一个类重载了运算符”()”,则该类的对象就成为函数对象
Dev C++中的 Accumulate
源码1
源码2
函数指针和函数对象分别作为参数实例
#include <iostream>
#include <vector>
#include <algorithm>
#include <numeric>
#include <functional>
using namespace std;
int sumSquares(int total, int value) {
return total + value * value;
}
template <class T>
void PrintInterval(T first, T last) {
for(; first != last; ++first)
cout << *first << " ";
cout << endl;
}
template SumPowers {
private:
int power;
public:
SumPowers(int p):power(p) {}
const T operator() (const T & total, const T & value) {
T v = value;
for(int i = 0; i < power - 1; ++i)
v = v * value;
return total + v;
}
};
void main() {
const int SIZE = 10;
int a1[] = { 1,2,3,4,5,6,7,8,9,10 };
vector<int> v(a1, a1+SIZE);
cout << "1) "; PrintInterval(v.begin(), v.end());
int result = accumulate(v.begin(), v.end(), 0, SumSquares);
cout << "2) 平方和: " << result << endl;
result = accumulate(v.begin(), v.end(), 0, SumPowers<int>(3));
cout << "3) 立方和: " << result << endl;
result = accumulate(v.begin(), v.end(), 0, SumPowers<int>(4));
cout << "4) 4次方和: " << result;
}
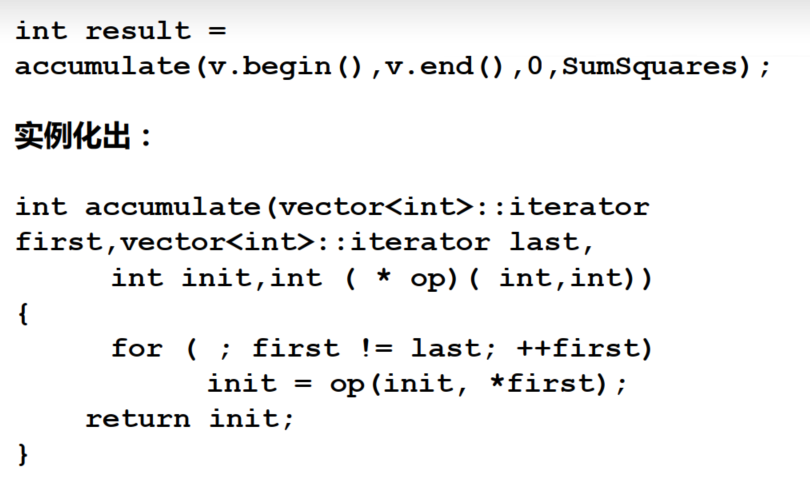
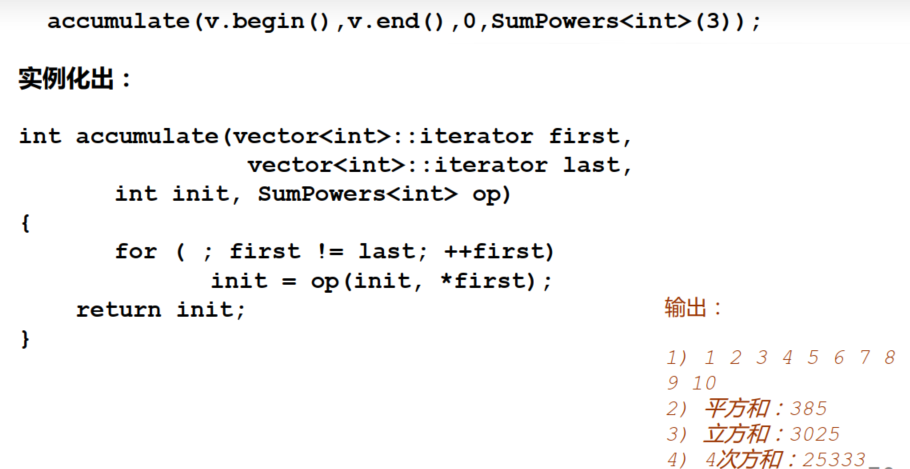
- 函数对象的好处在于,像上例中,容易修改想要累加的幂次这个参数;如果是函数指针想要实现这个功能,就必须使用全局变量或者写很多个这样的二次方三次方…的函数
STL中的函数对象类模板
greater函数对象类
greater应用
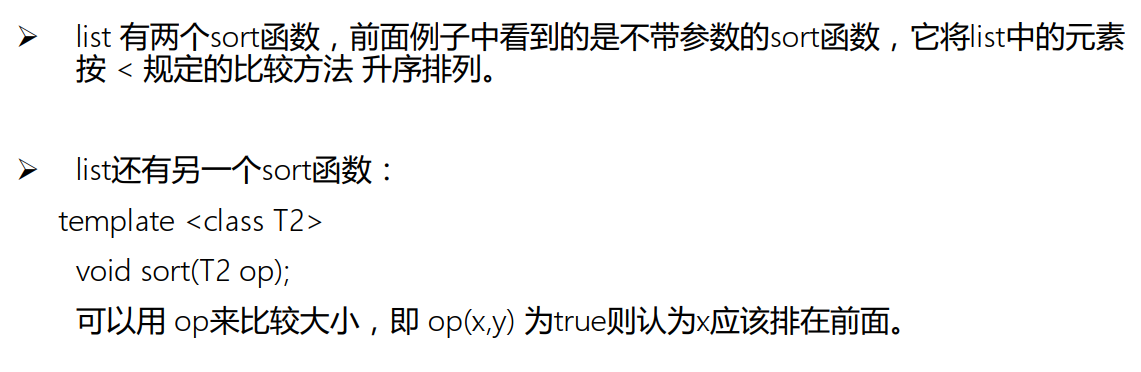
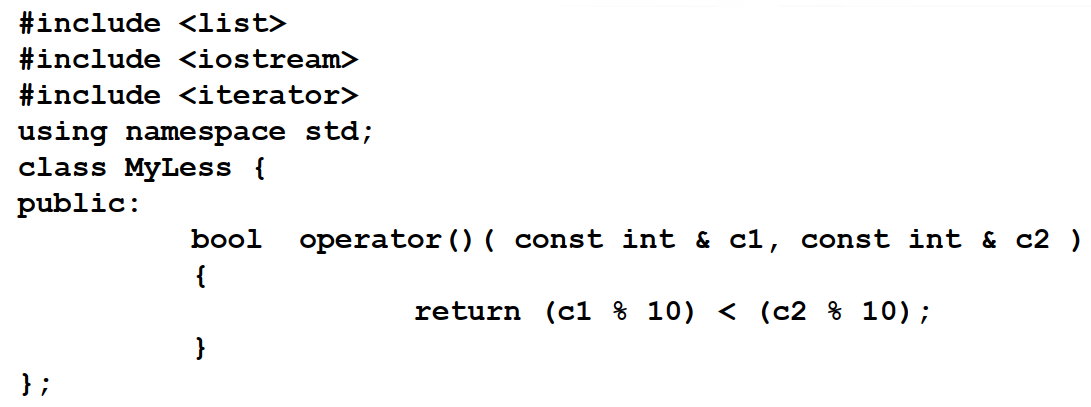
引入函数对象后,STL中“大”“小”关系
实例:MyMax编写
编写MyMax函数,满足如下程序:
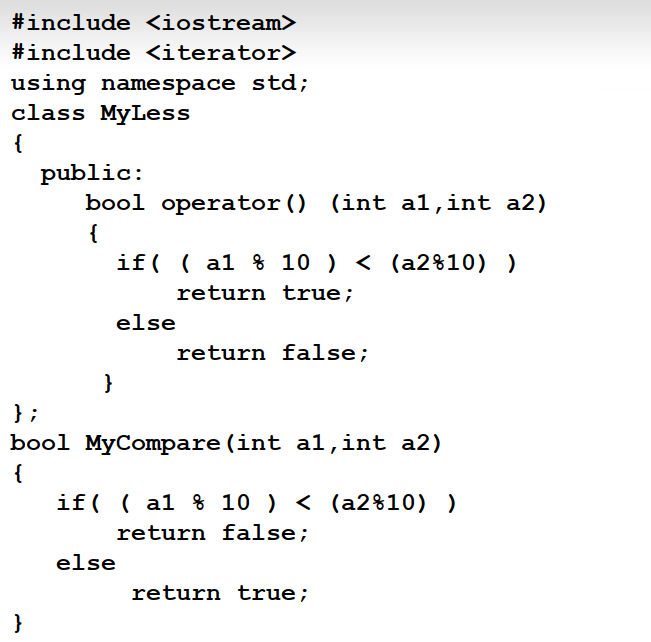
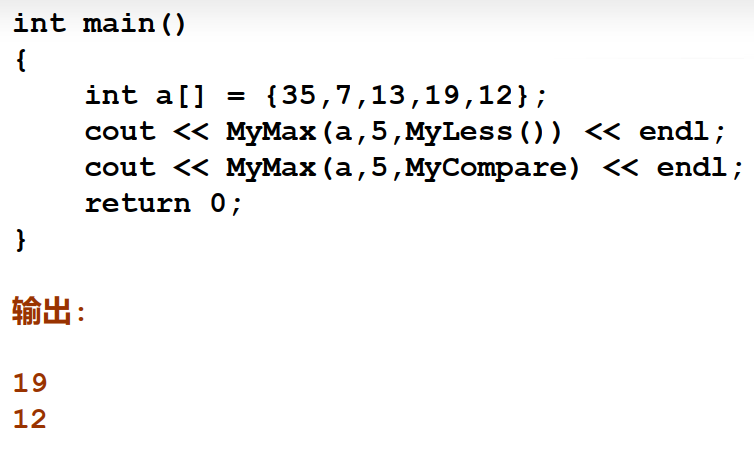
template <class T, class Pred>
T MyMax(T * p, int n, Pred myless) {
T tmp_max = p[0];
for(int i = 1; i < n; ++i) {
if(myless(tmp_max, p[i])
tmp_max = p[i];
}
return p[i];
}