在 Flutter 中的组件样式,都是通过组件上的 style 属性进行设置的,这与 React Native 很类似。
例如,在 Text 组件里设置样式。
new Text('Hello',
style: new TextStyle(
fontSize: 24.0,
fontWeight: FontWeight.w900,
fontFamily: "Georgia",
)
);
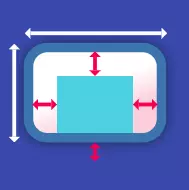
与 React Native 不同的是,有一些样式不不能在 style 里面设置的。例如 width,height,color 等属性。因为 Flutter 认为这样应该是组件的属性而不是样式。
new Text(
'Hello',
style: new TextStyle(
fontSize: 24.0,
fontWeight: FontWeight.w900,
fontFamily: "Georgia",
),
textAlign: TextAlign.center,
)
容器大小
var container = new Container(
width: 320.0,
height: 240.0,
);
容器边距
边距只要是 padding(内边距) 和 margin(外边距)两个设置。边距只适用于 Container。
new Container(
padding: new EdgeInsets.all(20.0),
// padding: 20px;
padding: new EdgeInsets.only(left: 30.0, right: 0.0, top: 20.0, bottom: 20.0),
// padding-left: 30px;
// padding-right: 0;
// padding-top: 20px;
// padding-bottom: 20px;
padding: new EdgeInsets.symmetric(vertical: 10.0, horizontal: 20.0),
// padding: 10px 20px;
// 同理,对于 margin 也是一样
margin: new EdgeInsets.all(20.0),
)
位置信息
如果要使用绝对定位,那么需要把内容包裹在 Positioned 容器里,而 Positioned 又需要包裹在 Stack 容器里。
var container = new Container(
child: new Stack(
children: [
new Positioned(
child: new Container(
child: new Text("Lorem ipsum"),
),
left: 24.0,
top: 24.0,
),
],
),
width: 320.0,
height: 240.0,
);
容器边框
容器的边框设置,使用 Border 对象。边框只适用于 Container。
decoration: new BoxDecoration(
color: Colors.red[400],
// 这里设置
border: new Border.all(width: 2.0, style: BorderStyle.solid),
),
容器圆角
要设置容器的圆角,使用 BorderRadius 对象,它只能使用于 Container。
new Container(
decoration: new BoxDecoration(
color: Colors.red[400],
// 这里设置
borderRadius: new BorderRadius.all(
const Radius.circular(8.0),
),
),
padding: new EdgeInsets.all(16.0),
),
BorderRadius
有以下的属性与方法。
- BorderRadius.all() - 创建所有半径的边界半径 radius。
- BorderRadius.circular() - 同时设置四个圆角。
- BorderRadius.horizontal() - 在水平方向上设置圆角。
- BorderRadius.only() - 只设置某个角。
- BorderRadius.vertical() - 在垂直方向上设置圆角。
borderRadius: new BorderRadius.circular(5.0));阴影效果
在 Flutter 里设置阴影效果,需要使用 BoxShadow 对象。阴影效果只适用于 Container。
等效于 css 上的阴影效果设置。decoration: new BoxDecoration(
color: Colors.red,
boxShadow: <BoxShadow>[
new BoxShadow (
offset: new Offset(0.0, 2.0), // (x, y)
blurRadius: 4.0,
color: const Color(0xcc000000),
),
new BoxShadow (
offset: new Offset(0.0, 6.0),
blurRadius: 20.0,
color: const Color(0x80000000),
),
],
),
box-shadow: 0 2px 4px rgba(0, 0, 0, 0.8),
0 6px 20px rgba(0, 0, 0, 0.5);