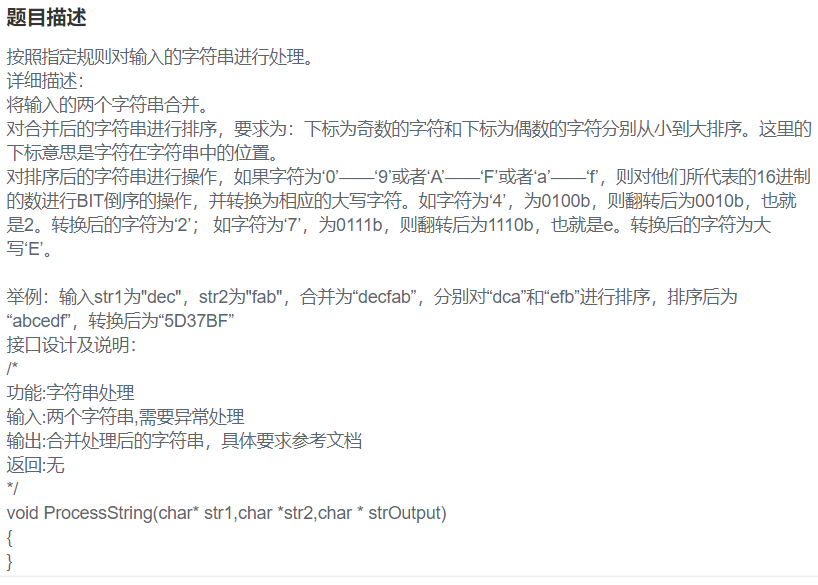
#include <iostream>
#include <vector>
#include <string>
#include <algorithm>
using namespace std;
string code_book = "0123456789ABCDEFabcdef";
string decode_book = "084C2A6E195D3B7F5D3B7F";
string code(string input) {
string output;
output = input;
for (int i = 0; i < input.size(); i++)
{
for (int j = 0; j < code_book.size(); j++)
{
if (output[i]==code_book[j])
{
output[i] = decode_book[j];
//*****************************************
//必须写break,否则会被重复处理
//*****************************************
break;
}
}
}
return output;
}
int main() {
string str_1;
string str_2;
while (cin>>str_1)
{
cin >> str_2;
string str_all = str_1 + str_2;
str_1.clear();
str_2.clear();
for (int i = 0; i < str_all.size(); i++)
{
if (i%2==0)
{
str_1+=str_all[i];
}
else
{
str_2 += str_all[i];
}
}
sort(str_1.begin(), str_1.end());
sort(str_2.begin(), str_2.end());
int str_1_index = 0;
int str_2_index = 0;
for (int i = 0; i < str_all.size(); i++)
{
if (i % 2 == 0)
{
str_all[i] = str_1[str_1_index];
str_1_index++;
}
else
{
str_all[i] = str_2[str_2_index];
str_2_index++;
}
}
str_all = code(str_all);
cout << str_all << endl;
}
}