为什么使用数组
int a, b, c, d;
int a1, a2, a3, a4, a5, a6, a7....;
int a[100]; //定义100个int类型
a[0], a[1], a[2], a[3], a[4]...
[0][1][2][3][4][5][6][7]...
//连续地址空间存储的
//静态数组,大小是静态固定的,上来就要告诉计算机,我需要100个连续的int空间
//然后,我想用几个就用几个。但是,千万不能要超。
//a[0] ... a[99],这些是100个,但不能用a[100],这是第101个
//因为,a[101]这个位置,计算机可能存储了其他的东西,有可能是危险的东西(越界访问)
一维数组
#include <iostream>
using namespace std;
int a[10]; //全局变量,初始值默认是0
int n;
int main()
{
int b[10]; //局部变量,初始值是计算机随机给的
cin >> n;
for (int i = 0; i < n; i++) cin >> a[i];
for (int i = 0; i < n; i++) cout << a[i] << ' ';
cout << '\n';
return 0;
}
#include <iostream>
#include <cstdio>
using namespace std;
const int N = 1e5 + 10;
int a[N], b[N];
int n;
int main()
{
cin >> n;
for (int i = 0; i < n; i++) cin >> a[i];
for (int i = 0; i < n; i++) cout << a[i] << ' ';
puts("");
return 0;
}
int a[5] = {1, 2, 3, 4, 5};
a[-1]; //这是不对的,下标不能是负数
memset(a, 0, sizeof a); //把a[i]全部置成0
二维数组
#include <iostream>
using namespace std;
const int N = 110;
int a[N][N];
int n, m; //n行m列
int main()
{
cin >> n >> m;
for (int i = 0; i < n; i++)
for (int j = 0; j < n; j++)
cin >> a[i][j];
//逐行逐列扫描
for (int i = 0; i < n; i++){
for (int j = 0; j < n; j++) cout << a[i][j] << ' ';
puts("");
}
return 0;
}
字符类型和字符数组
// 字符类型
char c;
int a[N];
double a[N];
bool a[N];
// 字符数组
char s[N];
char s[N][N];
/*
.#...#
...#..
#.....
*/
// 界定符
'a' 字符 ' '
"a" 字符串 "abc" "" " "
a[0] = '\n';
cout << a[0];
#include <bits/stdc++.h>
using namespace std;
int main()
{
cout << sizeof ('a') << '\n';
cout << sizeof ("a") << '\n';
return 0;
}
/*
1
2
*/
字符占一个字节,“a”会增加一个字节,用来存放字符串结束符'\0',所以占2字节
// 字符串读入
#include <cstdio>
using namespace std;
char s[110];
int main()
{
scanf("%s", s);
printf("%s\n", s);
return 0;
}
// string类型
#include <iostream>
using namespace std;
int main()
{
string s;
cin >> s;
cout << s << '\n';
return 0;
}
// 读入一整行
// ab cd ed
#include <iostream>
using namespace std;
int main()
{
string s;
getline(cin, s);
cout << s << '\n';
return 0;
}
// 字符串处理函数
#include <iostream>
#include <cstring>
using namespace std;
int main()
{
string s;
s = "abcdef";
cout << s << '\n';
cout << s.size() << '\n'; //输出字符串大小
cout << s.substr(2) << '\n'; //截取子串,是位置2开始往后所有
cout << s.substr(2, 3) << '\n'; //从位置2开始,截取三位
return 0;
}
/*
二维字符数组
.....#
####.#
###..#
##.#..
*/
#include <iostream>
#include <cstdio>
using namespace std;
const int N = 110;
char s[N][N];
int n, m;
int main()
{
cin >> n >> m;
for (int i = 0; i < n; i++) scanf("%s", s[i]);
for (int i = 0; i < n; i++) printf("%s\n", s[i]);
return 0;
}
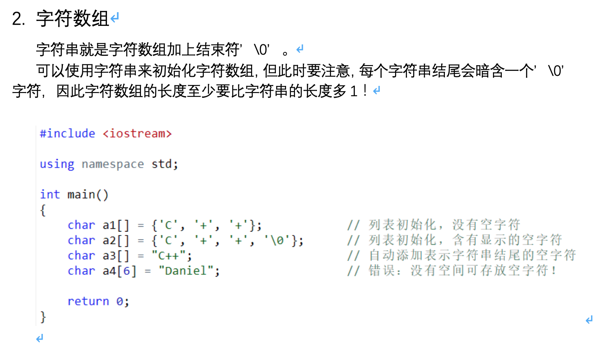
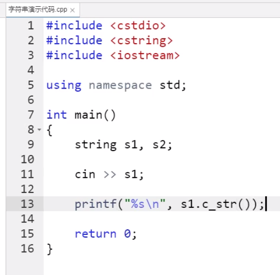

空间复杂度
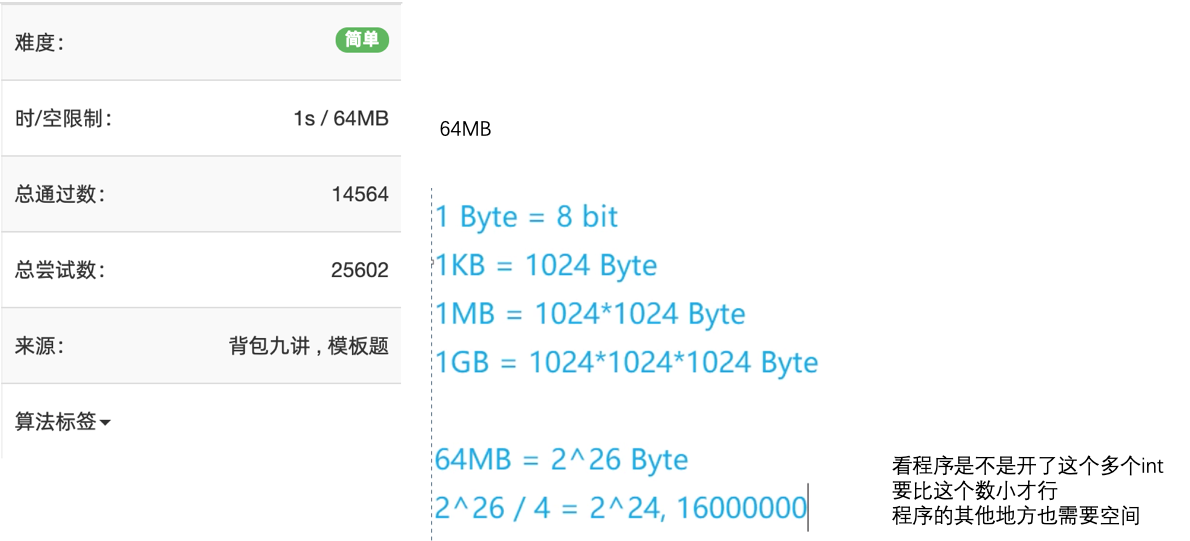
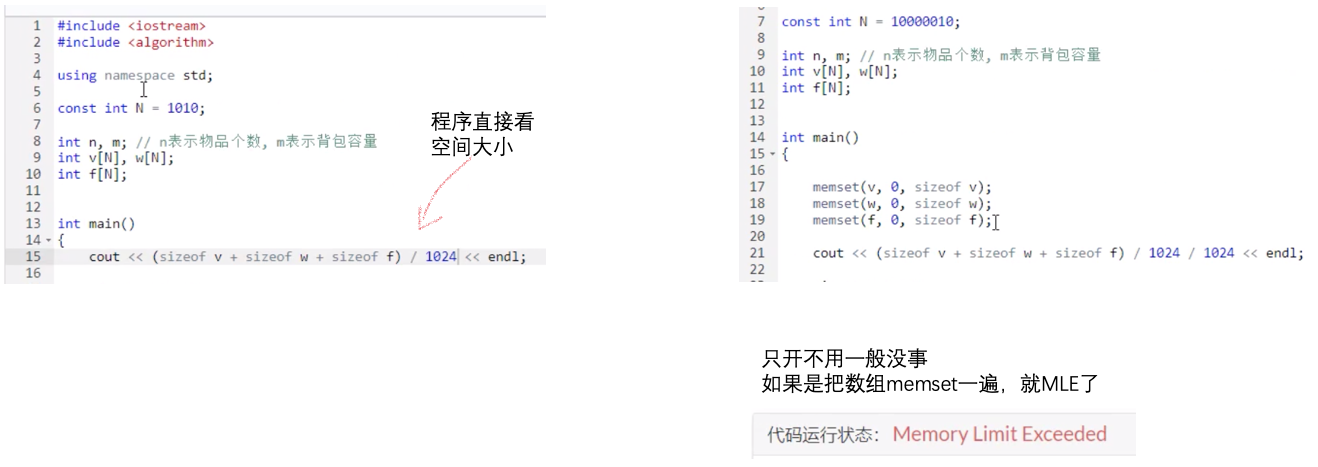
一本通习题
一维数组
与指定数字相同的数的个数
//
陶陶摘苹果
//
计算书费
//
数组逆序重存放
//
年龄与疾病
//
校门外的树
//原题
向量点积计算
//
开关灯
//
查找特定的值
//
不高兴的津津
//原题
最大值和最小值的差
//打擂台
不与最大数相同的数字之和
//
白细胞计数
//
直方图
//
最长平台
//
整数去重
//
铺地毯
//
二维数组
矩阵交换行
//
同行列对角线的格
//
计算矩阵边缘元素之和
//
计算鞍点
//
图像相似度
//
矩阵加法
//
矩阵乘法
//
矩阵转置
//
图像旋转
//
图像模糊处理
//
字符数组
统计数字字符个数
//
找第一个只出现一次的字符
//
基因相关性
//
石头剪子布
//
输出亲朋字符串
//
合法C标识符查
//
配对碱基链
//
密码翻译
//
加密的病历单
//
将字符串中的小写字母转换成大写字母
//
整理药名
//
验证子串
//string s;
//s.find()
删除单词后缀
//string s;
//s.erase(len - 2)
//使用char s[40];
//匹配后缀成功后,利用s[len-2] = '\0'进行截断
单词的长度
//getline(cin, s);
//然后遍历,碰到空格算一个单词
//要注意输出的格式
//使用while (cin >> s),更好编写
//不过要注意一下格式控制的小技巧
//本地调试要使用freopen
//输出格式如下
3,3,4,2,10,3,4,7,5
最长最短单词
//
单词翻转
//getline(cin, s);
//比较麻烦,构造一个一个单词出来,然后翻转
//使用while (cin >> s)
//会两个点,格式错误,调不出来
字符串p型编码
//
判断字符串是否为回文
//
最高分数的学生姓名
//
连续出现的字符
//
最长单词2
//