定义
在一个程序中,该类只创建一个实例对象。
类图
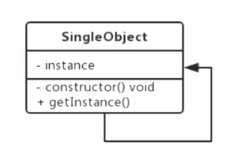
代码
基本的单例模式
class Window {
private static instance: Window;
private constructor() {}
static getInstance() {
if (!Window.instance) {
Window.instance = new Window();
}
return Window.instance;
}
}
let w1 = Window.getInstance();
let w2 = Window.getInstance();
console.log(w1 === w2);
单例与构建过程分离
function Window() {}
let createInstance = (function () {
let instance: Window;
return function () {
if (!instance) {
instance = new Window();
}
return instance;
};
})();
let w1 = createInstance();
let w2 = createInstance();
console.log(w1 === w2);
封装变化,可以创建任何类型实例
function Window() {}
function Modal() {}
let createInstance = function (Constructor: any) {
let instance: any;
return function (this: any) {
if (!instance) {
Constructor.apply(this, arguments);
Object.setPrototypeOf(this, Constructor.prototype);
instance = this;
}
return instance;
};
};
let createWindow = createInstance(Window);
let createModal = createInstance(Modal);
let w2 = new (createWindow as any)();
let w1 = new (createWindow as any)();
let m1 = new (createModal as any)();
let m2 = new (createModal as any)();
console.log(w1 === w2);
console.log(m1 === m2);
模态框实例
<button id="show">显示窗口</button>
<button id="hide">隐藏窗口</button>
<script>
class LoginModal {
static instance
constructor(id) {
this._element = document.createElement("div")
this._element.innerHTML = `
<form>用户名<input /><button type='submit'>提交</button></form>
`
this._element.style.cssText = 'border-radius:10px;border:3px solid #333;width:200px;height:100px;position: absolute;top:45%;left:45%;'
document.body.appendChild(this._element)
}
show() {
this._element.style.display = "block"
}
hide() {
this._element.style.display = 'none'
}
static getInstance() {
if (!LoginModal.instance) {
LoginModal.instance = new LoginModal()
}
return LoginModal.instance
}
}
document.getElementById("show").addEventListener("click", function () {
LoginModal.getInstance().show()
})
document.getElementById("hide").addEventListener("click", function () {
LoginModal.getInstance().hide()
})
服务端使用缓存cache
let express = require("express");
let app = express();
let fs = require("fs");
// 缓存一定要写成单例
let cache = {};
app.get("/api/users/:id", function (req, res) {
let id = req.params.id;
if (cache[id]) {
res.json(cache[id]);
} else {
fs.readFile(`./users/${id}.json`, "utf8", (err, data) => {
let user = JSON.parse(data);
res.json(user);
});
}
});