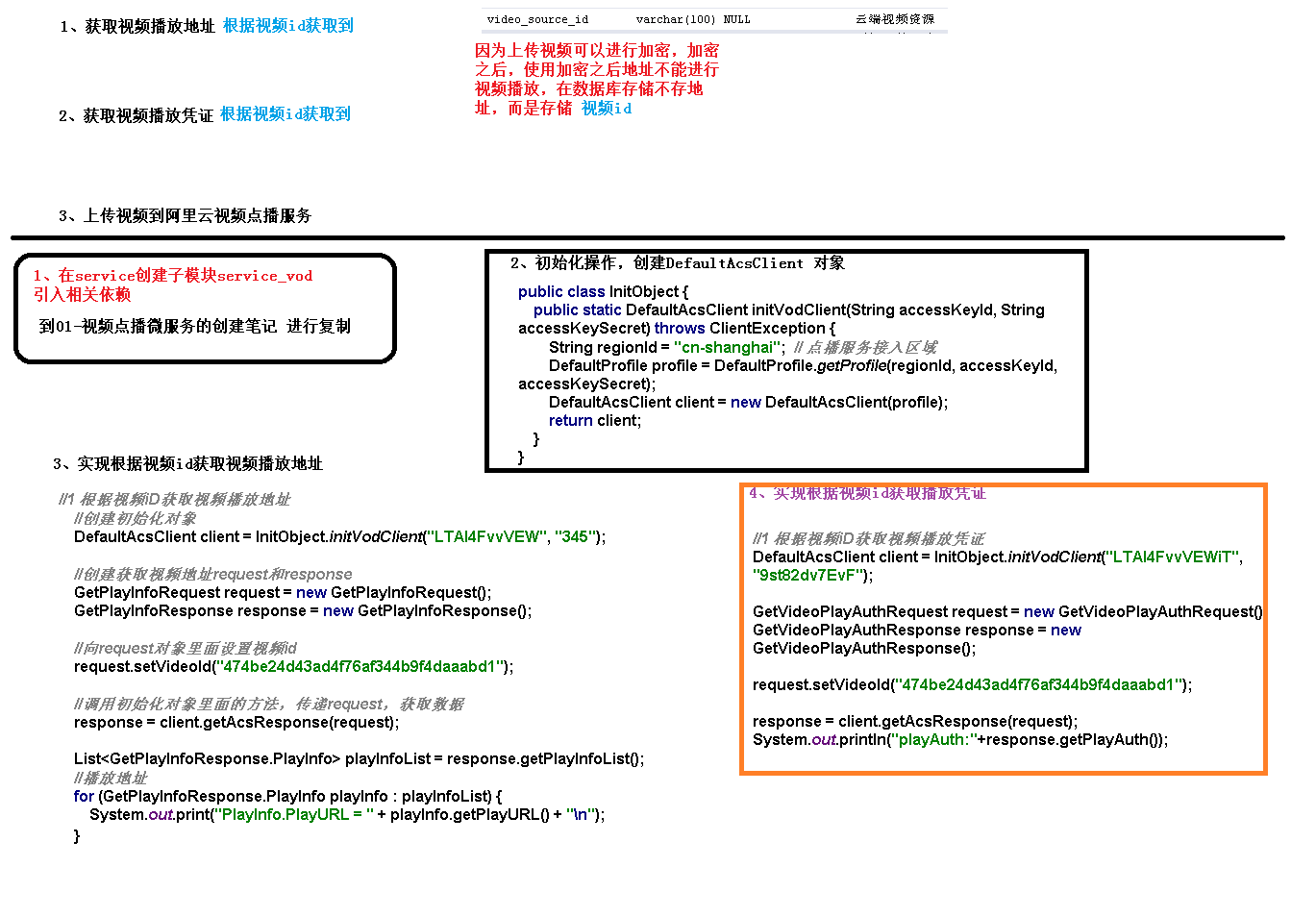
一、服务端SDK
1、简介
sdk的方式将api进行了进一步的封装,不用自己创建工具类。
我们可以基于服务端SDK编写代码来调用点播API,实现对点播产品和服务的快速操作。
2、功能介绍
SDK封装了对API的调用请求和响应,避免自行计算较为繁琐的 API签名。
支持所有点播服务的API,并提供了相应的示例代码。
支持7种开发语言,包括:Java、Python、PHP、.NET、Node.js、Go、C/C++。
通常在发布新的API后,我们会及时同步更新SDK,所以即便您没有找到对应API的示例代码,也可以参考旧的示例自行实现调用。
二、使用SDK
1、安装
参考文档:https://help.aliyun.com/document_detail/57756.html
添加maven仓库的配置和依赖到pom ```javacom.aliyun aliyun-java-sdk-core 4.3.3 com.aliyun aliyun-java-sdk-vod 2.15.5 com.google.code.gson gson 2.8.2
<a name="BKqqD"></a>
## 2、初始化
参考文档:[https://help.aliyun.com/document_detail/61062.html](https://help.aliyun.com/document_detail/61062.html)<br />根据文档示例创建 AliyunVODSDKUtils.java
```java
package com.atguigu.aliyunvod.util;
public class AliyunVodSDKUtils {
public static DefaultAcsClient initVodClient(String accessKeyId, String accessKeySecret) throws ClientException {
String regionId = "cn-shanghai"; // 点播服务接入区域
DefaultProfile profile = DefaultProfile.getProfile(regionId, accessKeyId, accessKeySecret);
DefaultAcsClient client = new DefaultAcsClient(profile);
return client;
}
}
3、创建测试类
创建 VodSdkTest.java
package com.atguigu.aliyunvod;
public class VodSdkTest {
String accessKeyId = "你的accessKeyId";
String accessKeySecret = "你的accessKeySecret";
}
三、创建测试用例
参考文档:https://help.aliyun.com/document_detail/61064.html
1、获取视频播放凭证
根据文档中的代码,修改如下
/**
* 获取视频播放凭证
* @throws ClientException
*/
@Test
public void testGetVideoPlayAuth() throws ClientException {
//初始化客户端、请求对象和相应对象
DefaultAcsClient client = AliyunVodSDKUtils.initVodClient(accessKeyId, accessKeySecret);
GetVideoPlayAuthRequest request = new GetVideoPlayAuthRequest();
GetVideoPlayAuthResponse response = new GetVideoPlayAuthResponse();
try {
//设置请求参数
request.setVideoId("视频ID");
//获取请求响应
response = client.getAcsResponse(request);
//输出请求结果
//播放凭证
System.out.print("PlayAuth = " + response.getPlayAuth() + "\n");
//VideoMeta信息
System.out.print("VideoMeta.Title = " + response.getVideoMeta().getTitle() + "\n");
} catch (Exception e) {
System.out.print("ErrorMessage = " + e.getLocalizedMessage());
}
System.out.print("RequestId = " + response.getRequestId() + "\n");
}
2、获取视频播放地址
/**
* 获取视频播放地址
* @throws ClientException
*/
@Test
public void testGetPlayInfo() throws ClientException {
//初始化客户端、请求对象和相应对象
DefaultAcsClient client = AliyunVodSDKUtils.initVodClient(accessKeyId, accessKeySecret);
GetPlayInfoRequest request = new GetPlayInfoRequest();
GetPlayInfoResponse response = new GetPlayInfoResponse();
try {
//设置请求参数
//注意:这里只能获取非加密视频的播放地址
request.setVideoId("视频ID");
//获取请求响应
response = client.getAcsResponse(request);
//输出请求结果
List<GetPlayInfoResponse.PlayInfo> playInfoList = response.getPlayInfoList();
//播放地址
for (GetPlayInfoResponse.PlayInfo playInfo : playInfoList) {
System.out.print("PlayInfo.PlayURL = " + playInfo.getPlayURL() + "\n");
}
//Base信息
System.out.print("VideoBase.Title = " + response.getVideoBase().getTitle() + "\n");
} catch (Exception e) {
System.out.print("ErrorMessage = " + e.getLocalizedMessage());
}
System.out.print("RequestId = " + response.getRequestId() + "\n");
}
参考文档:https://help.aliyun.com/document_detail/53406.html
三、文件上传测试 - 安装SDK
1、配置pom
<dependency>
<groupId>com.aliyun</groupId>
<artifactId>aliyun-java-sdk-core</artifactId>
<version>4.3.3</version>
</dependency>
<dependency>
<groupId>com.aliyun.oss</groupId>
<artifactId>aliyun-sdk-oss</artifactId>
<version>3.1.0</version>
</dependency>
<dependency>
<groupId>com.aliyun</groupId>
<artifactId>aliyun-java-sdk-vod</artifactId>
<version>2.15.2</version>
</dependency>
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>fastjson</artifactId>
<version>1.2.28</version>
</dependency>
<dependency>
<groupId>org.json</groupId>
<artifactId>json</artifactId>
<version>20170516</version>
</dependency>
<dependency>
<groupId>com.google.code.gson</groupId>
<artifactId>gson</artifactId>
<version>2.8.2</version>
</dependency>
2、安装非开源jar包
在本地Maven仓库中安装jar包:
下载视频上传SDK,解压,命令行进入lib目录,执行以下代码
mvn install:install-file -DgroupId=com.aliyun -DartifactId=aliyun-sdk-vod-upload -Dversion=1.4.11 -Dpackaging=jar -Dfile=aliyun-java-vod-upload-1.4.11.jar
然后在pom中引入jar包
<dependency>
<groupId>com.aliyun</groupId>
<artifactId>aliyun-sdk-vod-upload</artifactId>
<version>1.4.11</version>
</dependency>
四、测试
1、创建测试文件
package com.atguigu.aliyunvod;
public class UploadTest {
//账号AK信息请填写(必选)
private static final String accessKeyId = "你的accessKeyId";
//账号AK信息请填写(必选)
private static final String accessKeySecret = "你的accessKeySecret";
}
2、测试本地文件上传
/**
* 视频上传
*/
@Test
public void testUploadVideo(){
//1.音视频上传-本地文件上传
//视频标题(必选)
String title = "3 - How Does Project Submission Work - upload by sdk";
//本地文件上传和文件流上传时,文件名称为上传文件绝对路径,如:/User/sample/文件名称.mp4 (必选)
//文件名必须包含扩展名
String fileName = "E:/共享/资源/课程视频/3 - How Does Project Submission Work.mp4";
//本地文件上传
UploadVideoRequest request = new UploadVideoRequest(accessKeyId, accessKeySecret, title, fileName);
/* 可指定分片上传时每个分片的大小,默认为1M字节 */
request.setPartSize(1 * 1024 * 1024L);
/* 可指定分片上传时的并发线程数,默认为1,(注:该配置会占用服务器CPU资源,需根据服务器情况指定)*/
request.setTaskNum(1);
/* 是否开启断点续传, 默认断点续传功能关闭。当网络不稳定或者程序崩溃时,再次发起相同上传请求,可以继续未完成的上传任务,适用于超时3000秒仍不能上传完成的大文件。
注意: 断点续传开启后,会在上传过程中将上传位置写入本地磁盘文件,影响文件上传速度,请您根据实际情况选择是否开启*/
request.setEnableCheckpoint(false);
UploadVideoImpl uploader = new UploadVideoImpl();
UploadVideoResponse response = uploader.uploadVideo(request);
System.out.print("RequestId=" + response.getRequestId() + "\n"); //请求视频点播服务的请求ID
if (response.isSuccess()) {
System.out.print("VideoId=" + response.getVideoId() + "\n");
} else {
/* 如果设置回调URL无效,不影响视频上传,可以返回VideoId同时会返回错误码。其他情况上传失败时,VideoId为空,此时需要根据返回错误码分析具体错误原因 */
System.out.print("VideoId=" + response.getVideoId() + "\n");
System.out.print("ErrorCode=" + response.getCode() + "\n");
System.out.print("ErrorMessage=" + response.getMessage() + "\n");
}
}