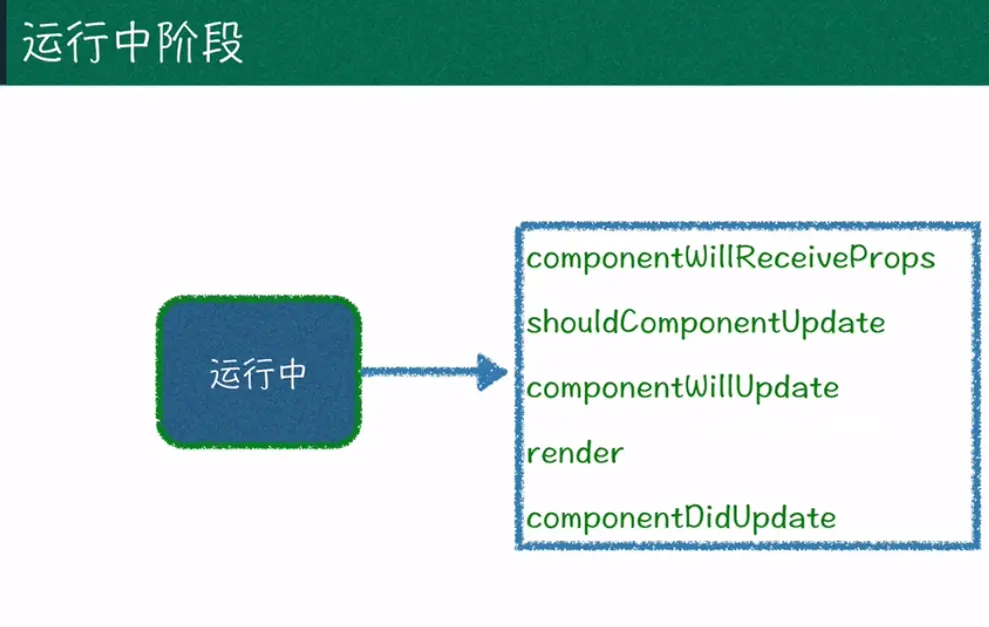

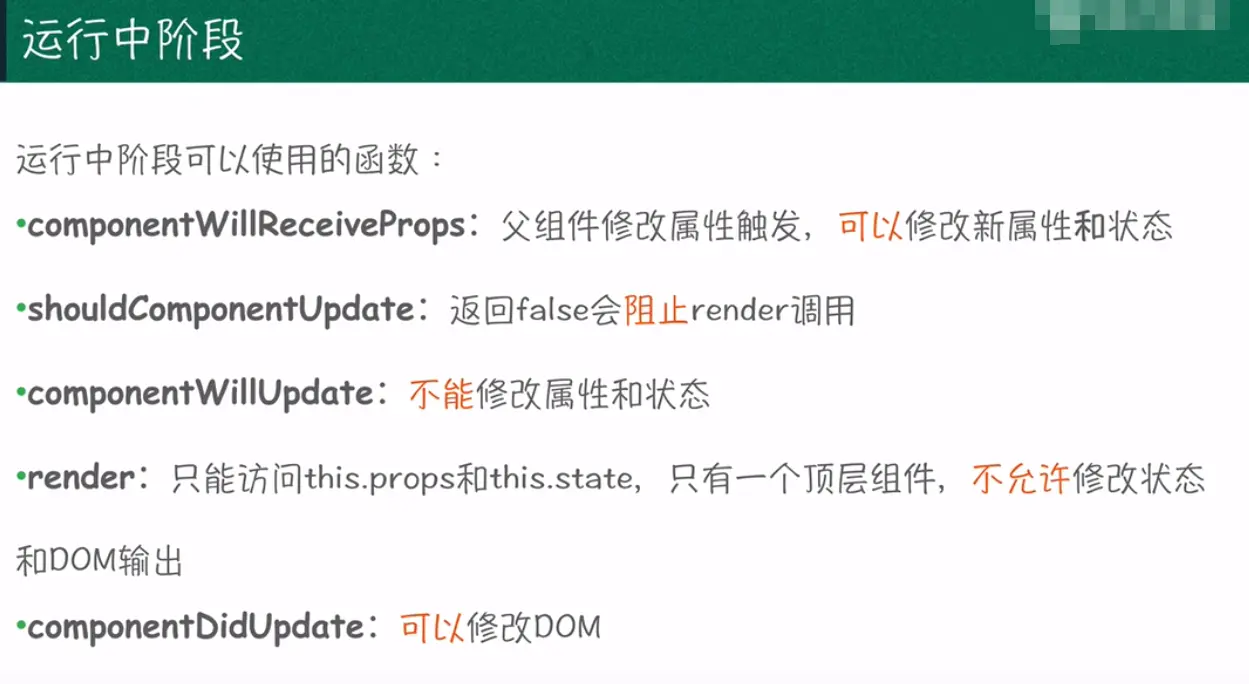
源码
//生命周期脚本
var HelloMassage = React.createClass({
//设置默认属性
getDefaultProps: function() {
console.log("初始化默认的属性");
return {
name: "test"
}
},
//设置默认状态
getInitialState: function() {
console.log("初始化状态成功");
return {
isLoading: false
}
},
//组件被挂载之前,Render 之前最后一次修改状态的机会
componentWillMount: function() {
//修改状态
this.setState({
isLoading: true
});
console.log("组件被挂载之前");
},
//组件被渲染之后
componentDidMount: function() {
console.log("组件被真实的渲染到页面中完成");
// console.log(this.refs);
// console.log(this.refs.uname);
//获取 jquery dom
// console.log($(this.refs.uname));
//获取 value
// console.log($(this.refs.uname).val());
//修改 css
// $(this.refs.uname).css({
// "color": "red"
// });
//自动完成(Autocomplete) 单词补充的示例
// var availableTags = [
// "ActionScript",
// "AppleScript",
// "Asp",
// "BASIC",
// "C",
// "C++",
// "Clojure",
// "COBOL",
// "ColdFusion",
// "Erlang",
// "Fortran",
// "Groovy",
// "Haskell",
// "Java",
// "JavaScript",
// "Lisp",
// "Perl",
// "PHP",
// "Python",
// "Ruby",
// "Scala",
// "Scheme"
// ];
// $(this.refs.uname).autocomplete({
// source: availableTags
// });
//获取 ReactDOM 这个获取到的是一个纯粹的 DOM 节点
console.log(ReactDOM.findDOMNode(this.refs.uname));
},
//不能修改属性和状态
componentWillUpdate: function(data) {
console.log("componentWillUpdate", data);
},
//可以修改 DOM
componentDidUpdate: function(data) {
console.log("componentDidUpdate", data);
},
//返回 false 会阻止 render 调用
shouldComponentUpdate: function(nextrops) {
console.log("shouldComponentUpdate", nextrops);
//返回一个 boolean 值
// return false;//false 的话页面就不会更新
return true;
},
//父组件修改属性触发,可以修改新属性和方法
componentWillReceiveProps: function(nextrops) {
console.log("componentWillReceiveProps", nextrops);
},
_change: function(e) {
// console.log(e);
// console.log(e.target);
console.log(e.target.value);
},
render: function() {
console.log("render 页面");
return (
<div>
{/*这里可以获取到父组件传递过来的 name 属性的值*/}
<label htmlFor="uname">{this.props.name}</label>
{/*<input ref="uname" value={this.props.name} onChange={this._change} type="text"/>*/}
<input id="uname" ref="uname" onChange={this._change} type="text"/>
<h1>{this.props.name}</h1>
{!this.state.isLoading ? <h1>loading...</h1> : <h1>ok</h1>}
</div>
)
}
});
//新建一个父组件
var HelloWorld = React.createClass({
getInitialState: function() {
return {
data: "父容器的状态"
}
},
click: function() {
this.setState({
data: "被改变"
})
},
render: function() {
return (
<form onClick={this.click}>
{/*使用父组件标签包裹着子组件 这样就可以通过父组件来给子组件传递一些属性了
*/}
<HelloMassage name={this.state.data} />
</form>
)
}
});
ReactDOM.render(
<HelloWorld name="我是父组件" />,
document.getElementById("example")
);