剑指 Offer 66. 构建乘积数组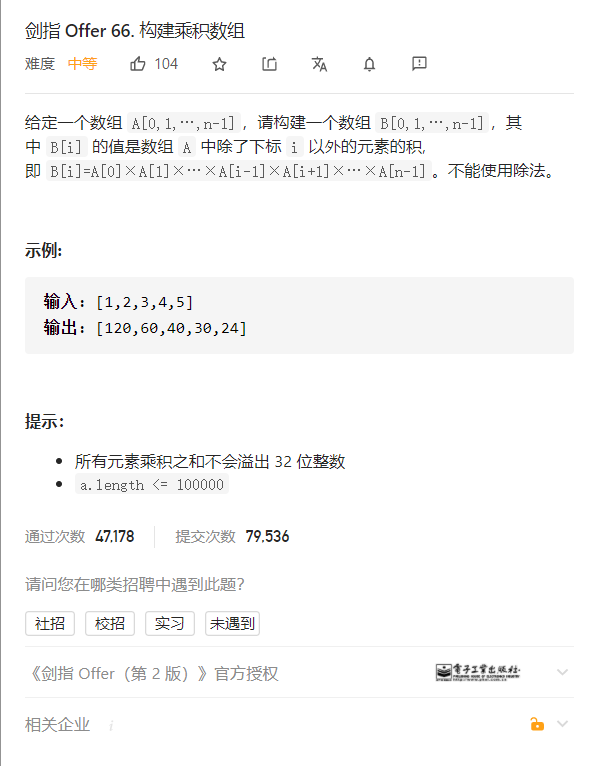
class Solution {
public int[] constructArr(int[] a) {
int len = a.length;
int[] arr = new int[len];
if(len==0)
return arr;
arr[0] = 1;
int t = a[0];
for(int i = 1; i < len; i++) {
arr[i] = t;
t*=a[i];
}
System.out.println(Arrays.toString(arr));
t = a[len-1];
for(int i = len-2;i>=0;i--) {
arr[i] *= t;
t *= a[i];
}
System.out.println(Arrays.toString(arr));
return arr;
}
}
剑指 Offer 68 - I. 二叉搜索树的最近公共祖先
class Solution {
public TreeNode lowestCommonAncestor(TreeNode root, TreeNode p, TreeNode q) {
if(root==null)
return null;
int min = Math.min(p.val,q.val);
int max = Math.max(p.val,q.val);
if(root.val==min || root.val==max)
return root;
if(root.val >min && root.val < max )
return root;
if(root.val > min && root.val > max)
return lowestCommonAncestor(root.left,p,q);
if(root.val<max && root.val<min)
return lowestCommonAncestor(root.right,p,q);
return null;
}
}
剑指 Offer 68 - II. 二叉树的最近公共祖先
/**
* Definition for a binary tree node.
* public class TreeNode {
* int val;
* TreeNode left;
* TreeNode right;
* TreeNode(int x) { val = x; }
* }
*/
class Solution {
public TreeNode lowestCommonAncestor(TreeNode root, TreeNode p, TreeNode q) {
return find(p,q,root);
}
TreeNode find(TreeNode p,TreeNode q,TreeNode root) {
if(root==null)
return null;
TreeNode l = find(p,q,root.left);
TreeNode r = find(p,q,root.right);
if(l!=null && r!=null){
return root;
}
if(root==q || root==p) {
if(l!=null || r!=null)
return root;
return root;
}
if(l!=null)
return l;
if(r!=null)
return r;
return null;
}
}
剑指 Offer 60. n个骰子的点数
dp[i][j] 表示一共i个筛子,掷出j点数的次数
第i个筛子可以是1,2,3,4,5,6 ,所以dp[i][j] = dp[i-1][j-1] + dp[i-1][j-2] + dp[i-1][j-3] + dp[i-1][j-4] + ..dp[i-1][j-6]
class Solution {
public double[] dicesProbability(int n) {
int[][] dp = new int[n+1][6*n+1];
for(int i = 1; i <= 6; i++) {
dp[1][i]=1;
}
for(int i = 2; i <= n ;i++) {
for(int j = i;j<=6*i;j++) {
for(int k = 1; k <= 6 && k<j ;k++) {
dp[i][j] += dp[i-1][j-k];
}
}
}
double count = 0;
for(int i = n;i<=6*n;i++) {
count+=dp[n][i];
}
double[] res = new double[6*n-n+1];
for(int i = 0 ; i < res.length; i++) {
res[i] = dp[n][i+n]/count;
}
return res;
}
}
剑指 Offer 62. 圆圈中最后剩下的数字
class Solution {
public int lastRemaining(int n, int m) {
int f = 0;
for(int i = 2; i <= n; i++) {
f = (m+f)%i;
}
return f;
}
}