预备知识:pair模板
template <class _T1, class _T2>
struct pair {
typedef _T1 first_type;
typedef _T2 second_type;
_T1 first;
_T2 second;
pair():first(), second(){}
pair(const _T1 & __a, const _T2 & __b) : first(__a), second(__b){}
template<class _U1, class _U2>
pair(const pair<_U1, class _U2> & __p): first(__p.first), second(__p.second){}
};
// map/multimap容器里面放的都是pair模板类的对象,且按照first从小到大排序
// 第三个构造函数用法示例:
pair<int, int> p(pair<double, double> (5.5, 4.6));
// p.first = 5, p.second = 4.6
multiset
multiset成员函数
用法示例
- 错误示例
```cpp
include
using namespace; class A{}; int main() { multiset a; a.insert(A()); // error }
/*
- 正确示例
```cpp
include
include
using namespace std; templatevoid Print(T first, T last) { for(; first != last; ++first)
cout << endl; } class A { private:cout << *first << " ";
public: A(int n):n(n){} friend bool operator < (const A & a1, const A & a2) {return a1.n < a2.n;} friend ostream & operator << (ostream & o, const A a) {int n;
} friend class MyLess; };o << a.n;
return o;
strcut MyLess {
bool operator()(const A & a1, const A & a2) {return (a1.n & 10 < (a2.n % 10);}
};
typedef multiset MSET1; // 用”<”比较大小 typedef multiset MSET2; // 用MyLess比较大小
void main() { const int SIZE = 6; A a[SIZE] = {4, 22, 19, 8, 33, 40}; MSET1 m1; m1.insert(a, a + SIZE); m1.insert(22); cout << m1.count(22) << endl; // 输出 2 Print(m1.begin(), m1.end()); // 输出 4 8 19 22 22 33 40 // m1元素:4 8 19 22 22 33 40 MSET1:iterator pp = m1.find(19); if(pp != m1.end()) // 条件为真说明找到了 cout << “found” << endl; // 输出 found // 输出 22,33 cout << m1.lower_bound(22) << “,” << m2.upper_bound(22) << endl; // pp指向被删除元素的下一个元素 pp = m1.erase(m1.lower_bound(22), m1.upper_bound(22)); Print(m1.begin(), m1.end()); // 输出 4 8 19 33 40 cout << *pp << endl; // 输出 33
MSET2 m2; // m2中的元素按照个位大小从小到大排序
m2.insert(a, a+SIZE);
Print(m2.begin(), m2.end()); // 输出 40 22 33 4 8 19
}
<a name="QS5gt"></a>
# set
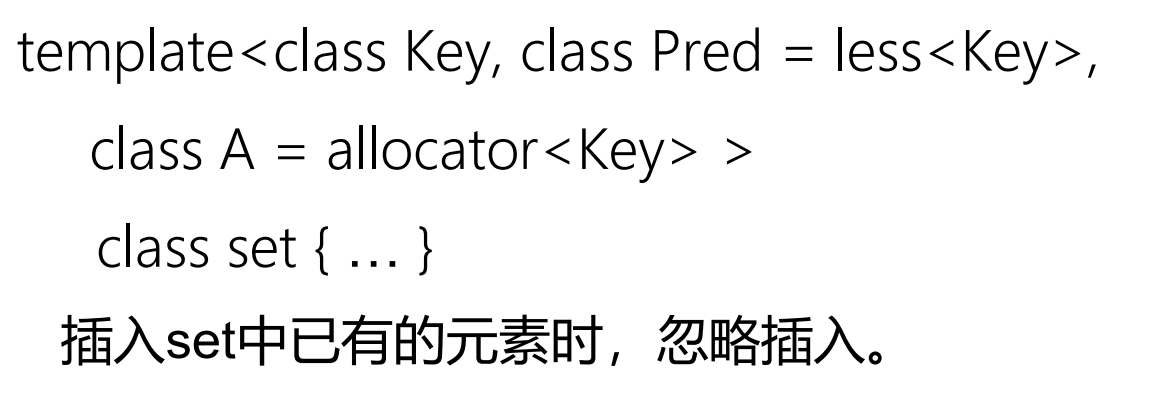
- 重复的意思是 "a < b" 和 "b < a"都不成立
```cpp
#include <iostream>
#include <set>
using namespace std;
void main() {
typedef set<int>::iterator IT;
int a[5] = {3,4,6,1,2};
set<int> st(a, a+5); // 这里是 1 2 3 4 6
pair<IT, bool> result;
result = st.insert(5); // st变成 1 2 3 4 5 6
if(result.second)
cout << *result.first << "inserted" << endl; // 输出:5 inserted
if(st.insert(5).second)
cout << *result.first << endl;
else
cout << *result.first << " already exists" << endl; // 输出 5 already exists
pair<IT, IT> bounds = st.equal_range(4);
cout << *bound.first << "," << *bounds.second; // 输出: 4,5
}