实验现象

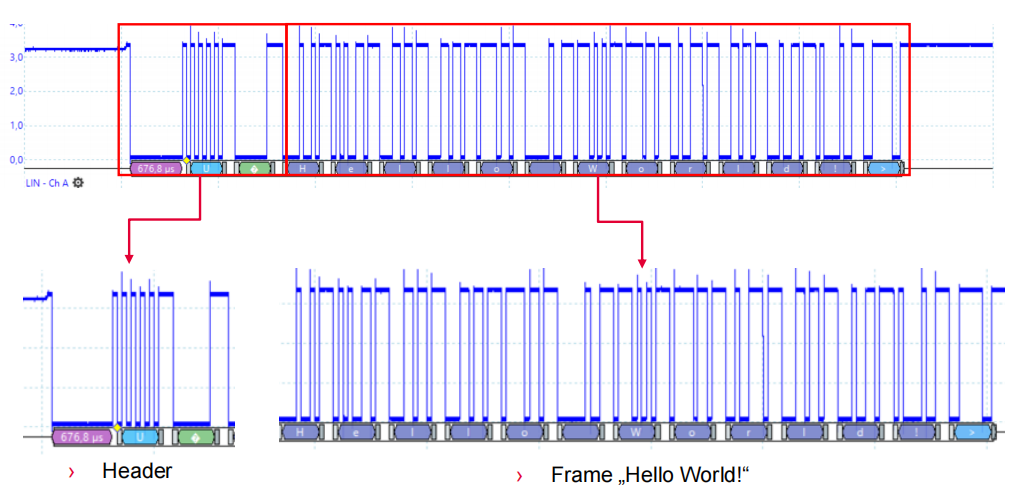
LIN控制子系统
代码
ASCLIN_LIN_Master.c

/*********************************************************************************************************************/
/*-----------------------------------------------------Includes------------------------------------------------------*/
/*********************************************************************************************************************/
#include "IfxAsclin.h"
#include "IfxAsclin_Lin.h"
#include "ASCLIN_LIN_Master.h"
/*********************************************************************************************************************/
/*------------------------------------------------------Macros-------------------------------------------------------*/
/*********************************************************************************************************************/
#define SERIAL_BAUDRATE 19200 /* Set the baud rate */
#define SIZE 12 /* Size of the message */
#define ID_BYTE 0x80 /* Set the LIN ID byte to a random number */
#define RX_PIN IfxAsclin1_RXB_P15_5_IN /* Set RX port pin */
#define TX_PIN IfxAsclin1_TX_P15_5_OUT /* Set TX port pin */
/*********************************************************************************************************************/
/*-------------------------------------------------Global variables--------------------------------------------------*/
/*********************************************************************************************************************/
static IfxAsclin_Lin g_linMaster;
/*********************************************************************************************************************/
/*----------------------------------------------Function Implementations---------------------------------------------*/
/*********************************************************************************************************************/
/* This function initializes the ASCLIN LIN module in master mode */
void init_ASCLIN_LIN_master(void)
{
/* Initialize one instance of IfxAsclin_Lin_Config with default values */
IfxAsclin_Lin_Config linMasterConfig;
IfxAsclin_Lin_initModuleConfig(&linMasterConfig, &MODULE_ASCLIN1);
linMasterConfig.linMode = IfxAsclin_LinMode_master; /* Set the LIN mode of operation */
linMasterConfig.brg.baudrate = SERIAL_BAUDRATE; /* Set the desired baud rate */
const IfxAsclin_Lin_Pins pins =
{
&RX_PIN, IfxPort_InputMode_pullUp, /* RX port pin */
&TX_PIN, IfxPort_OutputMode_pushPull, /* TX port pin */
IfxPort_PadDriver_cmosAutomotiveSpeed1
};
linMasterConfig.pins = &pins; /* Port pins configuration */
/* Initialize module */
IfxAsclin_Lin_initModule(&g_linMaster, &linMasterConfig);
}
/* This function sends the string "Hello World!" */
void send_ASCLIN_LIN_message(void)
{
uint8 txId = ID_BYTE; /* ID which gets transmitted within the header */
uint8 txData[SIZE] = {"Hello World!"}; /* Frame to send */
/* After the transmission of the LIN header by the master, the master itself starts the transmission
* of the message.
*/
IfxAsclin_Lin_sendHeader(&g_linMaster, &txId); /* Send LIN header */
if(g_linMaster.acknowledgmentFlags.txHeaderEnd == 1) /* If the LIN header has been transmitted */
{
IfxAsclin_Lin_sendResponse(&g_linMaster, txData, SIZE); /* Send message */
}
}