思路1 转为数字再拼回去

public ListNode addTwoNumbers(ListNode l1, ListNode l2) {
//拼接l1 l2
String numStr1 = String.valueOf(l1.val);
String numStr2 = String.valueOf(l2.val);
while(l1.next!=null){
numStr1+=String.valueOf(l1.next.val);
l1=l1.next;
}
while(l2.next!=null){
numStr2+=String.valueOf(l2.next.val);
l2=l2.next;
}
//使用大数防止溢出 使用StringBuffer进行反转
BigInteger num1 = new BigInteger(new StringBuffer(numStr1).reverse().toString());
BigInteger num2 = new BigInteger(new StringBuffer(numStr2).reverse().toString());
num1 = num1.add(num2); //这里使用add
//转为string
String numStr = new StringBuffer(String.valueOf(num1)).reverse().toString();
//构建链表
ListNode head = new ListNode(-1);
ListNode dummy = head;
for(int i=0;i<numStr.length();i++){
head.next = new ListNode(Integer.valueOf(numStr.charAt(i)+
""));
head = head.next;
}
return dummy.next;
}
思路2 递归
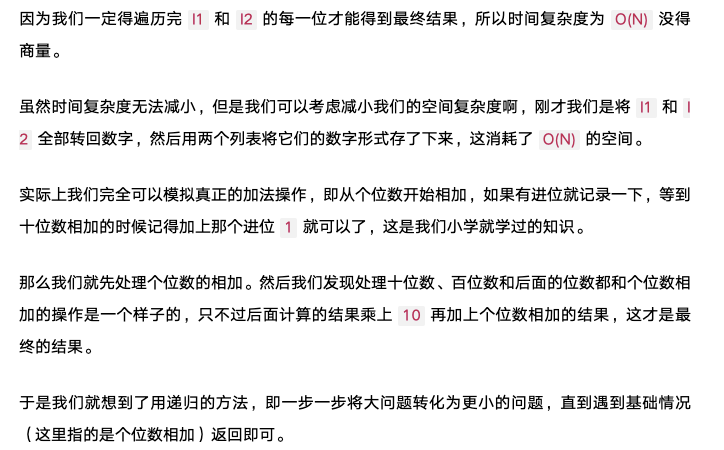
public ListNode addTwoNumbers(ListNode l1, ListNode l2) {
ListNode res = null;
//递归终止条件
if(l1==null&&l2==null)
return null;
if(l1==null&&l2!=null)
return l2;
if(l1!=null&&l2==null)
return l1;
//如果没有产生进位
if(l1.val+l2.val<10){
res = new ListNode(l1.val+l2.val);
res.next = addTwoNumbers(l1.next,l2.next);
}else{ //如果产生了进位 递归求三个数
res = new ListNode(l1.val+l2.val-10);
res.next = addTwoNumbers(l1.next,addTwoNumbers(l2.next,new ListNode(1)));
}
return res;
}