- 封装命令
- 结合cor实现undo
- ctrl+z回退操作
- 是用Command模式来实现的
- 用得挺多的,可能用的没感觉到
解析
- 别名:Action/Transaction
- Command封装了一个命令,比如菜单上的命令
- 命令要执行,必须要有个doit();或者exec();或者run();或者方法(不叫do是因为java中有do关键字)
- 要实现undo功能要有个undo();方法
- 要实现undo功能,do和undo要配着对来
- 如何实现一连串的undo?
- 责任链(无限拓展)===>FilterChain类+Filter接口及其实现类
- Command可以无限拓展,通过继承来扩展,但是容易造成类臃肿(违背单一职责原则……)
- 所有的Command都要放到一个列表List中去
- 动作:菜单的动作
- 事务:不能有中间状态(要么完成要么不完成)
- 宏命令:word===>命令是由好多个一连串的命令组成的大命令
- command与组合composite模式===>树状结构
- 继承、聚合、组成是类与类间的关系,不是设计模式
- 多次undo
- transaction回滚
URL类图
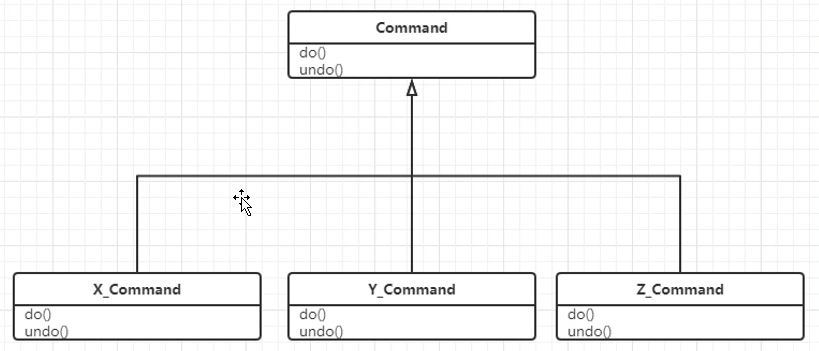
例子:命令回退
Content类
package com.mashibing.dp.command;
public class Content {
String msg = "hello everybody ";
}
Command类
package com.mashibing.dp.command;
public abstract class Command {
public abstract void doit(); //exec run
public abstract void undo();
}
CopyCommand类
package com.mashibing.dp.command;
public class CopyCommand extends Command {
Content c;
public CopyCommand(Content c) {
this.c = c;
}
@Override
public void doit() {
c.msg = c.msg + c.msg;
}
@Override
public void undo() {
c.msg = c.msg.substring(0, c.msg.length()/2);
}
}
DeleteCommand类
package com.mashibing.dp.command;
public class DeleteCommand extends Command {
Content c;
String deleted;
public DeleteCommand(Content c) {
this.c = c;
}
// 删除前5个字符
@Override
public void doit() {
deleted = c.msg.substring(0, 5);
c.msg = c.msg.substring(5, c.msg.length());
}
@Override
public void undo() {
c.msg = deleted + c.msg;
}
}
InsertCommand类
package com.mashibing.dp.command;
public class InsertCommand extends Command {
Content c;
String strToInsert = "http://www.mashibing.com";
public InsertCommand(Content c) {
this.c = c;
}
@Override
public void doit() {
c.msg = c.msg + strToInsert;
}
@Override
public void undo() {
c.msg = c.msg.substring(0, c.msg.length()-strToInsert.length());
}
}
命令回退的使用
package com.mashibing.dp.command;
import java.util.ArrayList;
import java.util.List;
public class Main {
public static void main(String[] args) {
Content c = new Content();
Command insertCommand = new InsertCommand(c);
// 默认插入http://www.mashibing.com
insertCommand.doit();
insertCommand.undo();
Command copyCommand = new CopyCommand(c);
insertCommand.doit();
insertCommand.undo();
Command deleteCommand = new DeleteCommand(c);
deleteCommand.doit();
deleteCommand.undo();
List<Command> commands = new ArrayList<>();
commands.add(new InsertCommand(c));
commands.add(new CopyCommand(c));
commands.add(new DeleteCommand(c));
for(Command comm : commands) {
comm.doit();
}
System.out.println(c.msg);
for(int i= commands.size()-1; i>=0; i--) {
commands.get(i).undo();
}
System.out.println(c.msg);
}
}