一、ehcache
1. 基本配置
- 引入依赖
<!--ehcache3.9配置-->
<dependency>
<groupId>org.ehcache</groupId>
<artifactId>ehcache</artifactId>
<version>3.9.0</version>
</dependency>
<!--springboot配置-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-cache</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<exclusions><!-- 去掉springboot默认配置 -->
<exclusion>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-logging</artifactId>
</exclusion>
</exclusions>
</dependency>
<!--JSR-107 API的jar 不用也行,xml配置去除jsr的相关配置-->
<dependency>
<groupId>javax.cache</groupId>
<artifactId>cache-api</artifactId>
<version>1.0.0</version>
</dependency>
配置文件
spring:
cache:
#ehcache配置文件路径
ehcache:
config: classpath:config/ehcache.xml
#指定缓存类型,可加可不加
#type: ehcache
创建缓存日志监听器
/**
* 缓存日志监听器
* @author Zhang Xin
* @date 2021-03-15
*/
public class EventLoggerListener implements CacheEventListener<Object, Object> {
private static final Logger logger = LoggerFactory.getLogger(EventLoggerListener.class);
@Override
public void onEvent(CacheEvent<?, ?> event) {
logger.info("缓存事件: 类型[{}], 键值[{}], 原值[{}], 新值[{}]",
event.getType(),
event.getKey(),
event.getOldValue(),
event.getNewValue());
}
}
在 resource 的 config 目录下创建 encache.xml 文件
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="http://www.ehcache.org/v3"
xmlns:jsr107="http://www.ehcache.org/v3/jsr107"
xsi:schemaLocation="
http://www.ehcache.org/v3 http://www.ehcache.org/schema/ehcache-core-3.0.xsd
http://www.ehcache.org/v3/jsr107 http://www.ehcache.org/schema/ehcache-107-ext-3.0.xsd">
<!-- <service>-->
<!-- <jsr107:defaults enable-statistics="true"/>-->
<!-- </service>-->
<service>
<jsr107:defaults>
<!--用别名“person”定义一个缓存,该别名从缓存模板“cache-template-default”继承-->
<jsr107:cache name="person" template="cache-template-default" />
</jsr107:defaults>
</service>
<!-- 不用上面的jsr107配置用下方的定义方式也行-->
<!-- 定义缓存空间CommonCache,之后的配置缓存空间名字person改成CommonCache即可 -->
<cache alias="CommonCache" uses-template="cache-template-default">
<expiry>
<ttl unit="seconds">300</ttl>
</expiry>
</cache>
<cache-template name="cache-template-default">
<!--本部分允许您添加缓存事件侦听器。我为5个事件添加了一个侦听器。
发生时,每个事件将由EventLogger类记录。-->
<listeners>
<listener>
<class>com.example.ehcache.ehcachelearn.listener.EventLoggerListener</class>
<event-firing-mode>ASYNCHRONOUS</event-firing-mode>
<event-ordering-mode>UNORDERED</event-ordering-mode>
<!--使用此侦听器将条目添加到缓存时,定义CREATED事件。-->
<events-to-fire-on>CREATED</events-to-fire-on>
<!--使用此侦听器在缓存中更新条目时定义一个UPDATED事件-->
<events-to-fire-on>UPDATED</events-to-fire-on>
<!-- 当条目从缓存中使用此侦听器过期时,定义一个EXPIRED事件。-->
<events-to-fire-on>EXPIRED</events-to-fire-on>
<!--当使用此侦听器从缓存中删除条目时,定义一个REMOVED事件。-->
<events-to-fire-on>REMOVED</events-to-fire-on>
<!--当使用此侦听器从缓存中逐出条目时,定义EVICTED事件。-->
<events-to-fire-on>EVICTED</events-to-fire-on>
</listener>
</listeners>
<resources>
<!--堆配置为允许2000个条目-->
<heap unit="entries">2000</heap>
<!--堆外存储配置有100 MB的空间。请记住,度量单位区分大小写。-->
<offheap unit="MB">100</offheap>
</resources>
</cache-template>
</config>
- 启动类加上注解@EnableCaching
@EnableCaching
@SpringBootApplication
public class SpringbootCacheApplication {
public static void main(String[] args) {
SpringApplication.run(SpringbootCacheApplication.class, args);
}
}
* XML配置详情
FQCN(Full Qualified Class Name)完全限定类名;
<config
xmlns:ehcache="http://www.ehcache.org/v3"
xmlns:jcache="http://www.ehcache.org/v3/jsr107">
<!--
可选
由CacheManager管理和生命周期化的服务
-->
<service>
<!--
另一个命名空间中的一个元素,这里以我们的JSR-107扩展为例
-->
<jcache:defaults>
<jcache:cache name="invoices" template="myDefaultTemplate"/>
</jcache:defaults>
</service>
<!--
可选
<cache>元素定义由强制性“ alias”属性标识的缓存,由CacheManager管理
-->
<cache alias="productCache">
<!--
可选,默认为java.lang.Object
-->
<key-type copier="org.ehcache.impl.copy.SerializingCopier">java.lang.Long</key-type>
<!--
可选,默认为java.lang.Object
-->
<value-type copier="org.ehcache.impl.copy.SerializingCopier">com.pany.domain.Product</value-type>
<!--
可选,默认为无有效期
可以使缓存条目在给定时间后过期
-->
<expiry>
<!--
<tti>空闲时间,即条目保持原状的最长时间
可以使缓存条目在给定时间后过期
其他选项是:
* <ttl>,生存时间;
* <class>,用于自定义Expiry实现
* <无>,无有效期
-->
<tti unit="minutes">2</tti>
</expiry>
<!--
可选,默认为不建议
驱逐顾问,可让您控制哪些条目仅应在万不得已时被逐出
org.ehcache.config.EvictionAdvisor实现的FQCN
-->
<eviction-advisor>com.pany.ehcache.MyEvictionAdvisor</eviction-advisor>
<!--
可选,
让我们将缓存配置为“直通式”,
也就是说,使用CacheLoaderWriter的Cache会在未命中加载,并在变异操作中进行写入。
-->
<loader-writer>
<!--
FQCN实现org.ehcache.spi.loaderwriter.CacheLoaderWriter
-->
<class>com.pany.ehcache.integration.ProductCacheLoaderWriter</class>
<!-- 另一个名称空间中的任何其他元素 -->
</loader-writer>
<!--
逐出之前,要保留在缓存中的最大条目数
-->
<heap unit="entries">200</heap>
<!--
可选
另一个名称空间中的任何其他元素
-->
</cache>
<!--
可选
<cache-template>定义了一个命名模板,该模板可以用作同一文件中的<cache>定义
它们具有与上述<cache>元素相同的属性
-->
<cache-template name="myDefaultTemplate">
<expiry>
<none/>
</expiry>
<!--
可选
另一个名称空间中的任何其他元素
-->
</cache-template>
<!--
通过在uses-template属性中引用缓存模板的名称来使用上述模板的<cache>:
-->
<cache alias="customerCache" uses-template="myDefaultTemplate">
<!--
添加键和值类型配置
-->
<key-type>java.lang.Long</key-type>
<value-type>com.pany.domain.Customer</value-type>
<!--
将模板设置的容量限制覆盖为新值
-->
<heap unit="entries">200</heap>
</cache>
</config>
2. 使用工具类
- 引入依赖 配置 JSONUtils
JSONUtils: ```java import com.alibaba.fastjson.JSON; import java.util.List;<dependency>
<groupId>com.alibaba</groupId>
<artifactId>fastjson</artifactId>
<version>1.2.75</version>
</dependency>
/**
- JSON工具类
- @author Zhang Xin
@date 2021-3-23 */ public class JSONUtils { private JSONUtils() {}
/**
- 对象转JSON格式
- @param o
@return */ public static String toJSONString(Object o){ return JSON.toJSONString(o); }
/**
- JSON格式字符串转泛型对象
- @param text
- @param clazz
- @param
@return */ public static
T parseObject(String text, Class clazz) { return JSON.parseObject(text, clazz); } public static
List parseList(String text, Class clazz) { return JSON.parseArray(text, clazz); }
}
2. 配置EhcacheUtils
详见[https://blog.csdn.net/q1248807225/article/details/105536606](https://blog.csdn.net/q1248807225/article/details/105536606)
```java
import org.ehcache.Cache;
import org.ehcache.CacheManager;
import org.ehcache.config.CacheConfiguration;
import org.ehcache.config.builders.CacheConfigurationBuilder;
import org.ehcache.config.builders.CacheManagerBuilder;
import org.ehcache.config.builders.ExpiryPolicyBuilder;
import org.ehcache.config.builders.ResourcePoolsBuilder;
import org.ehcache.config.units.EntryUnit;
import org.ehcache.config.units.MemoryUnit;
import java.time.Duration;
import java.util.List;
/**
* Ehcache缓存工具类
* @author ZX
* @date 2021-01-15
*/
public class EhcacheUtils {
/**
* 初始化Ehcache配置
*/
private static CacheConfiguration<String, String> usesConfiguredInCacheConfig;
/**
* 初始化管理器
*/
private static CacheManager cacheManager;
private static final String COMMON_CACHE = "person";
static {
init();
}
private static void init(){
if(usesConfiguredInCacheConfig == null) {
usesConfiguredInCacheConfig = CacheConfigurationBuilder
.newCacheConfigurationBuilder(String.class, String.class,
ResourcePoolsBuilder.newResourcePoolsBuilder()
// 基于堆内存
.heap(2000L, EntryUnit.ENTRIES)
// 基于内存
.offheap(20L, MemoryUnit.MB))
.withSizeOfMaxObjectGraph(2000L)
.withSizeOfMaxObjectSize(100L, MemoryUnit.KB)
//失效时间5秒,time-to-idle 最后一次使用后计算,所存储的时间5秒没对条目进行操作则失效
.withExpiry(ExpiryPolicyBuilder.timeToIdleExpiration(Duration.ofSeconds(5)))
.build();
}
if(cacheManager == null) {
cacheManager = CacheManagerBuilder.newCacheManagerBuilder()
.withDefaultSizeOfMaxObjectSize(50L, MemoryUnit.KB)
.withDefaultSizeOfMaxObjectGraph(2000)
.withCache(COMMON_CACHE, usesConfiguredInCacheConfig)
.build(true);
}
}
public static Cache<String, String> getCache(String cacheName){
return cacheManager
.getCache(cacheName, String.class, String.class);
}
/**
* 设置缓存
* @param key
* @param Value
*/
public static void set(String key, Object Value) {
getCache(COMMON_CACHE).put(key, JSONUtils.toJSONString(Value));
}
/**
* 获取缓存
* @param key
* @param clazz
* @param <T>
* @return
*/
public static <T> T get(String key, Class<T> clazz) {
return JSONUtils.parseObject(getCache(COMMON_CACHE).get(key), clazz);
}
/**
* 获取缓存
* @param key
* @return
*/
public static String get(String key) {
return getCache(COMMON_CACHE).get(key);
}
/**
* 获取缓存返回list列表
* @param key
* @param clazz
* @param <T>
* @return
*/
public static <T> List<T> list(String key, Class<T> clazz) {
return JSONUtils.parseList(getCache(COMMON_CACHE).get(key), clazz);
}
/**
* 移除缓存key
* @param key
*/
public static void remove(String key) {
getCache(COMMON_CACHE).remove(key);
}
/**
* 缓存清理
*/
public static void clear() {
getCache(COMMON_CACHE).clear();
}
/**
* 关闭管理器
*/
public static void close() {
cacheManager.close();
}
public static void main(String[] args) {
init();
set("haha", "哈哈哈");
String haha = get("haha");
System.out.println(haha);
}
}
如:withExpiry(ExpiryPolicyBuilder.timeToIdleExpiration(Duration.ofHours(24))
- no expiry 永不过期
- time-to-live 从缓存开始计算,所存储的时间
time-to-idle 最后一次使用后计算,所存储的时间
用 Controller 测试 ```java @RestController @RequestMapping(“/ehcache”) public class PersonController {
@GetMapping(“/a”) public void ehcacheSet(){
EhcacheUtils.set("haha", "哈哈哈");
System.out.println("存入缓存");
}
@GetMapping(“/b”) public void ehcacheGet(){
String haha = EhcacheUtils.get("haha");
System.out.println(haha);
}
}
结果:<br />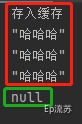<br />缓存工具类中设置了失效时间5秒,5秒没对条目进行操作则失效
<a name="N7s36"></a>
## 3. 使用注解
<a name="5UjaD"></a>
### 2.1 简单使用
1. 编写缓存配置类
```java
import java.util.concurrent.TimeUnit;
import javax.cache.CacheManager;
import javax.cache.configuration.MutableConfiguration;
import javax.cache.expiry.Duration;
import javax.cache.expiry.TouchedExpiryPolicy;
import org.springframework.boot.autoconfigure.cache.JCacheManagerCustomizer;
import org.springframework.stereotype.Component;
@Component
public class Ehcache3Config implements JCacheManagerCustomizer {
private static final String NAME_CACHE = "person";
@Override
public void customize(CacheManager cacheManager) {
// 创建别名为“person”的缓存。
cacheManager.createCache(NAME_CACHE,
new MutableConfiguration<>()
// 该行设置了过期策略。在这种情况下,我们将其设置为 2 秒。
// 因此,如果在过去的 2 秒钟内未触摸(创建,更新或访问)某个条目,则该条目将被驱逐。
.setExpiryPolicyFactory(TouchedExpiryPolicy.factoryOf(new Duration(TimeUnit.SECONDS, 2)))
.setStoreByValue(true).setStatisticsEnabled(true));
}
}
- 编写 Service 类,person 代表要读取的数据,加上注解 @Cacheable ```java import org.springframework.cache.annotation.Cacheable; import org.springframework.stereotype.Service;
@Service public class PersonService { @Cacheable(cacheNames = “person”,key = “#id”) public Person getPerson(Long id){ System.out.println(“调用Service”); Person person = new Person(id,”ramostear”,”ramostear@163.com”); return person; } }
3. Controller 调用
```java
@RestController
@RequestMapping("/persons")
public class PersonController {
@Autowired
private PersonService personService;
@GetMapping("/{id}")
public ResponseEntity<Person> person(@PathVariable(value = "id") Long id){
Person person = personService.getPerson(id);
System.out.println("调用接口");
return new ResponseEntity<>(person, HttpStatus.OK);
}
}
结果:
在 Config 配置文件中设置了过期策略为2秒。 因此,如果在过去的 2 秒钟内未触摸(创建,更新或访问)某个条目,则该条目将被驱逐,将重新调用Service中的方法
2.2 注解配置详情
@Cacheable
@Cacheable 的作用 主要针对方法配置,能够根据方法的请求参数对其结果进行缓存
@Cacheable 作用和配置方法
参数 | 解释 | example |
---|---|---|
value | 缓存的名称,在 spring 配置文件中定义,必须指定至少一个 | 例如: @Cacheable(value=”mycache”) @Cacheable(value={”cache1”,”cache2”} |
|
| key | 缓存的 key,可以为空,如果指定要按照 SpEL 表达式编写,如果不指定,则缺省按照方法的所有入参进行组合 | @Cacheable(value=”testcache”,key=”#userName”) |
| condition | (执行方法之前)
缓存的条件,可以为空,使用 SpEL 编写,返回 true 或者 false,只有为 true 才进行缓存 | @Cacheable(value=”testcache”,condition=”#userName.length()>2”) |
@Cacheable(value=”accountCache”),这个注释的意思是,当调用这个方法的时候,会从一个名叫 accountCache 的缓存中查询,如果没有,则执行实际的方法(即查询数据库),并将执行的结果存入缓存中,否则返回缓存中的对象。
@CachePut
@CachePut 的作用 主要针对方法配置,能够根据方法的请求参数对其结果进行缓存,和 @Cacheable 不同的是,它每次都会触发真实方法的调用
@CachePut 作用和配置方法
参数 | 解释 | example |
---|---|---|
value | 缓存的名称,在 spring 配置文件中定义,必须指定至少一个 | @CachePut(value=”my cache”) |
key | 缓存的 key,可以为空,如果指定要按照 SpEL 表达式编写,如果不指定,则缺省按照方法的所有参数进行组合 | @CachePut(value=”testcache”,key=”#userName”) |
condition | (执行完方法后) 缓存的条件,可以为空,使用 SpEL 编写,返回 true 或者 false,只有为 true 才进行缓存 |
@CachePut(value=”testcache”,condition=”#userName.length()>2”) |
@CachePut 注释,这个注释可以确保方法被执行,同时方法的返回值也被记录到缓存中,实现缓存与数据库的同步更新。
@CachePut(value="accountCache",key="#account.getName()")// 更新accountCache 缓存
public Account updateAccount(Account account) {
return updateDB(account);
}
@CacheEvict
@CachEvict 的作用 主要针对方法配置,能够根据一定的条件对缓存进行清空
@CacheEvict 作用和配置方法
参数 | 解释 | example |
---|---|---|
value | 缓存的名称,在 spring 配置文件中定义,必须指定至少一个 | @CacheEvict(value=”my cache”) |
key | 缓存的 key,可以为空,如果指定要按照 SpEL 表达式编写,如果不指定,则缺省按照方法的所有参数进行组合 | @CacheEvict(value=”testcache”,key=”#userName”) |
condition | (默认方法执行之后,看配置beforeInvocation) 缓存的条件,可以为空,使用 SpEL 编写,返回 true 或者 false,只有为 true 才进行缓存 |
@CacheEvict(value=”testcache”,condition=”#userName.length()>2”) |
allEntries | 是否清空所有缓存内容,缺省为 false,如果指定为 true,则方法调用后将立即清空所有缓存 | @CachEvict(value=”testcache”,allEntries=true) |
beforeInvocation | 是否在方法执行前就清空,缺省为 false,如果指定为 true,则在方法还没有执行的时候就清空缓存,缺省情况下,如果方法执行抛出异常,则不会清空缓存 | @CachEvict(value=”testcache”,beforeInvocation=true) |
实例
@CacheEvict(value="accountCache",key="#account.getName()")// 清空accountCache 缓存
public void updateAccount(Account account) {
updateDB(account);
}
@CacheEvict(value="accountCache",allEntries=true)// 方法调用后将立即清空 accountCache 缓存
public void reload() {
reloadAll()
}
@Cacheable(value="accountCache",condition="#userName.length() <=4")// 缓存名叫 accountCache
public Account getAccountByName(String userName) {
// 方法内部实现不考虑缓存逻辑,直接实现业务
return getFromDB(userName);
}
@CacheConfig
所有的@Cacheable()里面都有一个value=“xxx”的属性,这显然如果方法多了,写起来也是挺累的,如果可以一次性声明完 那就省事了, 所以,有了@CacheConfig这个配置,@CacheConfig is a class-level annotation that allows to share the cache names,如果你在你的方法写别的名字,那么依然以方法的名字为准。
例:
@CacheConfig("books")
public class BookRepositoryImpl implements BookRepository {
@Cacheable
public Book findBook(ISBN isbn) {...}
}
条件缓存(使用示例)
一些常用的条件缓存
EQ 就是 EQUAL 等于
NE 就是 NOT EQUAL 不等于
GT 就是 GREATER THAN 大于
GE 就是 GREATER THAN OR EQUAL 大于等于
LT 就是 LESS THAN 小于
LE 就是 LESS THAN OR EQUAL 小于等于
//@Cacheable将在执行方法之前( #result还拿不到返回值)判断condition,如果返回true,则查缓存;
@Cacheable(value = "user", key = "#id", condition = "#id lt 10")
public User conditionFindById(final Long id)
//@CachePut将在执行完方法后(#result就能拿到返回值了)判断condition,如果返回true,则放入缓存;
@CachePut(value = "user", key = "#id", condition = "#result.username ne 'zhang'")
public User conditionSave(final User user)
//@CachePut将在执行完方法后(#result就能拿到返回值了)判断unless,
//如果返回false,则放入缓存;(即跟condition相反)
@CachePut(value = "user", key = "#user.id", unless = "#result.username eq 'zhang'")
public User conditionSave2(final User user)
//@CacheEvict, beforeInvocation=false表示在方法执行之后调用(#result能拿到返回值了);
//且判断condition,如果返回true,则移除缓存;
@CacheEvict(value = "user", key = "#user.id", beforeInvocation = false, condition = "#result.username ne 'zhang'")
public User conditionDelete(final User user)
@Caching
(key)–>(value)
有时候我们可能组合多个Cache注解使用;比如用户新增成功后,我们要添加id–>user;username—>user;email—>user的缓存;此时就需要@Caching组合多个注解标签了。
@Caching(put = {
@CachePut(value = "user", key = "#user.id"),
@CachePut(value = "user", key = "#user.username"),
@CachePut(value = "user", key = "#user.email")
})
public User save(User user) {
自定义缓存注解
比如之前的那个@Caching组合,会让方法上的注解显得整个代码比较乱,此时可以使用自定义注解把这些注解组合到一个注解中,如:
@Caching(put = {
@CachePut(value = "user", key = "#user.id"),
@CachePut(value = "user", key = "#user.username"),
@CachePut(value = "user", key = "#user.email")
})
@Target({ElementType.METHOD, ElementType.TYPE})
@Retention(RetentionPolicy.RUNTIME)
@Inherited
public @interface UserSaveCache {
}
这样我们在方法上使用如下代码即可,整个代码显得比较干净。
@UserSaveCache
public User save(User user)
扩展优化
(key)–>(value)
比如findByUsername时,不应该只放username–>user,应该连同id—>user和email—>user一起放入;这样下次如果按照id查找直接从缓存中就命中了
@Caching(
cacheable = {
@Cacheable(value = "user", key = "#username")
},
put = {
@CachePut(value = "user", key = "#result.id", condition = "#result != null"),
@CachePut(value = "user", key = "#result.email", condition = "#result != null")
}
)
public User findByUsername(final String username) {
System.out.println("cache miss, invoke find by username, username:" + username);
for (User user : users) {
if (user.getUsername().equals(username)) {
return user;
}
}
return null;
}
其实对于:id—>user;username—->user;email—>user;更好的方式可能是:id—>user;username—>id;email—>id;保证user只存一份;如:
@CachePut(value="cacheName", key="#user.username", cacheValue="#user.username")
public void save(User user)
@Cacheable(value="cacheName", key="#user.username", cacheValue="#caches[0].get(#caches[0].get(#username).get())")
public User findByUsername(String username)
SpEL上下文数据
Spring Cache提供了一些供我们使用的SpEL上下文数据,下表直接摘自Spring官方文档:
名称 | 位置 | 描述 | 示例 |
---|---|---|---|
methodName | root对象 | 当前被调用的方法名 | root.methodName |
method | root对象 | 当前被调用的方法 | root.method.name |
target | root对象 | 当前被调用的目标对象 | root.target |
targetClass | root对象 | 当前被调用的目标对象类 | root.targetClass |
args | root对象 | 当前被调用的方法的参数列表 | root.args[0] |
caches | root对象 | 当前方法调用使用的缓存列表(如@Cacheable(value={“cache1”, “cache2”})),则有两个cache | root.caches[0].name |
argument name | 执行上下文 | 当前被调用的方法的参数,如findById(Long id),我们可以通过#id拿到参数 | user.id |
result | 执行上下文 | 方法执行后的返回值(仅当方法执行之后的判断有效,如‘unless’,’cache evict’的beforeInvocation=false) | result |
使用例子:
@CacheEvict(value = "user", key = "#user.id", condition = "#root.target.canCache() and #root.caches[0].get(#user.id).get().username ne #user.username", beforeInvocation = true)
public void conditionUpdate(User user)
二、jetcache
https://github.com/alibaba/jetcache/wiki/Home_CN
1.基本概念
(1) 简介:
JetCache是一个基于Java的缓存系统封装,提供统一的API和注解来简化缓存的使用。 JetCache 提供了比SpringCache 更加强大的注解,可以原生的支持TTL、两级缓存、分布式自动刷新,还提供了Cache接口用于手工缓存操作。当前有四个实现,RedisCache、TairCache(此部分未在github开源)、CaffeineCache(in memory) 和一个简易的 LinkedHashMapCache(in memory),要添加新的实现也是非常简单的。
(2) 全部特性:
- 通过统一的API访问Cache系统
- 通过注解实现声明式的方法缓存,支持TTL和两级缓存
- 通过注解创建并配置Cache实例
- 针对所有Cache实例和方法缓存的自动统计
- Key的生成策略和Value的序列化策略是可以配置的
- 分布式缓存自动刷新,分布式锁 (2.2+)
- 异步Cache API (2.2+,使用Redis的lettuce客户端时)
- Spring Boot支持
(3) 要求:
JetCache需要JDK1.8、Spring Framework4.0.8以上版本。Spring Boot为可选,需要1.1.9以上版本。如果不使用注解(仅使用jetcache-core),Spring Framework也是可选的,此时使用方式与Guava/Caffeinecache类似。
** 依赖哪个jar?
- jetcache-anno-api:定义jetcache的注解和常量,不传递依赖。如果你想把Cached注解加到接口上,又不希望你的接口jar传递太多依赖,可以让接口jar依赖 jetcache-anno-api。
- jetcache-core:核心api,完全通过编程来配置操作Cache,不依赖Spring。两个内存中的缓存实现LinkedHashMapCache 和 CaffeineCache 也由它提供。
- jetcache-anno:基于Spring提供@Cached和@CreateCache注解支持。
- jetcache-redis:使用jedis提供Redis支持。
- jetcache-redis-lettuce(需要JetCache2.3以上版本):使用lettuce提供Redis支持,实现了JetCache异步访问缓存的的接口。
- jetcache-starter-redis:Spring Boot方式的Starter,基于Jedis。
- jetcache-starter-redis-lettuce(需要JetCache2.3以上版本):Spring Boot方式的Starter,基于Lettuce。
2.使用jetcache
引入依赖
<dependency>
<groupId>com.alicp.jetcache</groupId>
<artifactId>jetcache-starter-redis</artifactId>
<version>2.4.4</version>
</dependency>
配置yml文件
jetcache:
# 每隔多久统计信息的时长配置
statIntervalMinutes: 15
# 是否配置前缀
areaInCacheName: false
local:
default:
# 本地缓存类型
type: linkedhashmap
# key的序列化转化的协议
keyConvertor: fastjson
# 本地缓存最大个数
limit: 10000
# 缓存的时间全局默认值
defaultExpireInMillis: 10000
remote:
default:
# 缓存数据库类型
type: redis
keyConvertor: fastjson
valueEncoder: java
valueDecoder: java
poolConfig:
minIdle: 5
maxIdle: 20
maxTotal: 50
host: 127.0.0.1
port: 6379
- 用@EnableMethodCache ,@EnableCreateCacheAnnotation这两个注解分别激活@Cached 和@CreateCache 注解
@SpringBootApplication
// 激活Cached 注解
@EnableMethodCache(basePackages = "com.zx.mypackage")
// 激活CreateCache 注解
@EnableCreateCacheAnnotation
public class ServerApplication {
public static void main(String[] args) {
SpringApplication.run(ServerApplication.class, args);
}
}
- 代码中使用cache注解
@Service
public class UserServiceImpl implements UserService {
@Autowired
private UserMapper userMapper;
//使用ehcache配置的缓存名users_test
private final String USER_CACHE_NAME = "users_test";
@Override
public List<User> listUser() {
return userMapper.selectUserList();
}
@Override
// @Cacheable(value = USER_CACHE_NAME, key = "#id")
@Cacheable(value = USER_CACHE_NAME, key = "'user' + #id")
public User selectUserById(Integer id) {
return userMapper.selectUserById(id);
}
@Override
// @CacheEvict(value = USER_CACHE_NAME, key = "#id")
@CacheEvict(value = USER_CACHE_NAME, key = "'user_' + #id")
public void delete(Integer id) {
userMapper.delete(id);
}
@Override
// @CacheEvict(value = USER_CACHE_NAME, key = "#user.userId")
@CacheEvict(value = USER_CACHE_NAME, key = "'user' + #user.userId")
// @CachePut(value = USER_CACHE_NAME, key = "'user' + #user.userId")
// 测试发现只将结果清除,key未清除,导致查询继续使用缓存但结果为空????
public void update(User user) {
userMapper.update(user);
}
}