JavaFX有其他内置的形状,如:
- Arc
- Circle
- CubicCurve
- Ellipse
- Line
- Path
- Polygon
- Polyline
- QuadCurve
- Rectangle
- SVGPath
Text
以下代码显示了如何创建路径(Path)。
```java import javafx.application.Application; import javafx.geometry.Insets; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.effect.DropShadow; import javafx.scene.layout.HBox; import javafx.scene.layout.VBox; import javafx.scene.paint.Color; import javafx.scene.shape.Ellipse; import javafx.scene.shape.LineTo; import javafx.scene.shape.MoveTo; import javafx.scene.shape.Path; import javafx.stage.Stage;
public class Main extends Application {
@Override
public void start(Stage stage) throws Exception {
Group group = new Group();
Scene scene = new Scene(group);
stage.setTitle("Checkbox Sample");
stage.setWidth(230);
stage.setHeight(120);
Path path = new Path();
path.getElements().add(new MoveTo(0.0f, 50.0f));
path.getElements().add(new LineTo(100.0f, 100.0f));
VBox vbox = new VBox();
vbox.getChildren().addAll(path);
vbox.setSpacing(5);
HBox root = new HBox();
root.getChildren().add(vbox);
root.setSpacing(40);
root.setPadding(new Insets(20, 10, 10, 20));
group.getChildren().add(root);
stage.setScene(scene);
stage.show();
}
public static void main(String[] args) {
launch(args);
}
}
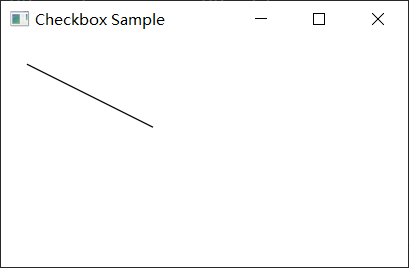<br />Path元素实际上从javafx.scene.shape.PathElement类扩展,它仅在Path对象的上下文中使用。<br />所以不能实例化一个LineTo类放在场景图中。使用To作为后缀的类是Path元素,而不是Shape节点。<br />例如,MoveTo和LineTo对象实例可添加到Path对象的Path元素中,而不可以添加到场景的形状。
<a name="IehEI"></a>
## 示例-1
以下代码显示了如何将QuadCurveTo添加到路径。
```java
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.shape.MoveTo;
import javafx.scene.shape.Path;
import javafx.scene.shape.QuadCurveTo;
import javafx.stage.Stage;
public class Main extends Application {
@Override
public void start(Stage stage) {
Group root = new Group();
Scene scene = new Scene(root, 300, 150);
stage.setScene(scene);
stage.setTitle("曲线");
Path path = new Path();
MoveTo moveTo = new MoveTo();
moveTo.setX(0.0f);
moveTo.setY(50.0f);
QuadCurveTo quadTo = new QuadCurveTo();
quadTo.setControlX(25.0f);
quadTo.setControlY(0.0f);
quadTo.setX(50.0f);
quadTo.setY(50.0f);
path.getElements().add(moveTo);
path.getElements().add(quadTo);
root.getChildren().add(path);
scene.setRoot(root);
stage.show();
}
public static void main(String[] args) {
launch(args);
}
}
实例-2
使用Path,MoveTo和CubicCurveTo创建曲线 -
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.shape.CubicCurveTo;
import javafx.scene.shape.MoveTo;
import javafx.scene.shape.Path;
import javafx.stage.Stage;
public class Main extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage stage) {
stage.setTitle("ComboBoxSample");
Scene scene = new Scene(new Group(), 450, 250);
Path path = new Path();
MoveTo moveTo = new MoveTo();
moveTo.setX(0.0f);
moveTo.setY(0.0f);
CubicCurveTo cubicTo = new CubicCurveTo();
cubicTo.setControlX1(0.0f);
cubicTo.setControlY1(0.0f);
cubicTo.setControlX2(100.0f);
cubicTo.setControlY2(100.0f);
cubicTo.setX(100.0f);
cubicTo.setY(50.0f);
path.getElements().add(moveTo);
path.getElements().add(cubicTo);
Group root = (Group) scene.getRoot();
root.getChildren().add(path);
stage.setScene(scene);
stage.show();
}
}
实例-3
减去两个形状以创建路径,如下代码 -
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.paint.Color;
import javafx.scene.shape.*;
import javafx.stage.Stage;
public class Main extends Application {
@Override
public void start(Stage stage) throws Exception {
stage.setTitle("Shapes");
Group root = new Group();
Scene scene = new Scene(root, 300, 300, Color.WHITE);
Ellipse bigCircle = new Ellipse(100,100,50,75/2);
bigCircle.setStrokeWidth(3);
bigCircle.setStroke(Color.BLACK);
bigCircle.setFill(Color.WHITE);
Ellipse smallCircle = new Ellipse(100,100,35/2,25/2);
Shape shape = Path.subtract(bigCircle, smallCircle);
shape.setStrokeWidth(1);
shape.setStroke(Color.BLACK);
shape.setFill(Color.rgb(255, 200, 0));
root.getChildren().add(shape);
stage.setScene(scene);
stage.show();
}
public static void main(String[] args) {
launch(args);
}
}
实例-4
使用VLineTo创建垂直线,如下代码所示 -
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.layout.VBox;
import javafx.scene.shape.MoveTo;
import javafx.scene.shape.Path;
import javafx.scene.shape.VLineTo;
import javafx.stage.Stage;
public class Main extends Application {
@Override
public void start(final Stage stage) {
stage.setTitle("HTML");
stage.setWidth(500);
stage.setHeight(500);
Scene scene = new Scene(new Group());
VBox root = new VBox();
Path path = new Path();
path.getElements().add(new MoveTo(50.0f, 0.0f));
path.getElements().add(new VLineTo(50.0f));
root.getChildren().addAll(path);
scene.setRoot(root);
stage.setScene(scene);
stage.show();
}
public static void main(String[] args) {
launch(args);
}
}