1 TextField
TextField用于接收用户的文本输入
- keyboardType键盘的类型,
- style设置样式,
- textAlign文本对齐方式,
- maxLength最大显示行数等等;
- decoration:用于设置输入框相关的样式
- icon:设置左边显示的图标
- labelText:在输入框上面显示一个提示的文本
- hintText:显示提示的占位文字
- border:输入框的边框,默认底部有一个边框,可以通过InputBorder.none删除掉
- filled:是否填充输入框,默认为false
- fillColor:输入框填充的颜色
- onChanged:监听输入框内容的改变,传入一个回调函数
- onSubmitted:点击键盘中右下角的down时,会回调的一个函数
(1) 样式, 监听函数
```dart class HomeContent extends StatelessWidget { @override Widget build(BuildContext context) { return Container(
); } }padding: EdgeInsets.all(20),
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
TextFieldDemo()
],
),
class TextFieldDemo extends StatefulWidget { @override _TextFieldDemoState createState() => _TextFieldDemoState(); }
class _TextFieldDemoState extends State
<a name="JANeG"></a>
## (2) controller
我们可以给TextField添加一个控制器(Controller),可以使用它设置文本的初始值,也可以使用它来监听文本的改变;<br />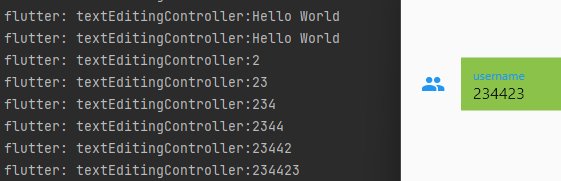
```dart
class TextFieldDemo extends StatefulWidget {
@override
_TextFieldDemoState createState() => _TextFieldDemoState();
}
class _TextFieldDemoState extends State<TextFieldDemo> {
final textEditingController = TextEditingController();
@override
void initState() {
super.initState();
// 1.设置默认值
textEditingController.text = "Hello World";
// 2.监听文本框
textEditingController.addListener(() {
print("textEditingController:${textEditingController.text}");
});
}
@override
Widget build(BuildContext context) {
return TextField(
controller: textEditingController, // 设置controller
decoration: InputDecoration(
icon: Icon(Icons.people),
labelText: "username",
hintText: "请输入用户名",
border: InputBorder.none,
filled: true,
fillColor: Colors.lightGreen
),
);
}
}
2 FormField
(1) 基本使用
class FormDemo extends StatefulWidget {
@override
_FormDemoState createState() => _FormDemoState();
}
class _FormDemoState extends State<FormDemo> {
final registerFormKey = GlobalKey<FormState>();
String? username, password;
void registerForm() {
registerFormKey.currentState?.save();
print("username:$username password:$password");
}
@override
Widget build(BuildContext context) {
return Form(
key: registerFormKey,
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
TextFormField(
decoration: InputDecoration(
icon: Icon(Icons.people),
labelText: "用户名或手机号"
),
onSaved: (value) {
username = value;
},
),
TextFormField(
obscureText: true,
decoration: InputDecoration(
icon: Icon(Icons.lock),
labelText: "密码"
),
onSaved: (value) {
password = value;
},
),
SizedBox(height: 16,),
Container(
width: double.infinity,
height: 44,
child: RaisedButton(
color: Colors.lightGreen,
child: Text("注 册", style: TextStyle(fontSize: 20, color: Colors.white),),
onPressed: registerForm
),
)
],
),
);
}
}
(2) 验证表单数据
- 点击按钮时验证
- 失去焦点时自动验证