ESLint
供一个插件化的
**javascript**
代码检测工具 ,做 代码格式检测使用的
打开项目中的 .eslintrc.js
文件
// ESLint 配置文件遵循 commonJS 的导出规则,所导出的对象就是 ESLint 的配置对象
// 文档:https://eslint.bootcss.com/docs/user-guide/configuring
module.exports = {
// 表示当前目录即为根目录,ESLint 规则将被限制到该目录下
root: true,
// env 表示启用 ESLint 检测的环境
env: {
// 在 node 环境下启动 ESLint 检测
node: true
},
// ESLint 中基础配置需要继承的配置
extends: ["plugin:vue/vue3-essential", "@vue/standard"],
// 解析器
parserOptions: {
parser: "babel-eslint"
},
// 需要修改的启用规则及其各自的错误级别
/**
* 错误级别分为三种:
* "off" 或 0 - 关闭规则
* "warn" 或 1 - 开启规则,使用警告级别的错误:warn (不会导致程序退出)
* "error" 或 2 - 开启规则,使用错误级别的错误:error (当被触发的时候,程序会退出)
*/
rules: {
"no-console": process.env.NODE_ENV === "production" ? "warn" : "off",
"no-debugger": process.env.NODE_ENV === "production" ? "warn" : "off"
}
};
解决 ESLint 错误,通常情况下我们有两种方式:
- 按照
ESLint
的要求修改代码 - 修改
ESLint
的验证规则
修改 **ESLint**
的验证规则:
- 在
.eslintrc.js
文件中,新增一条验证规则
"quotes": "error" // 默认
"quotes": "warn" // 修改为警告
"quotes": "off" // 修改不校验
Prettier
- 一个代码格式化工具
- 开箱即用
- 可以直接集成到
VSCode
之中 在保存时,让代码直接符合
ESLint
标准(需要通过一些简单配置)ESLint 与 Prettier 配合解决代码格式问题
在
VSCode
中安装prettier
插件(搜索prettier
),这个插件可以帮助我们在配置prettier
的时候获得提示- 在项目中新建
.prettierrc
文件,该文件为perttier
默认配置文件 - 在该文件中写入如下配置:
{
// 不尾随分号
"semi": false,
// 使用单引号
"singleQuote": true,
// 多行逗号分割的语法中,最后一行不加逗号
"trailingComma": "none"
}
- 打开
VSCode
《设置面板》 - 在设置中,搜索
save
,勾选Format On Save
针对于 ESLint 和 prettier 之间的冲突问题:
- 打开
.eslintrc.js
配置文件 - 在
rules
规则下,新增一条规则 - 重启项目
约定式提交规范
对于**git**
提交规范 来说,不同的团队可能会有不同的标准,以目前使用较多的 Angular团队规范 延伸出的 Conventional Commits specification(约定式提交) 为例
约定式提交规范要求如下: ```javascript[optional scope]:
[optional body]
[optional footer(s)]
———— 翻译 ——————-
<类型>[可选 范围]: <描述>
[可选 正文]
[可选 脚注]
其中 `<type>` 类型,必须是一个可选的值,比如:
1. 新功能:`feat`
1. 修复:`fix`
1. 文档变更:`docs`
1. ....
也就是说,如果要按照 **约定式提交规范** 来去做的化,那么你的一次提交描述应该式这个样子的:<br />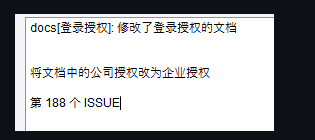
<a name="kjOaw"></a>
# Commitizen助你规范化提交代码
`commitizen` 仓库名为 [cz-cli](https://github.com/commitizen/cz-cli) ,它提供了一个 `git cz` 的指令用于代替 `git commit`,简单一句话介绍它:
> 当你使用 `commitizen` 进行代码提交(git commit)时,`commitizen` 会提交你在提交时填写所有必需的提交字段!
1. 全局安装`Commitizen`
```javascript
npm install -g commitizen@4.2.4
安装并配置
cz-customizable
插件使用
npm
下载cz-customizable
npm i cz-customizable@6.3.0 --save-dev
添加以下配置到
package.json
中...
"config": {
"commitizen": {
"path": "node_modules/cz-customizable"
}
}
项目根目录下创建
.cz-config.js
自定义提示文件module.exports = {
// 可选类型
types: [
{ value: 'feat', name: 'feat: 新功能' },
{ value: 'fix', name: 'fix: 修复' },
{ value: 'docs', name: 'docs: 文档变更' },
{ value: 'style', name: 'style: 代码格式(不影响代码运行的变动)' },
{
value: 'refactor',
name: 'refactor: 重构(既不是增加feature,也不是修复bug)'
},
{ value: 'perf', name: 'perf: 性能优化' },
{ value: 'test', name: 'test: 增加测试' },
{ value: 'chore', name: 'chore: 构建过程或辅助工具的变动' },
{ value: 'revert', name: 'revert: 回退' },
{ value: 'build', name: 'build: 打包' }
],
// 消息步骤
messages: {
type: '请选择提交类型:',
customScope: '请输入修改范围(可选):',
subject: '请简要描述提交(必填):',
body: '请输入详细描述(可选):',
footer: '请输入要关闭的issue(可选):',
confirmCommit: '确认使用以上信息提交?(y/n/e/h)'
},
// 跳过问题
skipQuestions: ['body', 'footer'],
// subject文字长度默认是72
subjectLimit: 72
}
使用
git cz
代替git commit
使用git cz
代替git commit
,即可看到提示内容
那么到这里我们就已经可以使用git cz
来代替了 git commit
实现了规范化的提交诉求了,但是当前依然存在着一个问题,那就是我们必须要通过 git cz
指令才可以完成规范化提交!
Git Hooks
**git**
在执行某个事件之前或之后进行一些其他额外的操作
我们所期望的 阻止不合规的提交消息,那么就需要使用到 hooks
的钩子函数。
Git Hook | 调用时机 | 说明 |
---|---|---|
pre-applypatch | git am 执行前 |
|
applypatch-msg | git am 执行前 |
|
post-applypatch | git am 执行后 |
不影响git am 的结果 |
pre-commit | git commit 执行前 |
可以用git commit --no-verify 绕过 |
commit-msg | git commit 执行前 |
可以用git commit --no-verify 绕过 |
post-commit | git commit 执行后 |
不影响git commit 的结果 |
pre-merge-commit | git merge 执行前 |
可以用git merge --no-verify 绕过。 |
prepare-commit-msg | git commit 执行后,编辑器打开之前 |
|
pre-rebase | git rebase 执行前 |
|
post-checkout | git checkout 或 git switch 执行后 |
如果不使用--no-checkout 参数,则在 git clone 之后也会执行。 |
post-merge | git commit 执行后 |
在执行git pull 时也会被调用 |
pre-push | git push 执行前 |
|
pre-receive | git-receive-pack 执行前 |
|
update | ||
post-receive | git-receive-pack 执行后 |
不影响git-receive-pack 的结果 |
post-update | 当 git-receive-pack 对 git push 作出反应并更新仓库中的引用时 |
|
push-to-checkout | 当`git-receive-pack 对<br />git push 做出反应并更新仓库中的引用时,以及当推送试图更新当前被签出的分支且<br />receive.denyCurrentBranch 配置被设置为<br />updateInstead 时 |
|
pre-auto-gc | git gc --auto 执行前 |
|
post-rewrite | 执行git commit --amend 或 git rebase 时 |
|
sendemail-validate | git send-email 执行前 |
|
fsmonitor-watchman | 配置core.fsmonitor 被设置为 .git/hooks/fsmonitor-watchman 或 .git/hooks/fsmonitor-watchmanv2 时 |
|
p4-pre-submit | git-p4 submit 执行前 |
可以用git-p4 submit --no-verify 绕过 |
p4-prepare-changelist | git-p4 submit 执行后,编辑器启动前 |
可以用git-p4 submit --no-verify 绕过 |
p4-changelist | git-p4 submit 执行并编辑完 changelist message 后 |
可以用git-p4 submit --no-verify 绕过 |
p4-post-changelist | git-p4 submit 执行后 |
|
post-index-change | 索引被写入到read-cache.c do_write_locked_index 后 |
PS:详细的 HOOKS介绍
可点击这里查看
整体的 hooks
非常多,当时我们其中用的比较多的其实只有两个:
Git Hook | 调用时机 | 说明 |
---|---|---|
pre-commit | git commit 执行前 它不接受任何参数,并且在获取提交日志消息并进行提交之前被调用。脚本 git commit 以非零状态退出会导致命令在创建提交之前中止。 |
可以用git commit --no-verify 绕过 |
commit-msg | git commit 执行前 可用于将消息规范化为某种项目标准格式。 还可用于在检查消息文件后拒绝提交。 |
可以用git commit --no-verify 绕过 |
简单来说这两个钩子:
commit-msg
:可以用来规范化标准格式,并且可以按需指定是否要拒绝本次提交pre-commit
:会在提交前被调用,并且可以按需指定是否要拒绝本次提交使用 husky + commitlint 检查提交描述是否符合规范要求
commitlint:用于检查提交信息
- husky:是
git hooks
工具
commitlint
安装依赖:
npm install --save-dev @commitlint/config-conventional@12.1.4 @commitlint/cli@12.1.4
创建
commitlint.config.js
文件echo "module.exports = {extends: ['@commitlint/config-conventional']}" > commitlint.config.js
打开
commitlint.config.js
, 增加配置项( config-conventional 默认配置点击可查看 ):module.exports = {
// 继承的规则
extends: ['@commitlint/config-conventional'],
// 定义规则类型
rules: {
// type 类型定义,表示 git 提交的 type 必须在以下类型范围内
'type-enum': [
2,
'always',
[
'feat', // 新功能 feature
'fix', // 修复 bug
'docs', // 文档注释
'style', // 代码格式(不影响代码运行的变动)
'refactor', // 重构(既不增加新功能,也不是修复bug)
'perf', // 性能优化
'test', // 增加测试
'chore', // 构建过程或辅助工具的变动
'revert', // 回退
'build' // 打包
]
],
// subject 大小写不做校验
'subject-case': [0]
}
}
注意:确保保存为
**UTF-8**
的编码格式,否则可能会出现错误husky
安装依赖:
npm install husky@7.0.1 --save-dev
启动
hooks
, 生成.husky
文件夹npx husky install
在
package.json
中生成prepare
指令( 需要 npm > 7.0 版本 )npm set-script prepare "husky install"
执行
prepare
指令npm run prepare
执行成功,提示
- 添加
commitlint
的hook
到husky
中,并指令在commit-msg
的hooks
下执行npx --no-install commitlint --edit "$1"
指令
至此, 不符合规范的 commit 将不再可提交: ``` PS F:\xxxxxxxxxxxxxxxxxxxxx\imooc-admin> git commit -m “测试” ⧗ input: 测试 ✖ subject may not be empty [subject-empty] ✖ type may not be empty [type-empty]npx husky add .husky/commit-msg 'npx --no-install commitlint --edit "$1"'
✖ found 2 problems, 0 warnings ⓘ Get help: https://github.com/conventional-changelog/commitlint/#what-is-commitlint
husky - commit-msg hook exited with code 1 (error)
<a name="TMS60"></a>
# 通过 pre-commit 检测提交时代码规范
通过 `**husky**`** 监测 **`**pre-commit**`** 钩子,在该钩子下执行 **`**npx eslint --ext .js,.vue src**` 指令来去进行相关检测:
1. 执行 `npx husky add .husky/pre-commit "npx eslint --ext .js,.vue src"` 添加 `commit` 时的 `hook` (`npx eslint --ext .js,.vue src` 会在执行到该 hook 时运行)
1. 该操作会生成对应文件 `pre-commit`
1. 关闭 `VSCode` 的自动保存操作
1. 修改一处代码,使其不符合 `ESLint` 校验规则
1. 执行 **提交操作** 会发现,抛出一系列的错误,代码无法提交
PS F:\xxxxxxxxxxxxxxxxxxx\imooc-admin> git commit -m ‘test’
F:\xxxxxxxxxxxxxxxx\imooc-admin\src\views\Home.vue 13:9 error Strings must use singlequote quotes
✖ 1 problem (1 error, 0 warnings)
1 error and 0 warnings potentially fixable with the --fix
option.
husky - pre-commit hook exited with code 1 (error)
6. 想要提交代码,必须处理完成所有的错误信息
<a name="g4AnM"></a>
# lint-staged 自动修复格式错误
通过 `pre-commit` 处理了 **检测代码的提交规范问题,当我们进行代码提交时,会检测所有的代码格式规范** 。<br />存在两个问题:
1. 我们只修改了个别的文件,没有必要检测所有的文件代码格式
1. 它只能给我们提示出对应的错误,我们还需要手动的进行代码修改
[lint-staged](https://github.com/okonet/lint-staged) 可以让你当前的代码检查 **只检查本次修改更新的代码,并在出现错误的时候,自动修复并且推送**<br />[lint-staged](https://github.com/okonet/lint-staged) 无需单独安装,我们生成项目时,`vue-cli` 已经帮助我们安装过了,所以我们直接使用就可以了
1. 修改 `package.json` 配置
```javascript
"lint-staged": {
"src/**/*.{js,vue}": [
"eslint --fix",
"git add"
]
}
- 如上配置,每次它只会在你本地
commit
之前,校验你提交的内容是否符合你本地配置的eslint
规则(这个见文档 ESLint ),校验会出现两种结果:- 如果符合规则:则会提交成功。
- 如果不符合规则:它会自动执行
eslint --fix
尝试帮你自动修复,如果修复成功则会帮你把修复好的代码提交,如果失败,则会提示你错误,让你修好这个错误之后才能允许你提交代码。
- 修改
.husky/pre-commit
文件 ```javascript!/bin/sh
. “$(dirname “$0”)/_/husky.sh”
npx lint-staged ```
- 再次执行提交代码
- 发现 暂存区中 不符合
ESlint
的内容,被自动修复