1 标准对话框
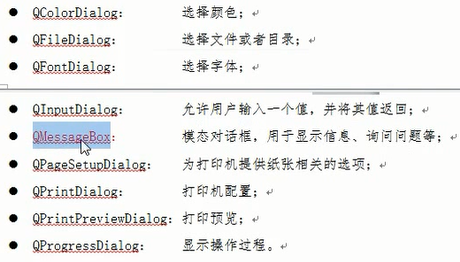
// 消息框
MainWindow::MainWindow(QWidget *parent)
: QMainWindow(parent)
, ui(new Ui::MainWindow)
{
ui->setupUi(this);
connect(ui->actionNew,&QAction::triggered,this,[=]()
{
//QMessageBox::critical(this,"错误","critical");
//QMessageBox::information(this,"信息","info");
// if(QMessageBox::question(this,"问题","question",QMessageBox::Save|QMessageBox::Cancel,
// QMessageBox::Cancel) == QMessageBox::Save)
// {
// qDebug() << "点击的是保存";
// }
// else
// {
// qDebug() << "点击的是取消";
// }
QMessageBox::warning(this,"警告","warning");
});
}
// 颜色对话框 与 文件对话框
MainWindow::MainWindow(QWidget *parent)
: QMainWindow(parent)
, ui(new Ui::MainWindow)
{
ui->setupUi(this);
connect(ui->actionNew,&QAction::triggered,this,[=]()
{
// 选择颜色对话框
//QColor cr = QColorDialog::getColor(QColor(255,0,0));
//qDebug() << cr.red() << cr.green() << cr.blue(); // 170 85 255
// 选择文件对话框
QString path = QFileDialog::getOpenFileName(this,"请选择你打开的文件","C:/Users/16481/Desktop","(*.txt *.jpg)");
qDebug() << path; // "C:/Users/16481/Desktop/新建位图图像.jpg"
});
}
2 自定义对话框
MainWindow::MainWindow(QWidget *parent)
: QMainWindow(parent)
, ui(new Ui::MainWindow)
{
ui->setupUi(this);
//点击新建菜单项弹出对话框
connect(ui->actionNew,&QAction::triggered,this,[=]()
{
// QDialog dlg(this);
// dlg.resize(200,100);
// dlg.exec(); //以模态显示对话框
//QDialog dlg2(this); //非模态显示对话框,要创建到堆上
QDialog* dlg2 = new QDialog(this);
dlg2->resize(200,100);
dlg2->show();
dlg2->setAttribute(Qt::WA_DeleteOnClose); //设置窗口属性:关闭窗口后销毁对象
});
}
3 自定义消息框
from PySide2.QtWidgets import QMessageBox, QApplication, QWidget, QPushButton, QAbstractButton
from PySide2.QtCore import QTimer
import sys
class TimeMsgBox(QMessageBox):
def __init__(self, title: str, text: str, timeout=5):
super().__init__()
self.timeout = timeout
# self.setIcon(QMessageBox.Warning) # 设置图标后会有提示音
self.setWindowTitle(title)
self.setText(text)
self.btn_accept = QPushButton(f"确 定 ({self.timeout})")
self.btn_reject = QPushButton("取 消")
self.addButton(self.btn_accept, QMessageBox.ButtonRole.AcceptRole)
self.addButton(self.btn_reject, QMessageBox.ButtonRole.RejectRole)
self.timer = QTimer(self)
self.timer.timeout.connect(self.on_timer_timeout)
self.timer.start(1000)
def on_timer_timeout(self):
self.timeout -= 1
self.btn_accept.setText(f"确 定 ({self.timeout})")
if self.timeout <= 0:
self.btn_accept.click()
class Example(QWidget):
def __init__(self):
super().__init__()
btn = QPushButton("Button", self)
btn.resize(btn.sizeHint())
btn.move(50, 50)
self.setWindowTitle("Example")
btn.clicked.connect(self.warning)
def warning(self):
msgBox = TimeMsgBox("天星科技", "定时关机时间到, 是否关机?")
ret = msgBox.exec_()
if msgBox.clickedButton() == msgBox.btn_accept:
print("1")
else:
print("2")
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = Example()
ex.show()
sys.exit(app.exec_())