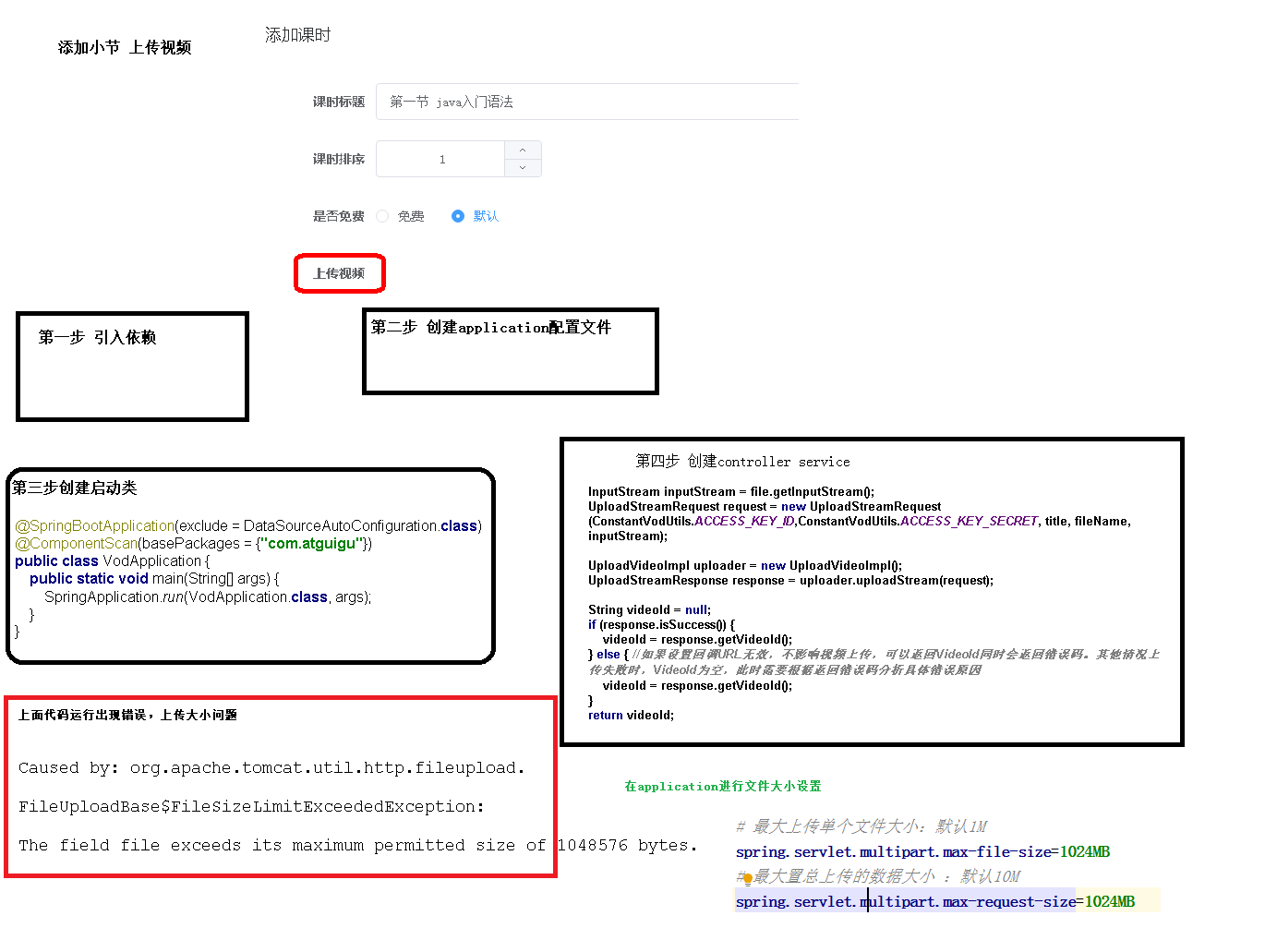
一、创建视频点播微服务
1、创建微服务模块
2、pom
(1)service-vod中引入依赖
<dependencies>
<dependency>
<groupId>com.aliyun</groupId>
<artifactId>aliyun-java-sdk-core</artifactId>
</dependency>
<dependency>
<groupId>com.aliyun.oss</groupId>
<artifactId>aliyun-sdk-oss</artifactId>
</dependency>
<dependency>
<groupId>com.aliyun</groupId>
<artifactId>aliyun-java-sdk-vod</artifactId>
</dependency>
<dependency>
<groupId>com.aliyun</groupId>
<artifactId>aliyun-sdk-vod-upload</artifactId>
</dependency>
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>fastjson</artifactId>
</dependency>
<dependency>
<groupId>org.json</groupId>
<artifactId>json</artifactId>
</dependency>
<dependency>
<groupId>com.google.code.gson</groupId>
<artifactId>gson</artifactId>
</dependency>
<dependency>
<groupId>joda-time</groupId>
<artifactId>joda-time</artifactId>
</dependency>
</dependencies>
3、application.properties
# 服务端口
server.port=8003
# 服务名
spring.application.name=service-vod
# 环境设置:dev、test、prod
spring.profiles.active=dev
#阿里云 vod
#不同的服务器,地址不同
aliyun.vod.file.keyid=your accessKeyId
aliyun.vod.file.keysecret=your accessKeySecret
# 最大上传单个文件大小:默认1M
spring.servlet.multipart.max-file-size=1024MB
# 最大置总上传的数据大小 :默认10M
spring.servlet.multipart.max-request-size=1024MB
4、logback.xml
5、启动类
VodApplication.java
package com.guli.vod;
@SpringBootApplication(exclude = DataSourceAutoConfiguration.class)
@ComponentScan(basePackages={"com.atguigu"})
public class VodApplication {
public static void main(String[] args) {
SpringApplication.run(VodApplication.class, args);
}
}
二、整合阿里云vod实现视频上传
1、创建常量类
ConstantPropertiesUtil.java
package com.guli.vod.util;
@Component
//@PropertySource("classpath:application.properties")
public class ConstantPropertiesUtil implements InitializingBean {
@Value("${aliyun.vod.file.keyid}")
private String keyId;
@Value("${aliyun.vod.file.keysecret}")
private String keySecret;
public static String ACCESS_KEY_ID;
public static String ACCESS_KEY_SECRET;
@Override
public void afterPropertiesSet() throws Exception {
ACCESS_KEY_ID = keyId;
ACCESS_KEY_SECRET = keySecret;
}
}
2、复制工具类到项目中
AliyunVodSDKUtils.java
package com.guli.vod.util;
public class AliyunVodSDKUtils {
public static DefaultAcsClient initVodClient(String accessKeyId, String accessKeySecret) throws ClientException {
String regionId = "cn-shanghai"; // 点播服务接入区域
DefaultProfile profile = DefaultProfile.getProfile(regionId, accessKeyId, accessKeySecret);
DefaultAcsClient client = new DefaultAcsClient(profile);
return client;
}
}
3、配置swagger
4、创建service
接口:VideoService.java
package com.guli.vod.service;
public interface VideoService {
String uploadVideo(MultipartFile file);
}
实现:VideoServiceImpl.java
package com.guli.vod.service.impl;
@Service
public class VideoServiceImpl implements VideoService {
@Override
public String uploadVideo(MultipartFile file) {
try {
InputStream inputStream = file.getInputStream();
String originalFilename = file.getOriginalFilename();
String title = originalFilename.substring(0, originalFilename.lastIndexOf("."));
UploadStreamRequest request = new UploadStreamRequest(
ConstantPropertiesUtil.ACCESS_KEY_ID,
ConstantPropertiesUtil.ACCESS_KEY_SECRET,
title, originalFilename, inputStream);
UploadVideoImpl uploader = new UploadVideoImpl();
UploadStreamResponse response = uploader.uploadStream(request);
//如果设置回调URL无效,不影响视频上传,可以返回VideoId同时会返回错误码。
// 其他情况上传失败时,VideoId为空,此时需要根据返回错误码分析具体错误原因
String videoId = response.getVideoId();
if (!response.isSuccess()) {
String errorMessage = "阿里云上传错误:" + "code:" + response.getCode() + ", message:" + response.getMessage();
log.warn(errorMessage);
if(StringUtils.isEmpty(videoId)){
throw new GuliException(20001, errorMessage);
}
}
return videoId;
} catch (IOException e) {
throw new GuliException(20001, "guli vod 服务上传失败");
}
}
}
5、创建controller
VideoAdminController.java
package com.guli.vod.service.impl;
@Service
public class VideoServiceImpl implements VideoService {
@Override
public String uploadVideo(MultipartFile file) {
try {
InputStream inputStream = file.getInputStream();
String originalFilename = file.getOriginalFilename();
String title = originalFilename.substring(0, originalFilename.lastIndexOf("."));
UploadStreamRequest request = new UploadStreamRequest(
ConstantPropertiesUtil.ACCESS_KEY_ID,
ConstantPropertiesUtil.ACCESS_KEY_SECRET,
title, originalFilename, inputStream);
UploadVideoImpl uploader = new UploadVideoImpl();
UploadStreamResponse response = uploader.uploadStream(request);
//如果设置回调URL无效,不影响视频上传,可以返回VideoId同时会返回错误码。
// 其他情况上传失败时,VideoId为空,此时需要根据返回错误码分析具体错误原因
String videoId = response.getVideoId();
if (!response.isSuccess()) {
String errorMessage = "阿里云上传错误:" + "code:" + response.getCode() + ", message:" + response.getMessage();
log.warn(errorMessage);
if(StringUtils.isEmpty(videoId)){
throw new GuliException(20001, errorMessage);
}
}
return videoId;
} catch (IOException e) {
throw new GuliException(20001, "guli vod 服务上传失败");
}
}
}
6、启动后端vod微服务
7、swagger测试
三、删除云端视频后端实现
文档:服务端SDK->Java SDK->媒资管理
https://help.aliyun.com/document_detail/61065.html?spm=a2c4g.11186623.6.831.654b3815cIxvma#h2—div-id-deletevideo-div-7
1、service
接口
void removeVideo(String videoId);
实现
@Override
public void removeVideo(String videoId) {
try{
DefaultAcsClient client = AliyunVodSDKUtils.initVodClient(
ConstantPropertiesUtil.ACCESS_KEY_ID,
ConstantPropertiesUtil.ACCESS_KEY_SECRET);
DeleteVideoRequest request = new DeleteVideoRequest();
request.setVideoIds(videoId);
DeleteVideoResponse response = client.getAcsResponse(request);
System.out.print("RequestId = " + response.getRequestId() + "\n");
}catch (ClientException e){
throw new GuliException(20001, "视频删除失败");
}
}
2、controller
@DeleteMapping("{videoId}")
public R removeVideo(@ApiParam(name = "videoId", value = "云端视频id", required = true)
@PathVariable String videoId){
videoService.removeVideo(videoId);
return R.ok().message("视频删除成功");
}
二、前端
1、定义api
api/edu/vod.js
import request from '@/utils/request'
const api_name = '/admin/vod/video'
export default {
removeById(id) {
return request({
url: `${api_name}/${id}`,
method: 'delete'
})
}
}