数组的定义类型为父类型,里面保存的实际元素类型为子类型。
应用案例:
- 要求创建一个Person对象,2个Student对象和2个Teacher对象,统一放在数组中,并调用每个对象的say方法。
- 如何调用子类特有的方法,比如Teacher有一个teach,Student有一个study,怎么调用?
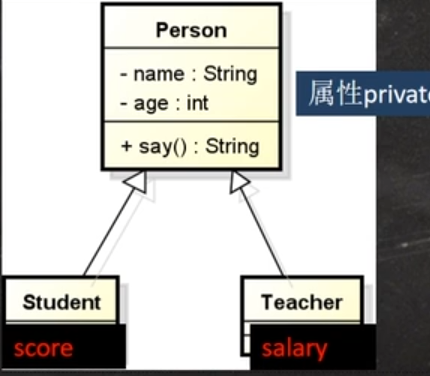
Person
package test;
public class Main {
public static void main(String[] args) {
//应用实例:现有一个继承结构如下:要求创建1个Person对象、
// 2个Student 对象和2个Teacher对象, 统一放在数组中,并调用每个对象say方法
//父类的引用可以指向子类的对象
Person[] persons = new Person[5];
persons[0] = new Person("jack", 20);
persons[1] = new Student("mary", 18, 100);
persons[2] = new Student("smith", 19, 30.1);
persons[3] = new Teacher("scott", 30, 20000);
persons[4] = new Teacher("king", 50, 25000);
//循环遍历多态数组,调用say
for (int i = 0; i < persons.length; i++) {
// person[i] 编译类型是 Person ,运行类型是是根据实际情况由JVM来判断
System.out.println(persons[i].say());//动态绑定机制
//编译类型Person中不含有这两个方法
// persons[i].teach();//报错!!!
// persons[i].study();//报错!!!
//解决方案: 使用 类型判断 + 向下转型.
if (persons[i] instanceof Student) {//判断person[i] 的运行类型是不是Student
Student student = (Student) persons[i];//向下转型
student.study();
//也可以使用一条语句 ((Student)persons[i]).study();
} else if (persons[i] instanceof Teacher) {
Teacher teacher = (Teacher) persons[i];
teacher.teach();
} else if (persons[i] instanceof Person) {
//就是Person类型,不做处理
} else {
System.out.println("你的类型有误, 请自己检查...");
}
}
}
}
Student
package test;
public class Student extends Person {
private double score;
public Student(String name, int age, double score) {
super(name, age);
this.score = score;
}
public double getScore() {
return score;
}
public void setScore(double score) {
this.score = score;
}
//重写父类say
@Override
public String say() {
return "学生 " + super.say() + " score=" + score;
}
//特有的方法
public void study() {
System.out.println("学生 " + getName() + " 正在学java...");
}
}
Teacher
package test;
public class Teacher extends Person {
private double salary;
public Teacher(String name, int age, double salary) {
super(name, age);
this.salary = salary;
}
public double getSalary() {
return salary;
}
public void setSalary(double salary) {
this.salary = salary;
}
//写重写父类的say方法
@Override
public String say() {
return "老师 " + super.say() + " salary=" + salary;
}
//特有方法
public void teach() {
System.out.println("老师 " + getName() + " 正在讲java课程...");
}
}
Main
package test;
public class Main {
public static void main(String[] args) {
//应用实例:现有一个继承结构如下:要求创建1个Person对象、
// 2个Student 对象和2个Teacher对象, 统一放在数组中,并调用每个对象say方法
//父类的引用可以指向子类的对象
Person[] persons = new Person[5];
persons[0] = new Person("jack", 20);
persons[1] = new Student("mary", 18, 100);
persons[2] = new Student("smith", 19, 30.1);
persons[3] = new Teacher("scott", 30, 20000);
persons[4] = new Teacher("king", 50, 25000);
//循环遍历多态数组,调用say
for (int i = 0; i < persons.length; i++) {
// person[i] 编译类型是 Person ,运行类型是是根据实际情况由JVM来判断
System.out.println(persons[i].say());//动态绑定机制
//编译类型Person中不含有这两个方法
// persons[i].teach();//报错!!!
// persons[i].study();//报错!!!
//解决方案: 使用 类型判断 + 向下转型.
if (persons[i] instanceof Student) {//判断person[i] 的运行类型是不是Student
Student student = (Student) persons[i];//向下转型
student.study();
//也可以使用一条语句 ((Student)persons[i]).study();
} else if (persons[i] instanceof Teacher) {
Teacher teacher = (Teacher) persons[i];
teacher.teach();
} else if (persons[i] instanceof Person) {
//就是Person类型,不做处理
} else {
System.out.println("你的类型有误, 请自己检查...");
}
}
}
}
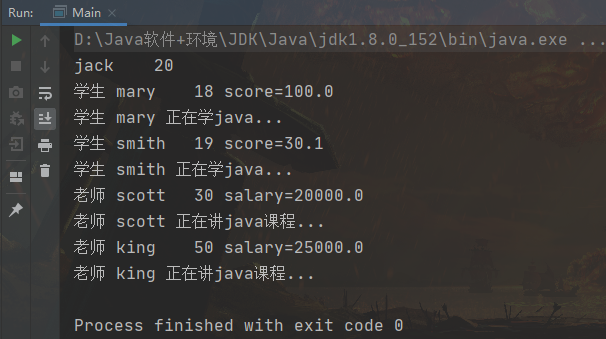