import 'dart:convert';
import 'package:flutter/material.dart';
import 'package:dio/dio.dart';
import 'dart:async';
import 'package:fluttertoast/fluttertoast.dart';
import './main.dart';
class LoginPage extends StatefulWidget {
_LoginPageState createState() => _LoginPageState();
}
class _LoginPageState extends State<LoginPage> {
TextStyle TheStyle = TextStyle(
color: Colors.white, fontSize: 17.0, fontWeight: FontWeight.w500);
var usernameController = new TextEditingController();
var userPwdController = new TextEditingController();
@override
Widget build(BuildContext context) {
return Scaffold(
// appBar: AppBar(
// title: Text('芝麻开门'),
// centerTitle: true,
// ),
backgroundColor: Color.fromRGBO(150, 180, 230, 3),
body: Center(
child: new Column(
//MainAxisSize在主轴方向占有空间的值,默认是max。还有一个min
mainAxisSize: MainAxisSize.max,
//MainAxisAlignment:主轴方向上的对齐方式,会对child的位置起作用,默认是start。
mainAxisAlignment: MainAxisAlignment.start,
children: <Widget>[
Container(
height: 25,),
Container(
width: 410,
height: 120,
padding: new EdgeInsets.fromLTRB(10, 0.0, 10.0, 10.0),
child: Container(
child: Image.network(
'https://cdn.nlark.com/yuque/0/2019/png/164272/1556429404436-33f4dea0-2d03-4d5a-b991-fa28eca50ffb.png'),
color: Color.fromRGBO(150, 180, 230, 1),
)),
Padding(
padding: EdgeInsets.all(10.0),
// 用户名输入框
child: TextField(
// 控制器
controller: usernameController,
keyboardType: TextInputType.number,
// maxLength: 11,
// maxLines: 1,
// 是否自动更正
autocorrect: false,
// 是否自动对焦
autofocus: false,
decoration: new InputDecoration(
suffixText: "可以用学号",
suffixStyle: TextStyle(fontSize: 12.0, fontWeight: FontWeight.w300,color: Colors.blueGrey[300]),
labelText: "用户名",
// labelStyle: TextStyle(fontSize: 17.0, fontWeight: FontWeight.w600,color: Colors.blueGrey[300]),
icon: new Icon(Icons.filter_tilt_shift,color: Colors.lightBlueAccent[300],),
),
onChanged: (text) {
//内容改变的回调
print('change $text');
},
onSubmitted: (text) {
//内容提交(按回车)的回调
print('submit $text');
},
),
),
Padding(
padding: EdgeInsets.all(10.0),
child: TextField(
//控制器
controller: userPwdController,
// 密码
obscureText: true,
decoration: new InputDecoration(
labelText: "密码",
icon: new Icon(Icons.lock_outline,color: Colors.lightBlueAccent[300],),
),
onChanged: (text) {
//内容改变的回调
print('change $text');
},
onSubmitted: (text) {
//内容提交(按回车)的回调
print('submit $text');
},
),
),
Container(
//这里写800已经超出屏幕了,可以理解为match_parent
width: 800.0,
margin: new EdgeInsets.fromLTRB(10.0, 10.0, 10.0, 10.0),
padding: new EdgeInsets.fromLTRB(10.0, 10.0, 10.0, 10.0),
//类似cardview
child: new Card(
color: Colors.blueAccent,
// 阴影
elevation: 3.0,
//按钮
child: new FlatButton(
disabledColor: Colors.grey,
disabledTextColor: Colors.black,
onPressed: () {
if (usernameController.text.isEmpty) {
//第三方的插件Toast,https://pub.dartlang.org/packages/fluttertoast
Fluttertoast.showToast(
msg: "用户名不能为空哦",
toastLength: Toast.LENGTH_SHORT,
gravity: ToastGravity.BOTTOM,
timeInSecForIos: 2,
backgroundColor: Colors.white70,
textColor: Colors.grey[800]);
} else if (userPwdController.text.isEmpty) {
Fluttertoast.showToast(
msg: "密码不能为空哦",
toastLength: Toast.LENGTH_SHORT,
gravity: ToastGravity.BOTTOM,
timeInSecForIos: 1,
backgroundColor: Colors.white70,
fontSize: 16.0,
textColor: Colors.black);
} else {
//弹出对话框,里面写着账号和密码
showDialog(
context: context,
builder: (_) {
return AlertDialog(
title: Text("对话框"),
content: Text(usernameController.text +
"\n" +
userPwdController.text),
actions: <Widget>[
//对话框里面的两个按钮
FlatButton(
onPressed: () {
Navigator.of(context).pop();
},
child: Text("取消")),
FlatButton(
//点击确定跳转到下一个界面,也就是HomePage
onPressed: () {
Navigator.push(
context,
MaterialPageRoute(
builder: (context) =>
new MyHomePage()));
},
child: Text("确定")),
],
);
});
}
},
child: new Padding(
padding: new EdgeInsets.all(10.0),
child: new Text(
'登录',
style: new TextStyle(
color: Colors.white, fontSize: 16.0),
),
)),
),
)
])));
}
}
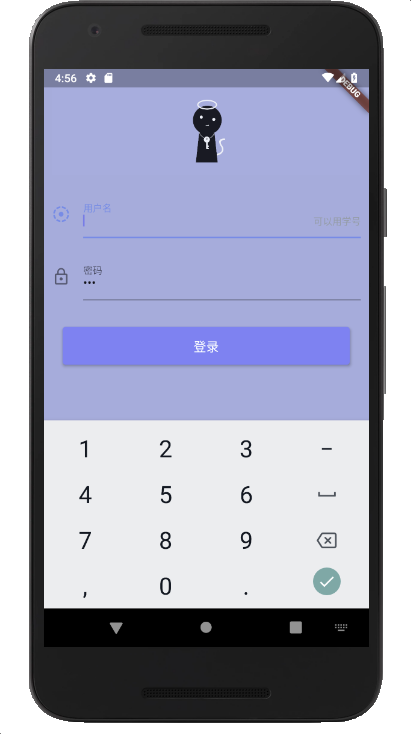