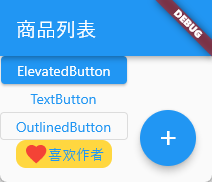
import 'package:flutter/material.dart';
main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: HomePage(),
);
}
}
class HomePage extends StatelessWidget {
@override
Widget build(BuildContext context) {
print("call HomePage build");
return Scaffold(
appBar: AppBar(
title: Text("商品列表"),
),
body: HomeContent(),
// 右下角按钮
floatingActionButton: FloatingActionButton(
child: Icon(Icons.add),
onPressed: () => print("clicked"),
),
);
}
}
class HomeContent extends StatefulWidget {
@override
_HomeContentState createState() => _HomeContentState();
}
class _HomeContentState extends State<HomeContent> {
@override
Widget build(BuildContext context) {
return Column(
children: [
// 其它按钮
ElevatedButton(
onPressed: () => {print("ElevatedButton")},
child: Text("ElevatedButton")),
TextButton(
onPressed: () => {print("TextButton")}, child: Text("TextButton")),
OutlinedButton(
onPressed: () => {print("OutlinedButton")},
child: Text("OutlinedButton")),
// 自定义按钮
TextButton(
style: ButtonStyle(
backgroundColor: MaterialStateProperty.all(Colors.amberAccent),
// 背景色
shape: MaterialStateProperty.all<OutlinedBorder>(
RoundedRectangleBorder(
borderRadius: BorderRadius.circular(10)))),
onPressed: () {},
child: Row(
mainAxisSize: MainAxisSize.min,
children: [
Icon(
Icons.favorite,
color: Colors.red,
),
Text("喜欢作者")
],
),
)
],
);
}
}