猜数字
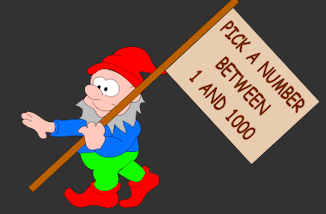
#!/usr/bin/env python
# encoding: utf-8
import random
""" Number Guessing Game
----------------------------------------
"""
attempts_list = []
def show_score():
if len(attempts_list) <= 0:
print("There is currently no high score, it's yours for the taking!\n")
else:
print("The current high score is {} attempts".format(min(attempts_list)))
def start_game():
print("Hello traveler! Welcome to the game of guesses!\n")
random_number = int(random.randint(1, 10))
player_name = input("What is your name? ")
wanna_play = input("Hi {}, would you like to play the guessing game? (Enter Yes/No) ".format(player_name))
attempts = 0
while wanna_play.lower() == "yes":
if attempts == 0:
show_score()
pass
try:
guess = input("\nPick a number between 1 and 10: ")
if int(guess) < 1 or int(guess) > 10:
raise ValueError("Please guess a number within the given range")
if int(guess) == random_number:
print("Nice! You got it!")
attempts += 1
attempts_list.append(attempts)
print("It took you {} attempts\n".format(attempts))
play_again = input("Would you like to play again? (Enter Yes/No) ")
attempts = 0
#show_score()
random_number = int(random.randint(1, 10))
if play_again.lower() == "no":
print("That's cool, have a good time!")
break
elif int(guess) > random_number:
print("It's lower")
attempts += 1
elif int(guess) < random_number:
print("It's higher")
attempts += 1
except ValueError as err:
print("Oh no!, that is not a valid value. Try again...")
print("({})".format(err))
pass
else:
print("That's cool, have a good time!")
if __name__ == '__main__':
start_game()
石头剪刀布
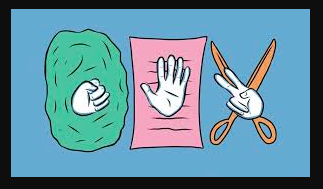
#!/usr/bin/env python
# encoding: utf-8
import random
import os
import re
""" Rock Paper Scissors
----------------------------------------
"""
while True:
print("\nRock, Paper, Scissors - Shoot!")
userChoice = input("Choose your weapon [R]ock], [P]aper, or [S]cissors: ")
if len(userChoice) < 1 or not re.match("[SsRrPp]", userChoice[0]):
print("Please choose a letter: ")
print("[R]ock, [S]cissors or [P]aper.")
continue
userChoice = userChoice[0].upper()
# Echo the user's choice
print("You chose: " + userChoice)
choices = ['R', 'P', 'S']
opponenetChoice = random.choice(choices)
print("I chose: " + opponenetChoice)
if opponenetChoice == str.upper(userChoice):
print("Tie! ")
elif opponenetChoice == 'R' and userChoice.upper() == 'S':
print("Rock beats scissors, I win! ")
continue
elif opponenetChoice == 'S' and userChoice.upper() == 'P':
print("Scissors beat paper! I win! ")
continue
elif opponenetChoice == 'P' and userChoice.upper() == 'R':
print("Paper beats rock, I win! ")
continue
else:
print("You win!")
pass
网址重定向
#!/usr/bin/env python
# encoding: utf-8
import time
from datetime import datetime as dt
""" Website Blocker
----------------------------------------
"""
hosts_path = r"/etc/hosts" # r is for raw string
hosts_temp = "hosts"
redirect = "127.0.0.1"
web_sites_list = ["www.baidu.com", "baidu.com"] # users can modify the list of the websites they want to block
while True:
now = dt.now()
if dt(now.year, now.month, now.day, 9) < now < dt(now.year, now.month, now.day, 12):
print("Working hours")
with open(hosts_path, "r") as file:
content = file.read()
for website in web_sites_list:
if website in content:
pass
else:
content += '\n' + redirect + " " + website
print(content)
#file.write(redirect + " " + website + "\n")
pass
pass
pass
else:
print("Fun time")
with open(hosts_path, "r") as file:
content = file.readlines()
file.seek(0) # reset the pointer to the top of the text file
for line in content:
# here comes the tricky line, basically we overwrite the whole file
if not any(website in line for website in web_sites_list):
print(line)
#file.write(line)
# do nothing otherwise
pass
#file.truncate() # this line is used to delete the trailing lines (that contain DNS)
pass
time.sleep(5)
二分查找
#!/usr/bin/env python
# encoding: utf-8
import random
""" Binary Search Algorithm
----------------------------------------
"""
#iterative implementation of binary search in Python
def binary_search(a_list, item):
"""
Performs iterative binary search to find the position of an integer in a given, sorted, list.
a_list -- sorted list of integers
item -- integer you are searching for the position of
"""
print('the list: ', a_list)
print('the item: ', item)
first = 0
last = len(a_list) - 1
while first <= last:
i = (first + last) // 2
if a_list[i] == item:
return 'found {item} at position {i}'.format(item=item, i=i)
elif a_list[i] > item:
last = i - 1
elif a_list[i] < item:
first = i + 1
else:
return 'not found {item} in the list'.format(item=item)
# recursive implementation of binary search in Python
def binary_search_recursive(a_list, item, idx):
"""
Performs recursive binary search of an integer in a given, sorted, list.
a_list -- sorted list of integers
item -- integer you are searching for the position of
"""
print('the list: ', a_list)
print('the item: ', item)
print('the idx: ', idx)
print()
first = 0
last = len(a_list) - 1
if len(a_list) == 0:
return '{item} was not found in the list'.format(item=item)
else:
i = (first + last) // 2
if item == a_list[i]:
return '{item} found at {i}'.format(item=item, i=idx+i)
else:
if a_list[i] < item:
return binary_search_recursive(a_list[i+1:], item, idx+i+1)
else:
return binary_search_recursive(a_list[:i], item, idx)
la = [random.randint(1, 30) for i in range(20)]
la.sort()
print(binary_search(la, 28))
print('\n\n---------------------')
print(binary_search_recursive(la, 28, 0))
计算器
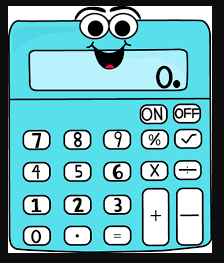
#!/usr/bin/env python
# encoding: utf-8
""" Calculator
----------------------------------------
"""
def addition ():
print("\n------------------\nAddition (enter 0 to calculate)")
n = float(input("Enter the number: "))
t = 0 #Total number enter
ans = 0
ipts = []
while n != 0:
ipts.append(n)
ans = ans + n
t += 1
n = float(input("Enter another number: "))
return [ans, ipts]
def subtraction ():
print("\n------------------\nSubtraction (enter 0 to calculate)");
n = float(input("Enter the number: "))
t = 0 #Total number enter
ans = 0
ipts = []
while n != 0:
ipts.append(n)
ans = ans - n
t += 1
n = float(input("Enter another number: "))
return [ans, ipts]
def multiplication ():
print("\n------------------\nMultiplication (enter 0 to calculate)")
n = float(input("Enter the number: "))
t = 0 #Total number enter
ans = 1
ipts = []
while n != 0:
ipts.append(n)
ans = ans * n
t += 1
n = float(input("Enter another number: "))
return [ans, ipts]
def average():
an = addition()
ans = an[0] / len(an[1])
return [ans, an[1]]
# main...
while True:
list = []
print("\n===================\nMy first Python Calculator!")
print("Enter 'a' for addition")
print("Enter 's' for substraction")
print("Enter 'm' for multiplication")
print("Enter 'v' for average")
print("Enter 'q' for quit")
c = input("operation: ")
if c != 'q':
if c == 'a':
list = addition()
print("Ans = ", list[0], "\nthe inputs: ", list[1])
elif c == 's':
list = subtraction()
print("Ans = ", list[0], "\nthe inputs ",list[1])
elif c == 'm':
list = multiplication()
print("Ans = ", list[0], "\nthe inputs ",list[1])
elif c == 'v':
list = average()
print("Ans = ", list[0], "\nthe inputs ",list[1])
else:
print ("Sorry, invilid character")
pass
pass
闹钟
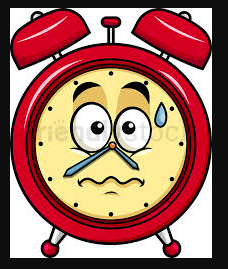
#!/usr/bin/env python
# encoding: utf-8
import datetime
import os
import time
import random
import webbrowser
""" Alarm Clock
----------------------------------------
"""
# If video URL file does not exist, create one
if not os.path.isfile("youtube_alarm_videos.txt"):
print('Creating "youtube_alarm_videos.txt"...')
with open("youtube_alarm_videos.txt", "w") as alarm_file:
alarm_file.write("https://www.youtube.com/watch?v=anM6uIZvx74")
pass
def check_alarm_input(alarm_time):
"""Checks to see if the user has entered in a valid alarm time"""
if len(alarm_time) == 1: # [Hour] Format
if alarm_time[0] < 24 and alarm_time[0] >= 0:
return True
elif len(alarm_time) == 2: # [Hour:Minute] Format
if alarm_time[0] < 24 and alarm_time[0] >= 0 and alarm_time[1] < 60 and alarm_time[1] >= 0:
return True
elif len(alarm_time) == 3: # [Hour:Minute:Second] Format
if alarm_time[0] < 24 and alarm_time[0] >= 0 and alarm_time[1] < 60 and alarm_time[1] >= 0 and alarm_time[2] < 60 and alarm_time[2] >= 0:
return True
return False
print('the current time: ', datetime.datetime.now())
# Get user input for the alarm time
print("Set a time for the alarm (Ex. 06:30 or 18:30:00)")
while True:
alarm_input = input(">> ")
try:
alarm_time = [int(n) for n in alarm_input.split(":")]
if check_alarm_input(alarm_time):
break
else:
raise ValueError
except ValueError:
print("ERROR: Enter time in HH:MM or HH:MM:SS format")
pass
# Convert the alarm time from [H:M] or [H:M:S] to seconds
seconds_hms = [3600, 60, 1] # Number of seconds in an Hour, Minute, and Second
alarm_seconds = sum([a*b for a,b in zip(seconds_hms[:len(alarm_time)], alarm_time)])
# Get the current time of day in seconds
now = datetime.datetime.now()
current_time_seconds = sum([a*b for a,b in zip(seconds_hms, [now.hour, now.minute, now.second])])
# Calculate the number of seconds until alarm goes off
time_diff_seconds = alarm_seconds - current_time_seconds
# If time difference is negative, set alarm for next day
if time_diff_seconds < 0:
time_diff_seconds += 86400 # number of seconds in a day
# Display the amount of time until the alarm goes off
#print("Alarm set to go off in %s" % datetime.timedelta(seconds=time_diff_seconds))
# Sleep until the alarm goes off
while time_diff_seconds > 0:
time.sleep(1)
time_diff_seconds -= 1
print("Alarm set to go off in %s" % datetime.timedelta(seconds=time_diff_seconds))
pass
#time.sleep(time_diff_seconds)
# Time for the alarm to go off
print("Wake Up!")
# Load list of possible video URLs
with open("youtube_alarm_videos.txt", "r") as alarm_file:
videos = alarm_file.readlines()
# Open a random video from the list
webbrowser.open(random.choice(videos))
Tic-Tac-Toe
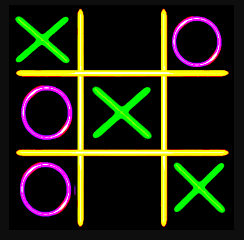
#!/usr/bin/env python
# encoding: utf-8
import random
import sys
""" Tic Tac Toe
----------------------------------------
"""
board = [i for i in range(0, 9)]
player, computer = '',''
# Corners, Center and Others, respectively
moves = ((1,7,3,9), (5,), (2,4,6,8))
# Winner combinations
winners = ((0,1,2), (3,4,5), (6,7,8), (0,3,6), (1,4,7), (2,5,8), (0,4,8), (2,4,6))
# Table
tab = range(1, 10)
def print_board():
x = 1
for i in board:
end = '\n' if x==9 else ' \n---------\n' if x%3==0 else ' | '
char = i if type(i) is str else ' '
x += 1
print(char, end=end)
pass
pass
def select_char():
chars = ('X','O')
if random.randint(0,1) == 0:
return chars[::-1]
return chars
def can_move(brd, player, move):
if move in tab and brd[move-1] == move-1:
return True
return False
def can_win(brd, player, move):
places=[]
x=0
for i in brd:
if i == player:
places.append(x);
x += 1
win = True
for tup in winners:
win = True
for ix in tup:
if brd[ix] != player:
win=False
break
if win == True:
break
return win
def make_move(brd, player, move, undo=False):
if can_move(brd, player, move):
brd[move-1] = player
win = can_win(brd, player, move)
if undo:
brd[move-1] = move-1
return (True, win)
return (False, False)
# AI goes here
def computer_move():
move = -1
# If I can win, others do not matter.
for i in range(1,10):
if make_move(board, computer, i, True)[1]:
move = i
break
if move == -1:
# If player can win, block him.
for i in range(1,10):
if make_move(board, player, i, True)[1]:
move=i
break
if move == -1:
# Otherwise, try to take one of desired places.
for tup in moves:
for mv in tup:
if move == -1 and can_move(board, computer, mv):
move=mv
break
return make_move(board, computer, move)
def space_exist():
return board.count('X') + board.count('O') != 9
player, computer = select_char()
print('Player is [%s] and computer is [%s]' % (player, computer))
result = '\n%%% Deuce ! %%%'
while space_exist():
print_board()
print('\n#Make your move ! [1-9] : ', end='')
move = input()
move = int(move) if move.isdigit() else 0
moved, won = make_move(board, player, move)
if not moved:
print('>> Invalid number ! Try again !')
continue
if won:
result='\n*** Congratulations ! You won ! ***'
break
elif computer_move()[1]:
result='\n=== You lose ! =='
break;
print_board()
print(result)
音乐播放器
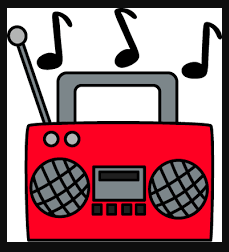
#!/usr/bin/env python
# encoding: utf-8
import os
import threading
import time
import tkinter.messagebox
from tkinter import *
from tkinter import filedialog
from tkinter import ttk
from ttkthemes import themed_tk as tk
from mutagen.mp3 import MP3
from pygame import mixer
""" Music Player
----------------------------------------
"""
root = tk.ThemedTk()
root.get_themes() # Returns a list of all themes that can be set
root.set_theme("radiance") # Sets an available theme
# Fonts - Arial (corresponds to Helvetica), Courier New (Courier), Comic Sans MS, Fixedsys,
# MS Sans Serif, MS Serif, Symbol, System, Times New Roman (Times), and Verdana
# Styles - normal, bold, roman, italic, underline, and overstrike.
statusbar = ttk.Label(root, text="Welcome to Melody", relief=SUNKEN, anchor=W, font='Times 10 italic')
statusbar.pack(side=BOTTOM, fill=X)
# Create the menubar
menubar = Menu(root)
root.config(menu=menubar)
# Create the submenu
subMenu = Menu(menubar, tearoff=0)
# playlist - contains the full path + filename
# playlistbox - contains just the filename
# Fullpath + filename is required to play the music inside play_music load function
playlist = []
def browse_file():
global filename_path
filename_path = filedialog.askopenfilename()
add_to_playlist(filename_path)
mixer.music.queue(filename_path)
def add_to_playlist(filename):
filename = os.path.basename(filename)
index = 0
playlistbox.insert(index, filename)
playlist.insert(index, filename_path)
index += 1
menubar.add_cascade(label="File", menu=subMenu)
subMenu.add_command(label="Open", command=browse_file)
subMenu.add_command(label="Exit", command=root.destroy)
def about_us():
tkinter.messagebox.showinfo('About Melody', 'This is a music player build using Python Tkinter')
pass
subMenu = Menu(menubar, tearoff=0)
menubar.add_cascade(label="Help", menu=subMenu)
subMenu.add_command(label="About Us", command=about_us)
mixer.init() # initializing the mixer
root.title("Melody")
root.iconbitmap(r'images/melody.ico')
# Root Window - StatusBar, LeftFrame, RightFrame
# LeftFrame - The listbox (playlist)
# RightFrame - TopFrame,MiddleFrame and the BottomFrame
leftframe = Frame(root)
leftframe.pack(side=LEFT, padx=30, pady=30)
playlistbox = Listbox(leftframe)
playlistbox.pack()
addBtn = ttk.Button(leftframe, text="+ Add", command=browse_file)
addBtn.pack(side=LEFT)
def del_song():
selected_song = playlistbox.curselection()
selected_song = int(selected_song[0])
playlistbox.delete(selected_song)
playlist.pop(selected_song)
pass
delBtn = ttk.Button(leftframe, text="- Del", command=del_song)
delBtn.pack(side=LEFT)
rightframe = Frame(root)
rightframe.pack(pady=30)
topframe = Frame(rightframe)
topframe.pack()
lengthlabel = ttk.Label(topframe, text='Total Length : --:--')
lengthlabel.pack(pady=5)
currenttimelabel = ttk.Label(topframe, text='Current Time : --:--', relief=GROOVE)
currenttimelabel.pack()
def show_details(play_song):
file_data = os.path.splitext(play_song)
if file_data[1] == '.mp3':
audio = MP3(play_song)
total_length = audio.info.length
else:
a = mixer.Sound(play_song)
total_length = a.get_length()
# div - total_length/60, mod - total_length % 60
mins, secs = divmod(total_length, 60)
mins = round(mins)
secs = round(secs)
timeformat = '{:02d}:{:02d}'.format(mins, secs)
lengthlabel['text'] = "Total Length" + ' - ' + timeformat
t1 = threading.Thread(target=start_count, args=(total_length,))
t1.start()
def start_count(t):
global paused
# mixer.music.get_busy(): - Returns False when we press the stop button (music stop playing)
# Continue - Ignores all of the statements below it. We check if music is paused or not.
current_time = 0
while current_time <= t and mixer.music.get_busy():
if paused:
continue
else:
mins, secs = divmod(current_time, 60)
mins = round(mins)
secs = round(secs)
timeformat = '{:02d}:{:02d}'.format(mins, secs)
currenttimelabel['text'] = "Current Time" + ' - ' + timeformat
time.sleep(1)
current_time += 1
pass
pass
def play_music():
global paused
if paused:
mixer.music.unpause()
statusbar['text'] = "Music Resumed"
paused = False
else:
try:
stop_music()
time.sleep(1)
selected_song = playlistbox.curselection()
selected_song = int(selected_song[0])
play_it = playlist[selected_song]
mixer.music.load(play_it)
mixer.music.play()
statusbar['text'] = "Playing music" + ' - ' + os.path.basename(play_it)
show_details(play_it)
except:
tkinter.messagebox.showerror('File not found', 'Melody could not find the file. Please check again.')
pass
def stop_music():
mixer.music.stop()
statusbar['text'] = "Music Stopped"
paused = False
def pause_music():
global paused
paused = True
mixer.music.pause()
statusbar['text'] = "Music Paused"
def rewind_music():
play_music()
statusbar['text'] = "Music Rewinded"
def set_vol(val):
volume = float(val) / 100
mixer.music.set_volume(volume)
# set_volume of mixer takes value only from 0 to 1. Example - 0, 0.1,0.55,0.54.0.99,1
muted = False
def mute_music():
global muted
if muted: # Unmute the music
mixer.music.set_volume(0.7)
volumeBtn.configure(image=volumePhoto)
scale.set(70)
muted = False
else: # mute the music
mixer.music.set_volume(0)
volumeBtn.configure(image=mutePhoto)
scale.set(0)
muted = True
pass
middleframe = Frame(rightframe)
middleframe.pack(pady=30, padx=30)
playPhoto = PhotoImage(file='images/play.png')
playBtn = ttk.Button(middleframe, image=playPhoto, command=play_music)
playBtn.grid(row=0, column=0, padx=10)
stopPhoto = PhotoImage(file='images/stop.png')
stopBtn = ttk.Button(middleframe, image=stopPhoto, command=stop_music)
stopBtn.grid(row=0, column=1, padx=10)
pausePhoto = PhotoImage(file='images/pause.png')
pauseBtn = ttk.Button(middleframe, image=pausePhoto, command=pause_music)
pauseBtn.grid(row=0, column=2, padx=10)
# Bottom Frame for volume, rewind, mute etc.
bottomframe = Frame(rightframe)
bottomframe.pack()
rewindPhoto = PhotoImage(file='images/rewind.png')
rewindBtn = ttk.Button(bottomframe, image=rewindPhoto, command=rewind_music)
rewindBtn.grid(row=0, column=0)
mutePhoto = PhotoImage(file='images/mute.png')
volumePhoto = PhotoImage(file='images/volume.png')
volumeBtn = ttk.Button(bottomframe, image=volumePhoto, command=mute_music)
volumeBtn.grid(row=0, column=1)
scale = ttk.Scale(bottomframe, from_=0, to=100, orient=HORIZONTAL, command=set_vol)
scale.set(70) # implement the default value of scale when music player starts
mixer.music.set_volume(0.7)
scale.grid(row=0, column=2, pady=15, padx=30)
def on_closing():
stop_music()
root.destroy()
pass
root.protocol("WM_DELETE_WINDOW", on_closing)
root.mainloop()
assets.zip