思路
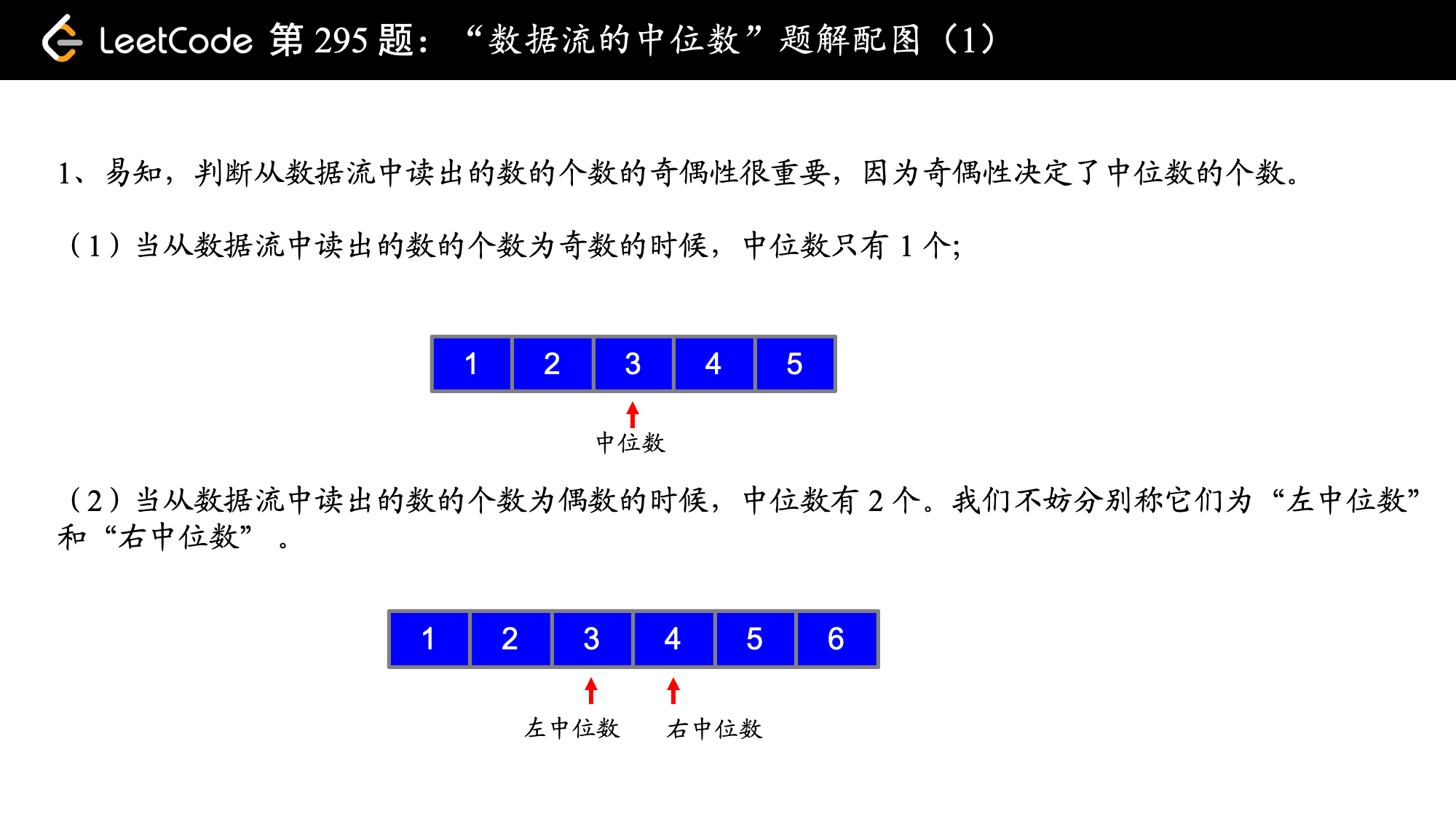
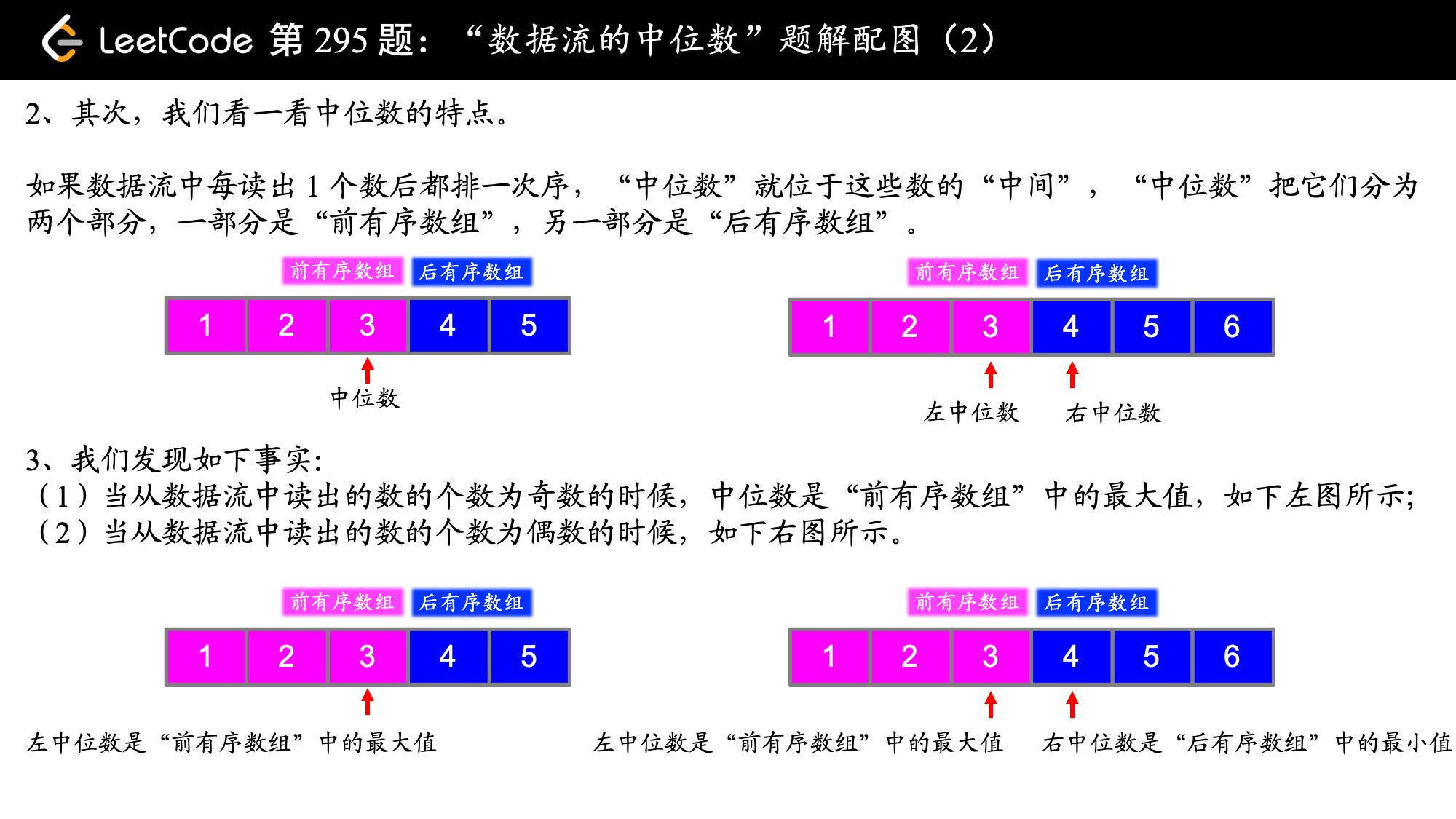
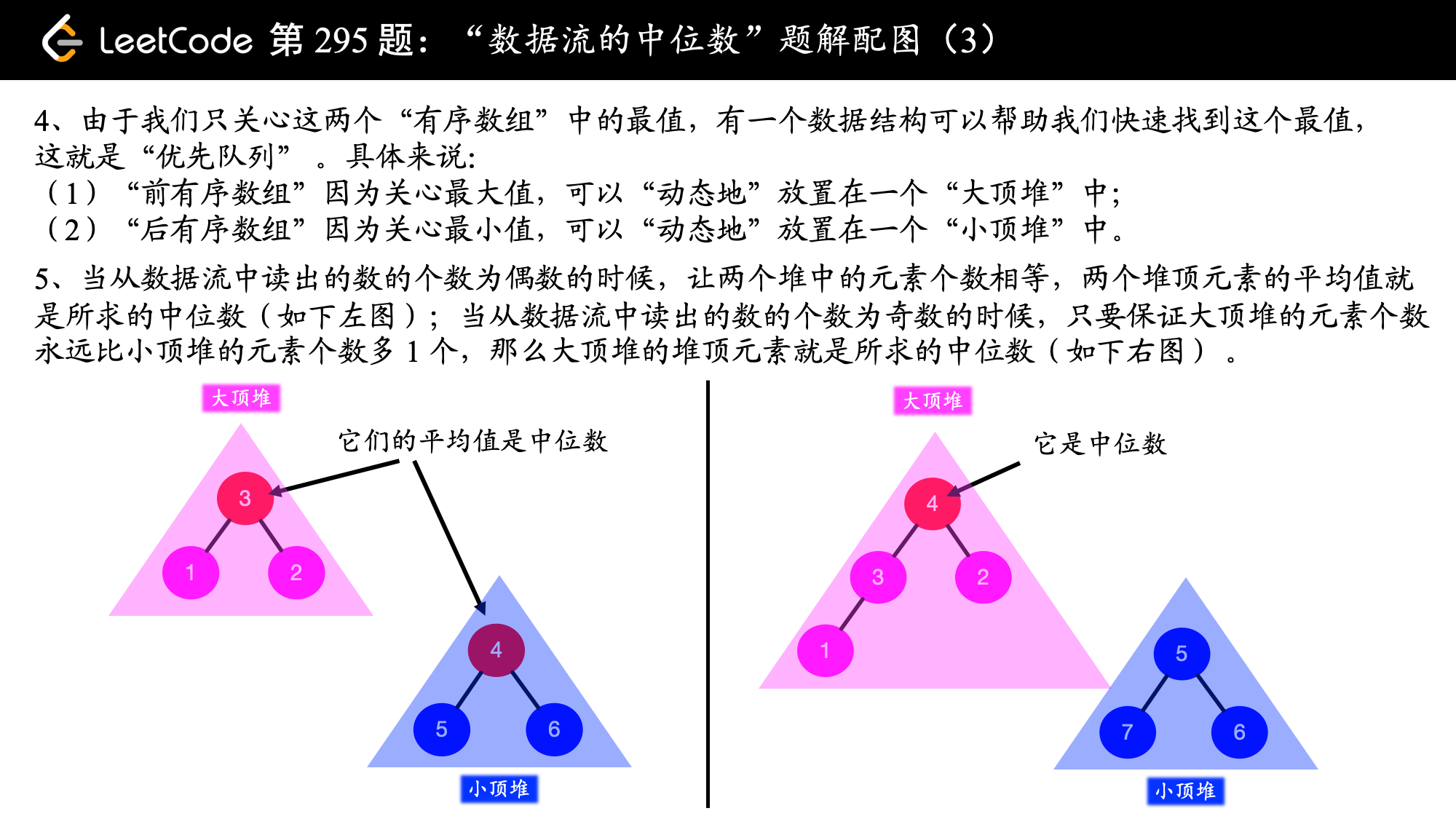
code
import java.util.PriorityQueue;
class MedianFinder {
private int count;
private PriorityQueue<Integer> maxheap;
private PriorityQueue<Integer> minheap;
/** initialize your data structure here. */
public MedianFinder() {
count = 0;
maxheap = new PriorityQueue<>((x,y)->y-x);//使用lambda来声明一个大根堆
minheap = new PriorityQueue<>();
}
public void addNum(int num) {
count++;
maxheap.offer(num); //数据先流入左半部分的大根堆
minheap.add(maxheap.poll()); //大根堆顶部流入右半部分的小根堆
if((count&1)!=0) //如果是偶数 将右半部分的最小元素流入左根堆
maxheap.add(minheap.poll());
}
public double findMedian() {
if(count&1==0)
return (double)maxheap.peek()+minheap.peek()/2;
else
return (double)maxheap.peek();
}
}