1 线程的创建
- Java 从语言级别支持多线程
- 如 Object 中的
wait()
和 notify()
- Java.lang 中的类
Thread
** ** - 线程体 —-
run()
1.1 继承 Thread 类
class MyThread extends Thread {
public void run() {
for (int i = 0; i < 100; ++i) {
System.out.println(" " + i);
}
}
}
1.2 向 Thread() 构造方法传递 Runnable 对象
class MyTack implements Runnable {
public void run() {
}
}
MyTask mytask = new MyTask();
Thread thread = new Thread(mytask);
thread.start();
1.3 使用匿名类
new Thread() {
public void run() {
for (int i = 0; i < 100; ++i)
System.out.println(i);
}
}
1.4 使用 Lambda 表达式
new Thread(() -> {
// ...
}).start();
2 线程的控制
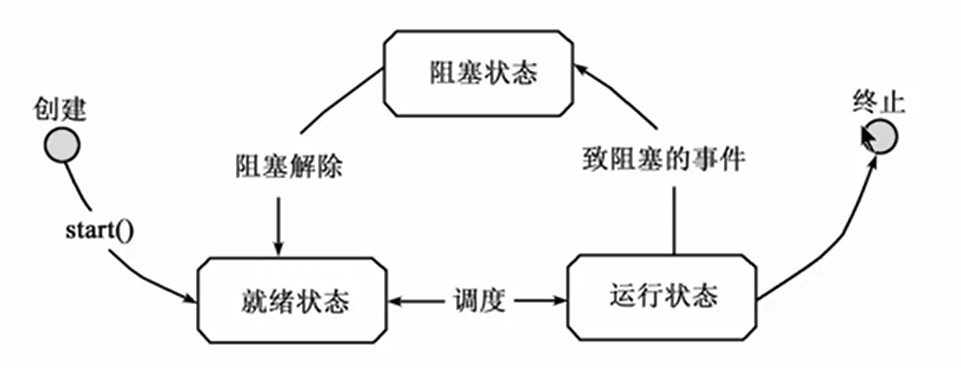
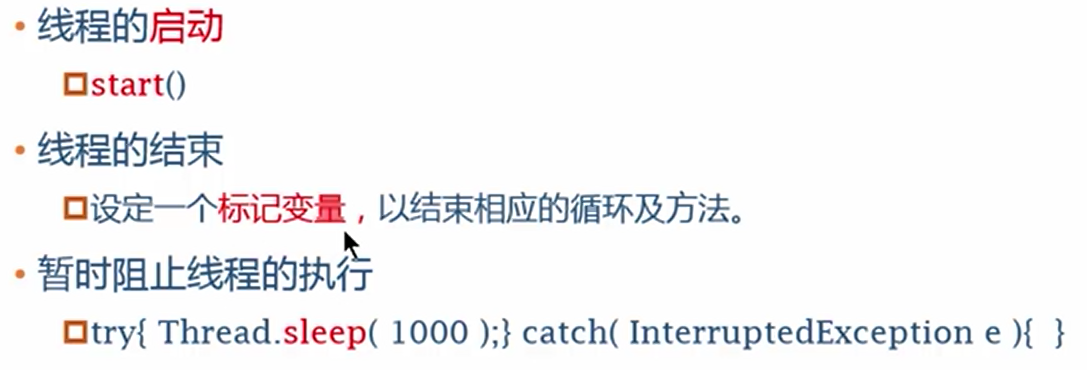

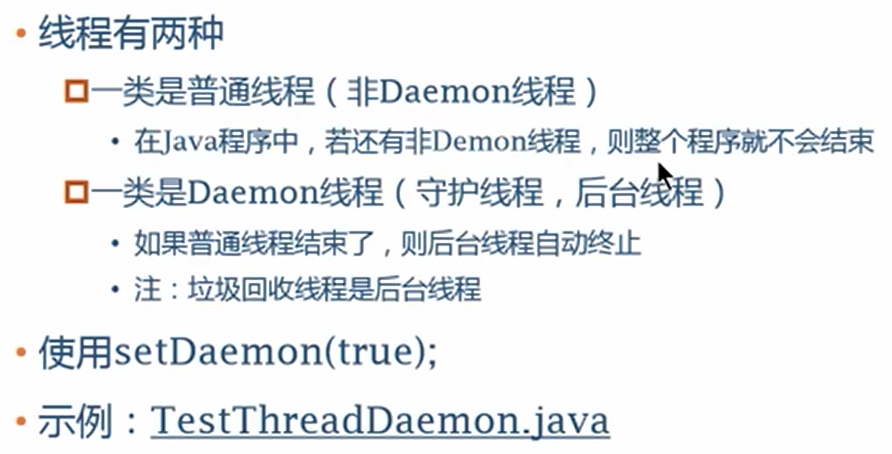
3 线程的同步
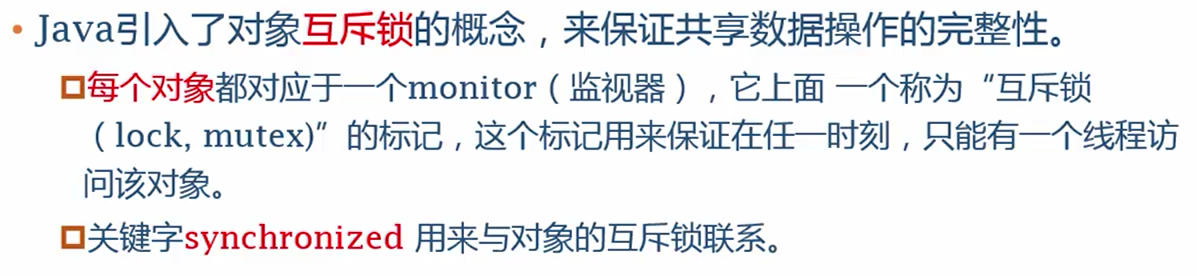
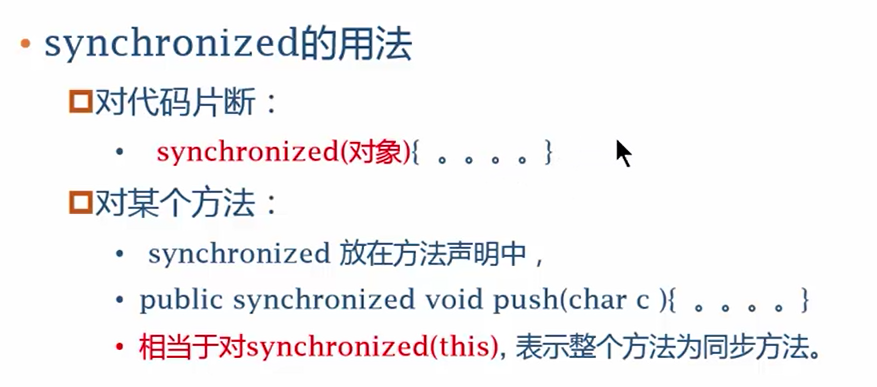
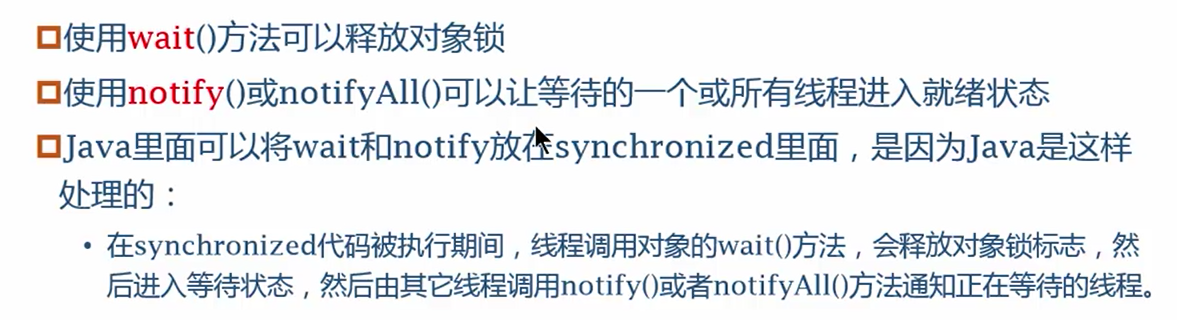
wait()
和 notify()
相当于操作系统中的 P
操作和 V
操作
class CubbyHole {
public int index = 0;
publin int[] data = new int[3];
public synchronized void put(int value) {
while (index == data.length) {
try {
this.wait();
} catch (InterruptedException e) {}
}
data[index] = value;
index++;
this.notify();
}
public synchronized int get() {
while (index <= 0) {
try {
this.wait();
} catch (InterruptedException e) {}
}
index--;
int val = data[index];
this.notify();
return val;
}
}
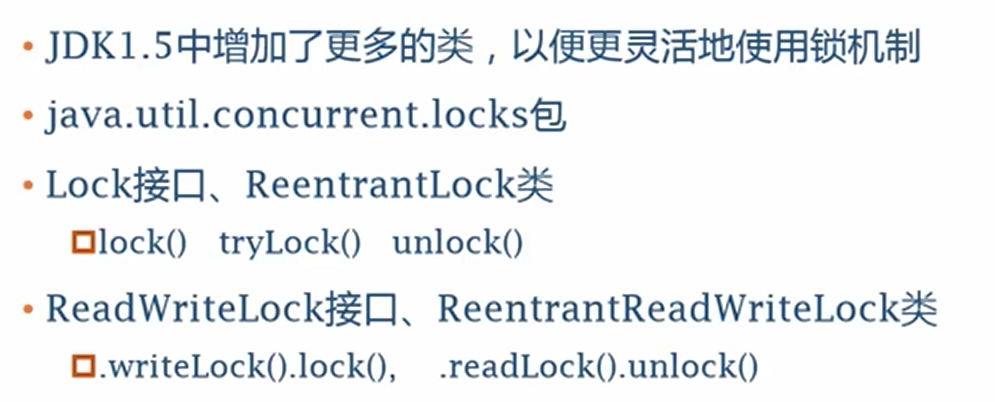