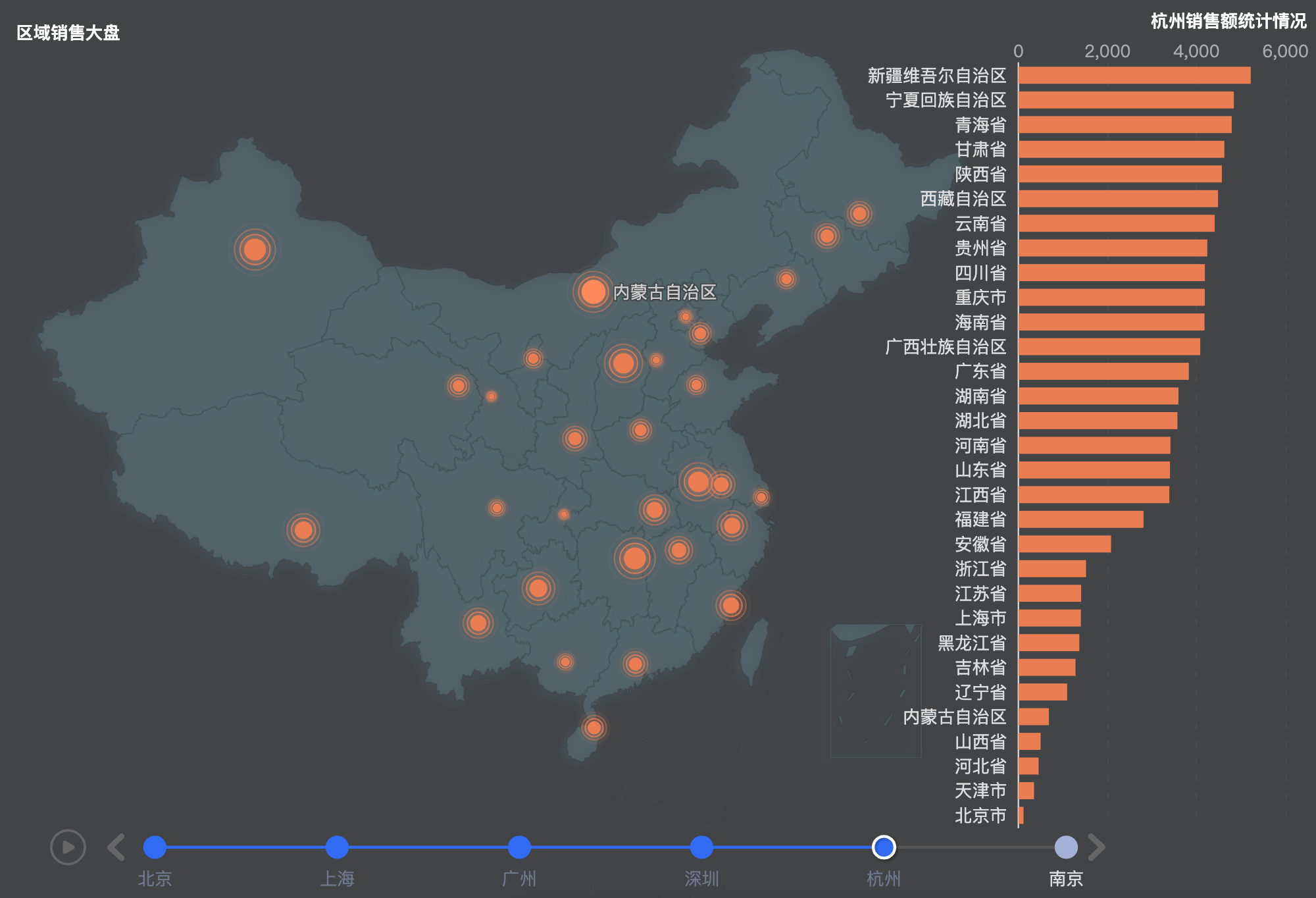
baselineOption
import * as echarts from 'echarts';
import {chinaJson} from '@/components/ECharts/Map';
import {getData, colors, provinces, convertData} from './_data';
import {geo, timeline, baseOption} from './config';
const cities = ['北京', '上海', '广州', '深圳', '杭州', '南京'];
const mapData = cities.map((it, i) => []);
const series = {
data1: getData(10, 6000),
data2: getData(10, 7000),
data3: getData(10, 8000),
data4: getData(10, 9000),
data5: getData(100, 6000),
data6: getData(100, 3000),
}
function chinaOption() {
echarts.registerMap('china', chinaJson);
// 柱图数据 [[], [], [], [], [], []]
provinces.forEach((item, index) => {
const key = item.name;
mapData.forEach((child, i) => {
const {value} = series[`data${i + 1}`].find(it => it.name === key) || {};
child.push({name: key, value})
child.sort((a, b) => a.value - b.value)
})
});
// 柱图 X轴数据 [[], [], [], [], [], []]
// const xdata = cities.map((it, i) => {
// return mapData[i].map(it => it.name);
// });
// 柱状图数据
const barData = cities.map((it, i) => {
return mapData[i].map(it => it.value);
});
const config = {
timeline: timeline(cities),
baseOption: {
...baseOption(provinces),
geo,
},
options: [],
}
// options[] 里面的每一项会合并 baseOption
cities.forEach((item, i) => {
const color = colors[3][i];
const rowData = {
title: [
{
text: '区域销售大盘',
left: 16,
top: 16,
textStyle: {
color: '#fff',
fontSize: 12,
}
},
{
id: 'statistic',
text: `${item}销售额统计情况`,
top: 8,
right: 4,
textStyle: {
color: '#fff',
fontSize: 12
}
}
],
series: [
// 2 散点图
{
type: 'effectScatter',
coordinateSystem: 'geo',
data: convertData(mapData[i]),
symbolSize: arr => arr[2],
showEffectOn: 'render',
hoverAnimation: true,
// 涟漪配置
// https://echarts.apache.org/zh/option.html#series-effectScatter.rippleEffect
rippleEffect: {
brushType: 'stroke'
},
label: {
normal: {
formatter: '{b}',
position: 'right',
show: false // 全部显示,太拥挤了,高亮显示文字
},
emphasis: {
show: true
}
},
// 散点图颜色和柱图保持一致
itemStyle: {
normal: {
color,
shadowBlur: 8,
shadowColor: color
}
},
zlevel: 1
},
// 1 柱状图
{
zlevel: 1.5,
type: 'bar',
data: barData[i],
itemStyle: {
normal: {color}
},
}
]
}
config.options.push(rowData);
});
return config;
}
export default chinaOption;
convertData
// 散点图的 value前2个值必须是坐标系,最后一个才是真实的数据
export function convertData(data) {
return data.map(item => {
const {name} = item;
const {geoCoordMap} = provinces.find(it => it.name === name) || {};
const value = [...geoCoordMap, randomValue(4, 16)]
return {
name,
value,
}
});
}