前言
开发SpringBoot项目过程中,不可避免的需要处理各种异常,SpringMVC架构中各层会出现大量的try {...} catch {...} finally {...}
代码块,不仅有大量的冗余代码,而且还影响代码的可读性。这样就需要定义个全局统一异常处理器,以便业务层再也不必处理异常。
推荐理由
- 代码复制到项目中通过简单的配置即可实现
-
实践
1、封装统一返回结果类
源代码 ```java public class AjaxResult {
//是否成功
private Boolean success;
//状态码
private Integer code;
//提示信息
private String msg;
//数据
private Object data;
public AjaxResult() {}
//自定义返回结果的构造方法
public AjaxResult(Boolean success,Integer code, String msg,Object data) {this.success = success;
this.code = code;
this.msg = msg;
this.data = data;
}
//自定义异常返回的结果
public static AjaxResult defineError(BusinessException de){AjaxResult result = new AjaxResult();
result.setSuccess(false);
result.setCode(de.getErrorCode());
result.setMsg(de.getErrorMsg());
result.setData(null);
return result;
}
//其他异常处理方法返回的结果
public static AjaxResult otherError(ErrorEnum errorEnum){AjaxResult result = new AjaxResult();
result.setMsg(errorEnum.getErrorMsg());
result.setCode(errorEnum.getErrorCode());
result.setSuccess(false);
result.setData(null);
return result;
}
public Boolean getSuccess() {return success;
}
public void setSuccess(Boolean success) {this.success = success;
}
public Integer getCode() {return code;
}
public void setCode(Integer code) {this.code = code;
}
public String getMsg() {return msg;
}
public void setMsg(String msg) {this.msg = msg;
}
public Object getData() {return data;
}
public void setData(Object data) {this.data = data;
}
}
<a name="HUvdB"></a>
### 2、自定义异常封装类
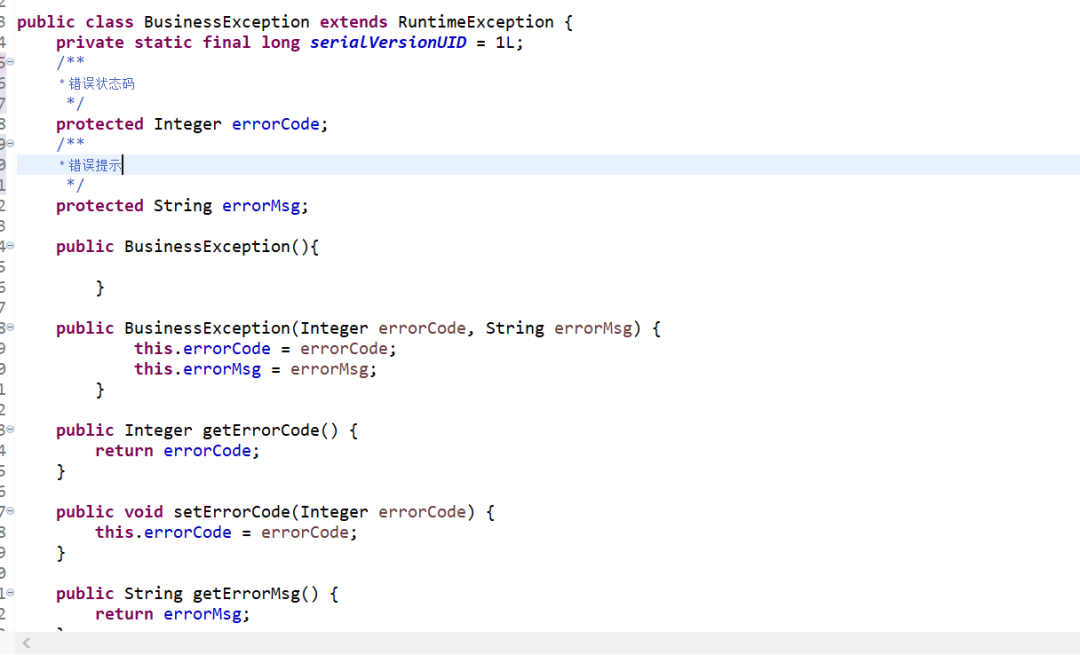<br />源码:
```java
public class BusinessException extends RuntimeException {
private static final long serialVersionUID = 1L;
/**
* 错误状态码
*/
protected Integer errorCode;
/**
* 错误提示
*/
protected String errorMsg;
public BusinessException(){
}
public BusinessException(Integer errorCode, String errorMsg) {
this.errorCode = errorCode;
this.errorMsg = errorMsg;
}
public Integer getErrorCode() {
return errorCode;
}
public void setErrorCode(Integer errorCode) {
this.errorCode = errorCode;
}
public String getErrorMsg() {
return errorMsg;
}
public void setErrorMsg(String errorMsg) {
this.errorMsg = errorMsg;
}
}
3、错误枚举,拒绝硬编码
源码
public enum ErrorEnum {
// 数据操作错误定义
SUCCESS(200, "成功"),
NO_PERMISSION(403,"无权限"),
NO_AUTH(401,"未登录"),
NOT_FOUND(404, "未找到该资源!"),
INTERNAL_SERVER_ERROR(500, "服务器异常请联系管理员"),
;
/** 错误码 */
private Integer errorCode;
/** 错误信息 */
private String errorMsg;
ErrorEnum(Integer errorCode, String errorMsg) {
this.errorCode = errorCode;
this.errorMsg = errorMsg;
}
public Integer getErrorCode() {
return errorCode;
}
public String getErrorMsg() {
return errorMsg;
}
}
4、全局异常处理类
源码
/**
* 全局异常处理器
*
*/
@RestControllerAdvice
public class GlobalExceptionHandler {
private static final Logger log = LoggerFactory.getLogger(GlobalExceptionHandler.class);
/**
* 处理自定义异常
*
*/
@ExceptionHandler(value = BusinessException.class)
public AjaxResult bizExceptionHandler(BusinessException e) {
log.error(e.getMessage(), e);
return AjaxResult.defineError(e);
}
/**
*处理其他异常
*
*/
@ExceptionHandler(value = Exception.class)
public AjaxResult exceptionHandler( Exception e) {
log.error(e.getMessage(), e);
return AjaxResult.otherError(ErrorEnum.INTERNAL_SERVER_ERROR);
}
}