迭代器
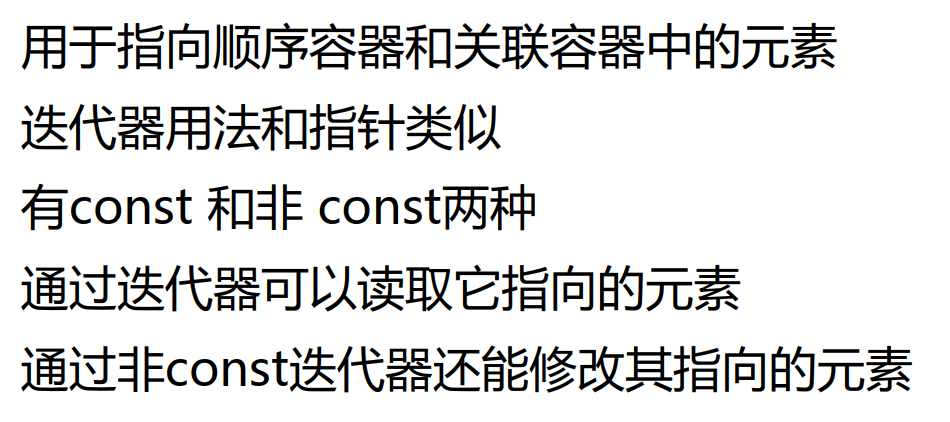
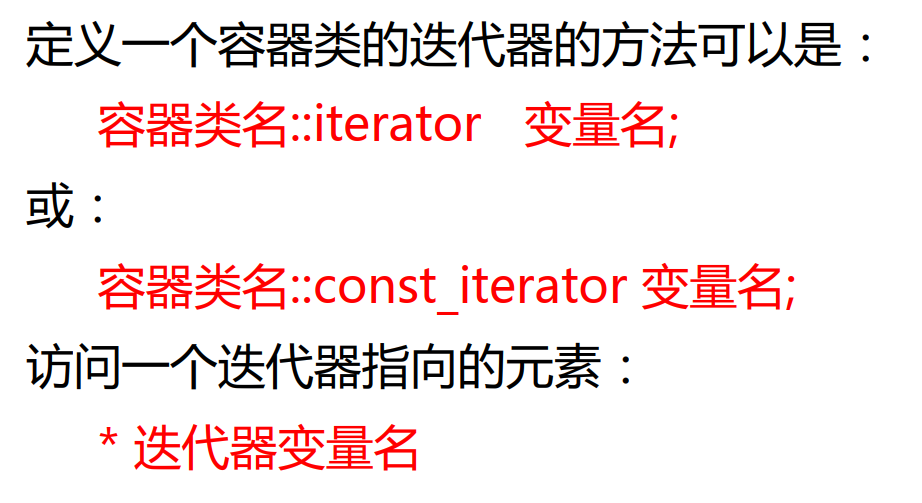

#include <vector>
#include <iostream>
using namespace std;
void main() {
vector<int> v;
v.push_back(1); v.push_back(2); v.push_back(3); v.push_back(4);
vector<int>::const_iterator i; // 常量迭代器
for(i = v.begin(); i != v.end(); ++i)
cout << *i << ",";
cout << endl;
vector<int>::reverse_iterator r; // 反向迭代器
for(r = v.rbegin(); r != vrend(); ++r) // ++r 实际上是往前走
cout << *r << ",";
cout << endl;
vector<int>::iterator j; // 非常量迭代器
for(j = v.begin(); j != v.end(); ++j)
*j = 100;
for(i = v.begin(); i != v.end(); ++i)
cout << *i << ",";
cout << endl;
/* 输出:
1,2,3,4,
4,3,2,1,
100,100,100,100,
*/
}
双向迭代器
随机访问迭代器
不同容器对应迭代器种类
vector的迭代器是随机访问迭代器
算法简介
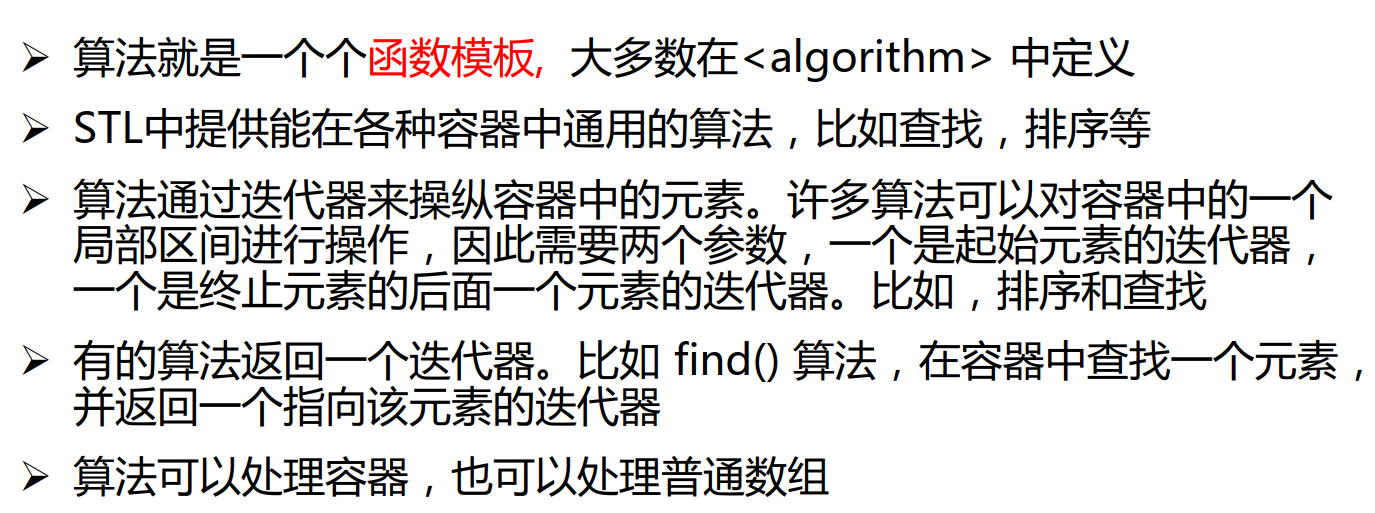
find()模板
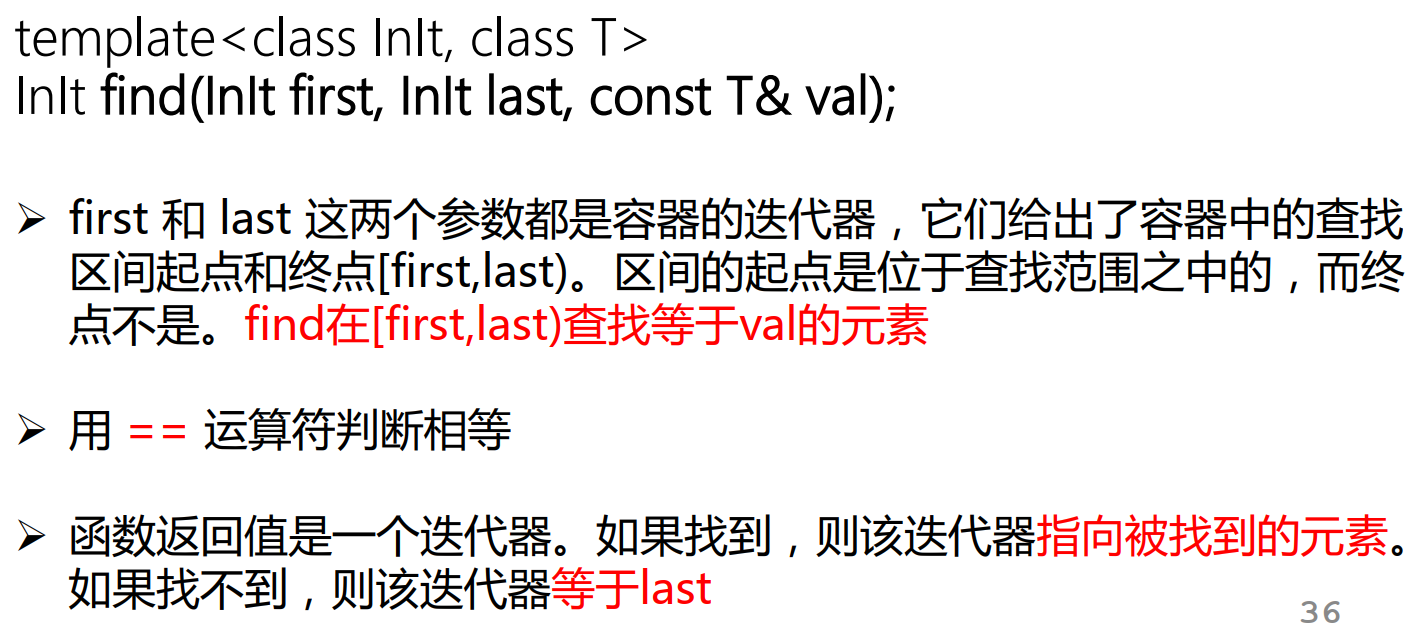
#include <vector>
#include <algorithm>
#include <iostream>
using namespace std;
void main() {
int array[10] = {10, 20, 30, 40};
vector<int> v;
v.push_back(1); v.push_back(2);
v.push_back(3); v.push_back(4);
vecotr<int>::iterator p;
p = find(v.begin(), v.end(), 3);
if(p != v.end())
cout << *p << endl; // 输出3
p = find(v.begin(), v.end(), 9);
if(p == v.end())
cout << "not found" << endl; // 输出 not found
p = find(v.begin()+1, v.end()-2, 1); //整个容器: [1,2,3,4], 查找区间: [2,3)
if(p != v.end())
cout << *p << endl; // 输出3
int *pp = find(array, array+4, 20);
cout << *pp << endl; // 输出20
}
STL中“大”“小”概念
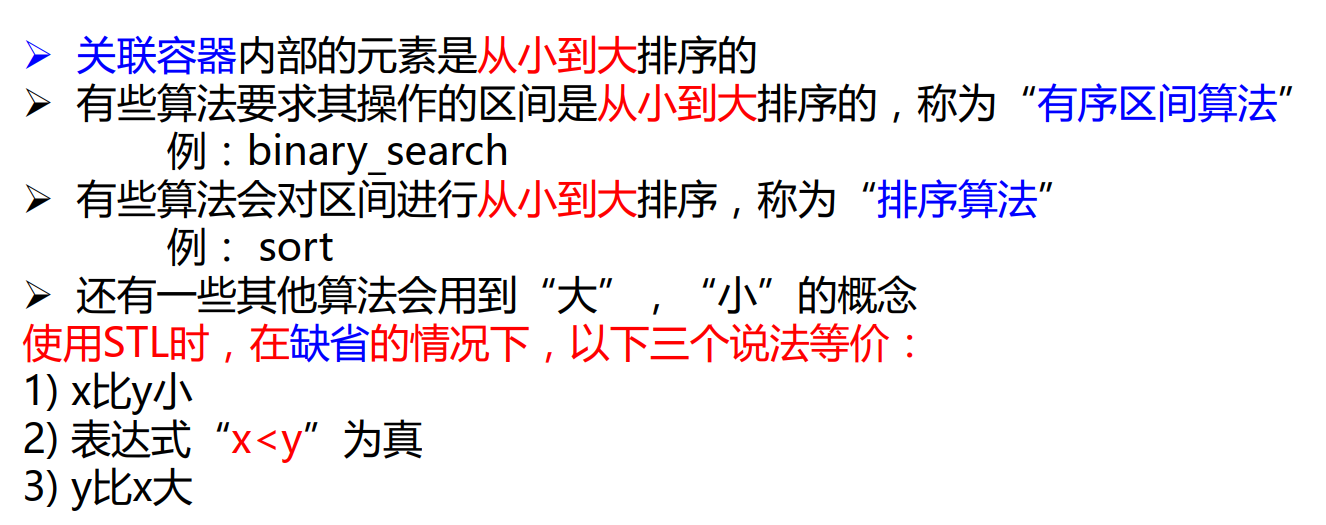
STL中“相等”概念演示
#include <iostream>
#include <algorithm>
using namespace std;
class A {
int v;
public:
A(int n):v(n){}
bool operator < (const A & a) {
cout << v << "<" << a.v << "?" << endl;
return false;
}
bool operator == (const A & a) {
cout << v << "==" << a.v << "?" << endl;
return v == a.v;
}
};
void main() {
A a[] = {A(1), A(2), A(3), A(4), A(5)};
cout << binary_search(a, a+4, A(9));
}
/* 输出:
3<9?
2<9?
1<9?
9<1?
*/