text
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: MyHomePage(title: '傻╮(╯▽╰)╭'),
);
}
}
class MyHomePage extends StatefulWidget {
MyHomePage({Key key, this.title}) : super(key: key);
final String title;
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
int _counter = 0;
void _incrementCounter() {
setState(() {
_counter+=2;
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text(
'假设生命倒计时是 $_counter 天 倒计时假装生命倒计时是 $_counter 天假装生命倒计时假装生命倒计时是 天假装生命倒时假装生命倒计时是3天假装生命倒计时',
textAlign: TextAlign.center,//居中对齐 .left .right 对齐 还有start end
maxLines: 2,//最大显示行数
overflow: TextOverflow.ellipsis,//TextOverflow.ellipsis结尾多余部分显示3个点,fade 渐变消失(垂直)
style: TextStyle(
fontSize: 26.0,
color: Color.fromARGB(200, 250, 120, 120),//第一个是透明度后3是rjb
decoration: TextDecoration.underline,//下划线
decorationStyle: TextDecorationStyle.solid,//实线
),
),
Text(
'$_counter 剩下',
style: TextStyle(
fontFamily: '微软雅黑',
fontSize: 30.0,
color: Color.fromARGB(255, 10, 200, 100)
),
),
Text(
'就乖乖学习',
),
],
),
),
floatingActionButton: FloatingActionButton(
onPressed: _incrementCounter,
tooltip: 'Increment',
child: Icon(Icons.add),
),
);
}
}
container
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.yellow,
),
home: Scaffold(
appBar: AppBar(
title: Text('hhh'),
),
body: Center(
child: Container(
child: new Text('hi dwh', style: TextStyle(fontSize: 28.0,color: Colors.blue),),
alignment: Alignment.topLeft,
width: 300,
height: 300,
//color: Colors.black,
padding: const EdgeInsets.fromLTRB(20, 30, 0, 0),//内边距
margin: const EdgeInsets.all(10),//外边距
decoration: new BoxDecoration(
gradient: const LinearGradient(//线性渐变
colors: [Colors.lightGreenAccent,Colors.lightGreen,Colors.pink]
),
border: Border.all(width: 2.0, color: Colors.blue,)//边框
),
),
),
),
);
}
}
引用外部Image
home: Scaffold(
appBar: AppBar(
title: Text('hhhh'),
),
body: Center(
child: Container(
child: Container(
child: new Image.network(
'https://img.isharebest.com/2018041509.jpg',
fit: BoxFit.contain,
//尽量充满,维持比例,fill全充满,
//fitWidth 横向充满,纵向多余裁切
//cover 图片不变形 四周裁切,scaleDown图片大小不变
)
),
width: 500.0,
height: 350.0,
color: Colors.yellow,
padding: const EdgeInsets.all(10),
),
),
),
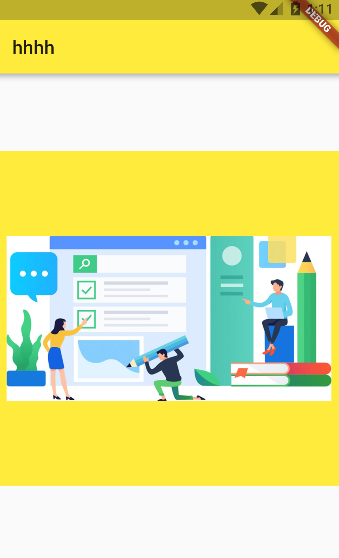
child: Container(
child: new Image.network(
'https://img.isharebest.com/2018041509.jpg',
fit: BoxFit.contain,
color: Colors.lightGreen,
colorBlendMode: BlendMode.darken,//颜色混合
//colorBlendMode: BlendMode.colorBurn //增强对比度
//colorBlendMode: BlendMode.colorDodge//提升曝光?
)
),
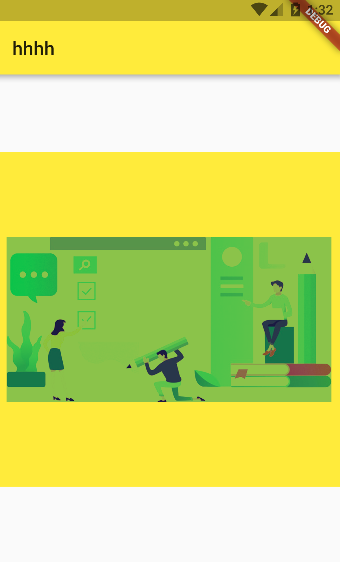
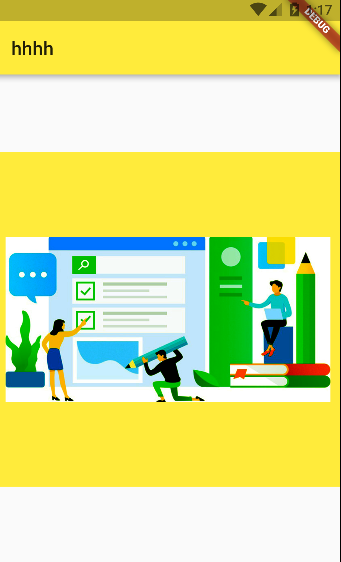
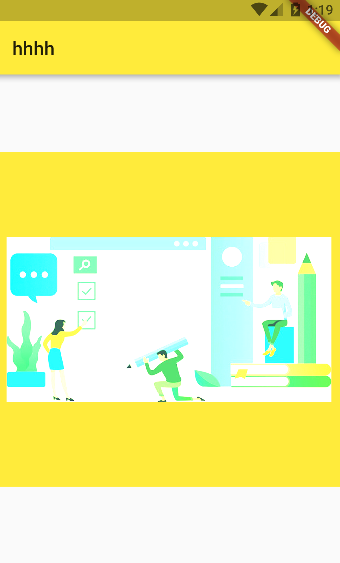
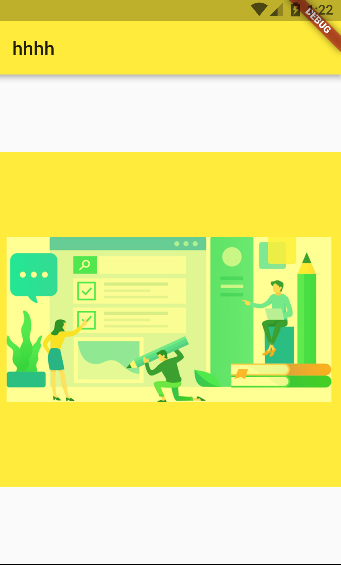