思路
方法 1:额外存储空间方法
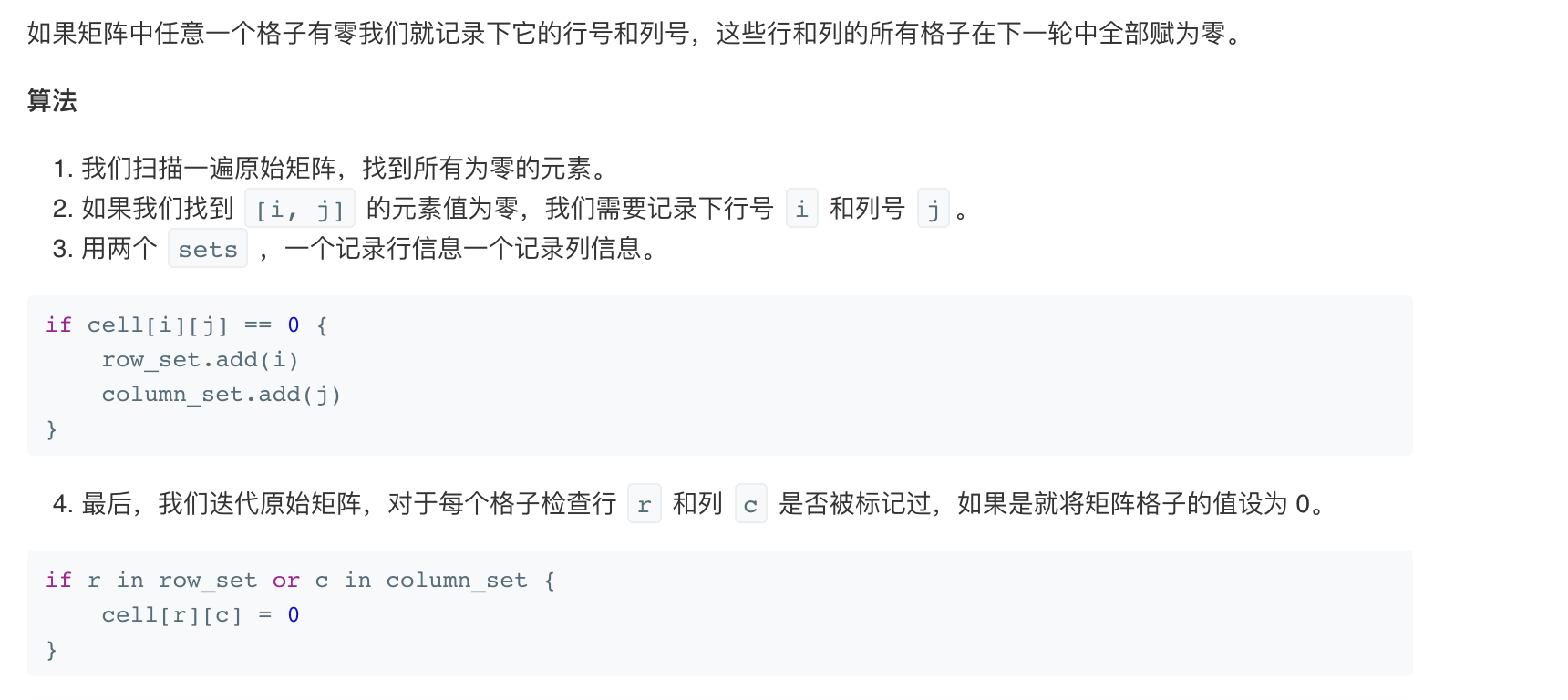
code
public void setZeroes(int[][] matrix) {
Set<Integer> rowSet = new HashSet<>();
Set<Integer> columnSet = new HashSet<>();
int rows = matrix.length;
int columns = matrix[0].length;
for(int i=0;i<rows;i++)
for(int j=0;j<columns;j++){
if(matrix[i][j]==0){
rowSet.add(i); //加到集合中去
columnSet.add(j);
}
}
for(int i=0;i<rows;i++){
for(int j=0;j<columns;j++){
if(rowSet.contains(i)||columnSet.contains(j)){
matrix[i][j]=0; //进行更新
}
}
}
}
方法2 不使用额外空间的解法
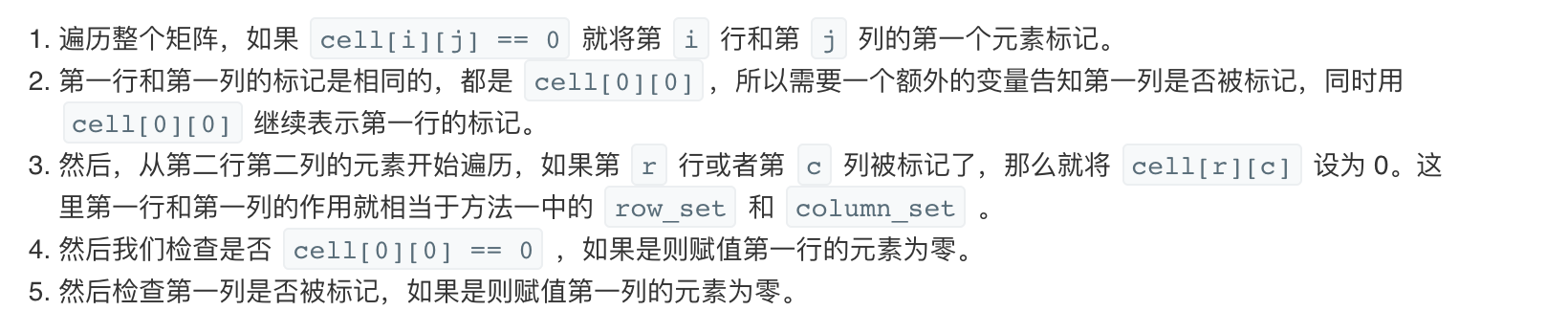
class Solution {
public void setZeroes(int[][] matrix) {
Boolean isCol = false;
int R = matrix.length;
int C = matrix[0].length;
for (int i = 0; i < R; i++) {
// Since first cell for both first row and first column is the same i.e. matrix[0][0]
// We can use an additional variable for either the first row/column.
// For this solution we are using an additional variable for the first column
// and using matrix[0][0] for the first row.
if (matrix[i][0] == 0) {
isCol = true;
}
for (int j = 1; j < C; j++) {
// If an element is zero, we set the first element of the corresponding row and column to 0
if (matrix[i][j] == 0) {
matrix[0][j] = 0;
matrix[i][0] = 0;
}
}
}
for (int i = 1; i < R; i++) {
for (int j = 1; j < C; j++) {
if (matrix[i][0] == 0 || matrix[0][j] == 0) {
matrix[i][j] = 0;
}
}
}
// See if the first row needs to be set to zero as well
if (matrix[0][0] == 0) {
for (int j = 0; j < C; j++) {
matrix[0][j] = 0;
}
}
if (isCol) {
for (int i = 0; i < R; i++) {
matrix[i][0] = 0;
}
}
}
}