效果图:
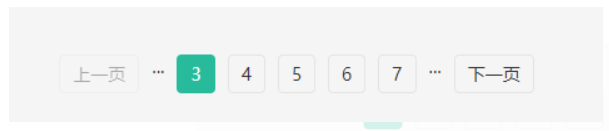
初始结构:
<template>
<div class="xtx-pagination">
<a href="javascript:;" class="disabled">上一页</a>
<span>...</span>
<a href="javascript:;" class="active">3</a>
<a href="javascript:;">4</a>
<a href="javascript:;">5</a>
<a href="javascript:;">6</a>
<a href="javascript:;">7</a>
<span>...</span>
<a href="javascript:;">下一页</a>
</div>
</template>
<script>
export default {
name: 'XtxPagination'
}
</script>
<style scoped lang="less">
.xtx-pagination {
display: flex;
justify-content: center;
padding: 30px;
> a {
display: inline-block;
padding: 5px 10px;
border: 1px solid #e4e4e4;
border-radius: 4px;
margin-right: 10px;
&:hover {
color: @xtxColor;
}
&.active {
background: @xtxColor;
color: #fff;
border-color: @xtxColor;
}
&.disabled {
cursor: not-allowed;
opacity: 0.4;
&:hover {
color: #333
}
}
}
> span {
margin-right: 10px;
}
}
</style>
落地代码:
<template>
<div class="xtx-pagination">
<a href="javascript:;" v-if="myCurrentPage===1" class="disabled">上一页</a>
<a href="javascript:;" v-else @click="go(-1)">上一页</a>
<!-- 如果起点按钮大于2就显示 -->
<span v-if="pageInfo.start>2">...</span>
<a href="javascript:;" @click="changePage(item)" :class="{active: myCurrentPage === item}" v-for="(item,idx) in pageInfo.pager" :key="idx"> {{item}} </a>
<!-- 如果当前终点按钮小于总页数就显示 当终点按钮是20总按钮也是20 就不显示 -->
<span v-if="pageInfo.end < pageInfo.pageCount">...</span>
<!-- 如果当前按钮等于最后一页就禁止点击 disabled -->
<a href="javascript:;" v-if="myCurrentPage==pageInfo.end" class="disabled">下一页</a>
<a href="javascript:;" v-else @click="go(1)">下一页</a>
</div>
</template>
<script>
import { computed, ref, watch } from 'vue'
export default {
name: 'XtxPagination',
props: {
total: { type: Number, default: 100 },
pageSize: { type: Number, default: 10 },
currentPage: { type: Number, default: 1 },
btnCount: { type: Number, default: 5 }
},
setup (props, { emit }) {
const myTotal = ref(100) // 总条数
const myPageSize = ref(5) // 每页共几条
const myCurrentPage = ref(3) // 用户实时点击,修改
const myBtnCount = ref(5) // 分页按钮的个数5个
// 让当前的页码处于正中间
// const pager = ref([1, 2, 3, 4, 5])
// 根据上边信息,实时计算 pager,起始页码,结束页码
const pageInfo = computed(() => {
// 总页数 = 总条数/每页几条
const pageCount = Math.ceil(myTotal.value / myPageSize.value)
// 起点 = 当前页数-总页数/2 举例 3 - Math.floor(5/2) = 1
let start = myCurrentPage.value - Math.floor((myBtnCount.value / 2))
// 终点 = 起点页数 + 总页数 - 1 距离 1 + 5 -1 || 3 + 5 -1
let end = start + myBtnCount.value - 1
// 意外1 当起点小于1
if (start < 1) {
start = 1
// 终点= 当前页数>总页数?总页数 否则 当前页数
end = myBtnCount.value > pageCount ? pageCount : myBtnCount.value
}
// 意外2 当终点大于最大页码
if (end > pageCount) {
end = pageCount
// 起点= 终点+(-所有页数+1)>1?1:= 终点+(-所有页数+1)
start = (end - myBtnCount.value + 1) < 1 ? 1 : (end - myBtnCount.value + 1)
}
const pager = []
for (let i = start; i <= end; i++) {
pager.push(i)
}
return { start, end, pageCount, pager }
})
// 上一页下一页
const go = (step) => {
myCurrentPage.value += step
}
const changePage = (page) => {
// 如果等于现在页页码 保持不动
if (page === myCurrentPage.value) return
myCurrentPage.value = page
emit('currentCahnge', page)
}
// 监听传入的值改变
watch(props, () => {
myTotal.value = props.total
myPageSize.value = props.pageSize
myCurrentPage.value = props.currentPage
myBtnCount.value = props.btnCount
}, { immediate: true })
return { myTotal, myPageSize, myCurrentPage, myBtnCount, pageInfo, go, changePage }
}
}
</script>
<style scoped lang="less">
.xtx-pagination {
display: flex;
justify-content: center;
padding: 30px;
> a {
display: inline-block;
padding: 5px 10px;
border: 1px solid #e4e4e4;
border-radius: 4px;
margin-right: 10px;
&:hover {
color: @xtxColor;
}
&.active {
background: @xtxColor;
color: #fff;
border-color: @xtxColor;
}
&.disabled {
cursor: not-allowed;
opacity: 0.4;
&:hover {
color: #333
}
}
}
> span {
margin-right: 10px;
}
}
</style>