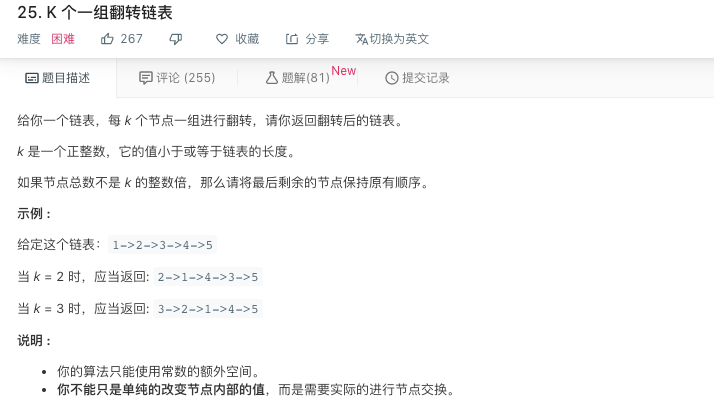
思路
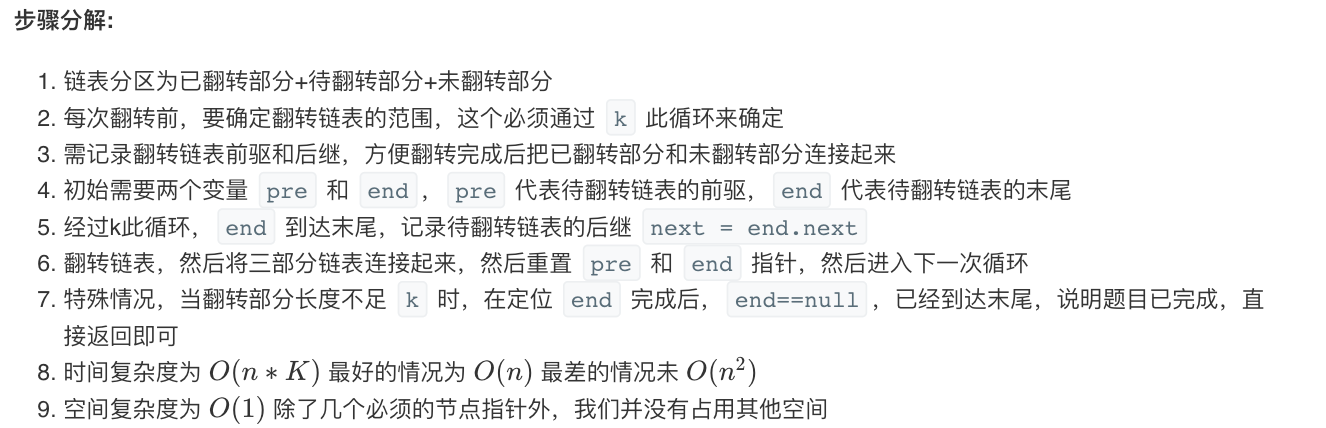
code
public ListNode reverseKGroup(ListNode head, int k) {
//定义虚拟头结点
ListNode dummy = new ListNode(0);
dummy.next= head;
//待反转链表的pre
ListNode pre = dummy;
//待反转链表的end
ListNode end = dummy;
//遍历一遍
while(end.next!=null){
//end迭代k次到达最后
for(int i=0;i<k&&end!=null;i++)
end = end.next;
//如果end到达了最后则跳出循环
if(end ==null)
break;
//待反转链表的start
ListNode start = pre.next;
//还未反转的部分的第一个元素 即为next
ListNode next = end.next;
//将待反转链表断掉
end.next = null;
//将pre的next执行反转之后的部分
pre.next = reverse(start);
//反转之后start为最后一个 重新连接上
start.next = next;
//更新pre 和end
pre = start;
end = start;
}
return dummy.next;
}
///常规的反转链表
public ListNode reverse(ListNode head){
ListNode pre = null;
ListNode curr = head;
while(curr!=null){
ListNode next = curr.next;
curr.next = pre;
pre = curr;
curr = next;
}
return pre;
}