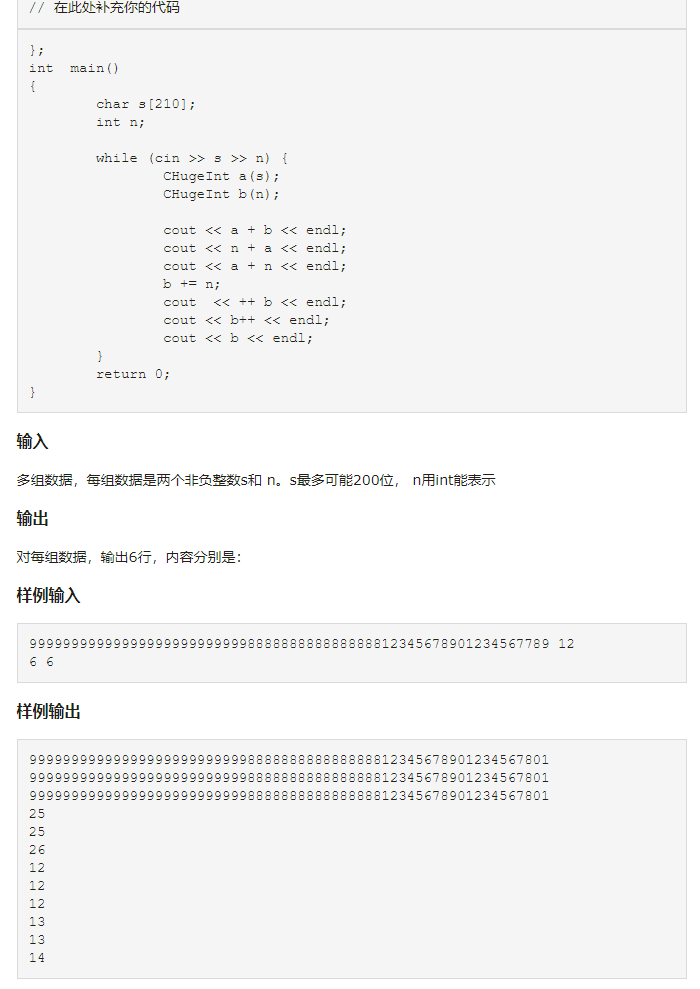
#include <iostream>
#include <cstring>
#include <cstdlib>
#include <cstdio>
using namespace std;
const int MAX = 110;
class CHugeInt
{
public:
int arr[MAX]{0};
int len;
CHugeInt(int n)
{
arr[0] = n;
len = 1;
}
CHugeInt(const char *s)
{
len = strlen(s) / 9 + 1; // 一个int元素最多存9位,因为int是10位,防止溢出
const char *p = s; // 指向第一个数字
int i = len;
// 把高位数存在数组的后面,如果出现高位进位,方便处理
while (i--)
{
for (int j = 0; j < 9 && *p != '\0'; ++j, ++p)
{
arr[i] = arr[i] * 10 + (*p - '0'); // 逐位将字符串读入int数组
}
}
}
CHugeInt(const CHugeInt &hi)
{
memcpy(arr, hi.arr, hi.len * sizeof(int));
len = hi.len;
}
CHugeInt operator+(const CHugeInt &hi) // 把右边的加到左边上
{
int i = 0;
CHugeInt tmp(*this);
int c = 0; // 低位进位
do
{
int sum = tmp.arr[i] + hi.arr[i] + c;
c = sum / 1000000000;
tmp.arr[i] = sum % 1000000000;
++i;
} while ((i <= hi.len) && (i <= tmp.len));
return tmp;
}
void operator+=(int n)
{
int sum = arr[0] + n;
int c = sum / 1000000000;
arr[1] += c;
arr[0] = sum % 1000000000;
}
CHugeInt &operator++()
{
int sum = arr[0] + 1;
if (sum == 1000000000)
{
arr[0] = 0;
arr[1] += 1;
}
else
{
arr[0] = sum;
}
return *this;
}
CHugeInt operator++(int)
{
CHugeInt tmp(*this);
int sum = arr[0] + 1;
if (sum == 1000000000)
{
arr[0] = 0;
arr[1] += 1;
}
else
{
arr[0] = sum;
}
return tmp;
}
friend ostream &operator<<(ostream &os, const CHugeInt &hi)
{
int i = hi.len;
while (i--)
{
os << hi.arr[i];
}
return os;
}
};
CHugeInt operator+(int n, const CHugeInt &hi)
{
CHugeInt tmp(hi);
int sum = tmp.arr[0] + n;
int c = sum / 1000000000;
tmp.arr[1] += c;
tmp.arr[0] = sum % 1000000000;
return tmp;
}
int main()
{
char s[210];
int n;
while (cin >> s >> n)
{
CHugeInt a(s);
CHugeInt b(n);
cout << a + b << endl;
cout << n + a << endl;
cout << a + n << endl;
b += n;
cout << ++b << endl;
cout << b++ << endl;
cout << b << endl;
}
return 0;
}
- 考察各种运算符的重载,“+”、自增运算符、流输出运算符。需要注意以下几点:
- memcpy()函数最后一个参数是拷贝的字节数,所以一般用n * sizeof()作为该参数的实参
- 前置“++”要返回自己引用,后置“++”别忘了自己加完了,返回加之前的自己的临时对象
- “+=”运算符也是需要重载的