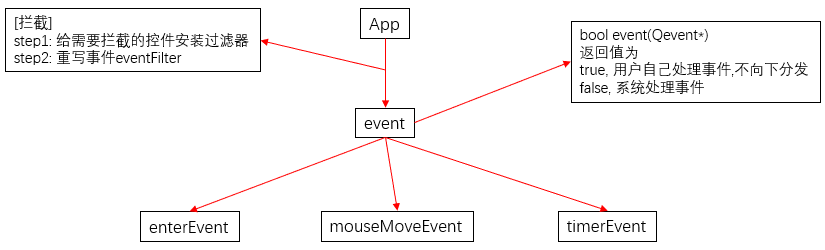
bool MyLabel::event(QEvent *e)
{
QMouseEvent *ev = static_cast<QMouseEvent*>(e);
if(e->type()==QEvent::MouseButtonPress) // 只有鼠标按下自己处理
{
QString str = QString("Event:鼠标按下了,x=%1,y=%2").arg(ev->x()).arg(ev->y());
qDebug() << str;
return true; // 返回值 是 true代表用户自己处理
}
// 其它事件,让父类做默认处理
return QLabel::event(e); // 返回值为false代表让系统处理,最好是让父类去处理
}
// widget.h
class Widget : public QWidget
{
Q_OBJECT
public:
Widget(QWidget *parent = nullptr);
~Widget();
private:
Ui::Widget *ui;
// 定时器事件
void timerEvent(QTimerEvent*);
// 定时器ID
int id1;
int id2;
// step2:重写事件过滤器
bool eventFilter(QObject*,QEvent*);
};
// widget.cpp step1:给ui->label安装事件过滤器
Widget::Widget(QWidget *parent)
: QWidget(parent)
, ui(new Ui::Widget)
{
ui->setupUi(this);
// 启动定时器 法一
id1 = startTimer(1000);
id2 = startTimer(2000);
// 启动定时器 法二
QTimer* timer1 = new QTimer(this);
timer1->start(500); // 每隔0.5秒发送信号
connect(timer1,&QTimer::timeout,[=](){
static int num = 0;
ui->label_4->setText(QString::number(num++));
});
// 点击按钮 暂停定时器
connect(ui->pushButton,&QPushButton::clicked,[=](){
timer1->stop();
});
// 给ui->label安装事件过滤器
ui->label->installEventFilter(this); // step1:安装过滤器
}
// widget.cpp step2:重写事件过滤器
bool Widget::eventFilter(QObject *obj, QEvent *e)
{
if(obj == ui->label)
{
if(e->type() == QEvent::MouseButtonPress)
{
QMouseEvent* ev = static_cast<QMouseEvent*>(e);
QString str = QString("Event Filter:鼠标按下了,x=%1,y=%2").arg(ev->x()).arg(ev->y());
qDebug() << str;
return true; // 返回值 是 true代表用户自己处理
}
}
return QWidget::eventFilter(obj,e);
}