html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>案例:计算商品价格</title>
<!-- IMPORT CSS -->
<link rel="stylesheet" type="text/css" href="price.css" />
</head>
<body>
<div class="wrap">
<div class="box">
<ul class="list">
<!-- <li>
<i></i>
<em>0</em>
<i></i>
<span>
单价:<strong>12.5元</strong>
小计:<strong>0元</strong>
</span>
</li> -->
</ul>
<div class="info">
<!-- <span>商品公合计:<em>0</em>件</span>
<span>共花费了:<em>0</em>元</span>
<span>其中最贵的商品单价是:<em>0</em>元</span> -->
</div>
</div>
</div>
<!-- IMPORT JS -->
<script src="../node_modules/jquery/dist/jquery.min.js"></script>
<script src="index.js"></script>
</body>
</html>
css
body,
ul,
li {
margin: 0;
padding: 0;
list-style: none;
}
.wrap {
margin: 0 auto;
width: 764px;
display: flex;
}
.wrap .box {
position: relative;
width: 479px;
height: 591px;
background: url(bg1.png) no-repeat center center;
}
.wrap .box .list {
position: absolute;
left: 0;
top: 90px;
width: 100%;
}
.wrap .box .list li {
box-sizing: border-box;
margin-bottom: 18px;
padding-left: 44px;
height: 44px;
overflow: hidden;
}
.wrap .box .list li i {
float: left;
margin-right: 10px;
width: 52px;
height: 44px;
cursor: pointer;
}
.wrap .box .list li i:nth-of-type(1) {
background: url(sub.png) no-repeat center center;
}
.wrap .box .list li i:nth-of-type(2) {
background: url(add.png) no-repeat center center;
}
.wrap .box .list li em {
width: 44px;
height: 36px;
float: left;
background: #fff;
border-radius: 5px;
font: 16px/36px arial;
text-align: center;
margin-right: 10px;
}
.wrap .box .list li span {
box-sizing: border-box;
float: left;
margin-left: 10px;
padding-left: 3px;
width: 214px;
height: 36px;
line-height: 36px;
border-radius: 5px;
background: #171818;
color: #878787;
font-size: 14px;
}
.wrap .box .list li span strong {
margin-right: 10px;
}
.info {
width: 100%;
height: 140px;
position: absolute;
left: 0;
bottom: 0;
color: #878787;
box-sizing: border-box;
padding: 20px 0 0 42px;
}
.info em {
width: 46px;
height: 36px;
display: inline-block;
background: #fff;
border-radius: 5px;
font: 16px/36px arial;
text-align: center;
margin: 0 10px 0;
}
.info span:nth-of-type(2) em {
width: 66px;
}
.info span:nth-of-type(3) em {
width: 66px;
}
.info span:nth-of-type(1) {
margin-right: 20px;
}
js:操作dom方式
// 操作dom方式
let cartModule = (function ($) {
let $btns = $('.list i'),
$counts = $('.list em'),
$strongs = $('.list strong'),
$ems = $('.info em');
// 实现加号减号的点击事件
function handleClick() {
$btns.click(function () {
let $this = $(this),
n = $this.index(); // JQ 中的 INDEX 获取的是元素在兄弟结构中的索引
// 根据点击按钮,获取当前行中:存储数字、单价、总价这几个元素
let $par = $this.parent(),
$count = $par.children('em'),
$strongs = $par.find('strong'),
$price = $strongs.eq(0), // eq 获取的依然是 JQ 对象,get 获取的是 JS 对象
$total = $strongs.eq(1);
// 0减号 2加号 根据点击的加减号,计算出最新购买的数量
let x = parseFloat($count.html());
if (n === 0) {
x--;
x < 0 ? x = 0 : null;
} else {
x++;
x > 10 ? x = 10 : null;
}
$count.html(x);
// 获取单价计算总价
$total.html(parseFloat($price.html()) * x + '元');
// 计算总信息
computed();
});
}
// 计算总信息
function computed () {
let allCount = 0,
allMoney = 0,
allPrice = [];
// 计算总购买数
$counts.each((index, item) => {
allCount += parseFloat($(item).html());
});
$ems.eq(0).html(allCount);
// 计算总价格和最高单价
$strongs.each((index, item) => {
let itemVal = parseFloat($(item).html());
if (index % 2 === 1) {
allMoney += itemVal;
} else {
// 只有购买了才进入比较的序列
if (parseFloat($(item).next('strong').html()) !== 0) {
allPrice.push(itemVal);
}
}
});
$ems.eq(1).html(allMoney);
$ems.eq(2).html(Math.max(...allPrice));
}
return {
init() { // init:function(){}
handleClick();
}
}
})(jQuery);
cartModule.init();
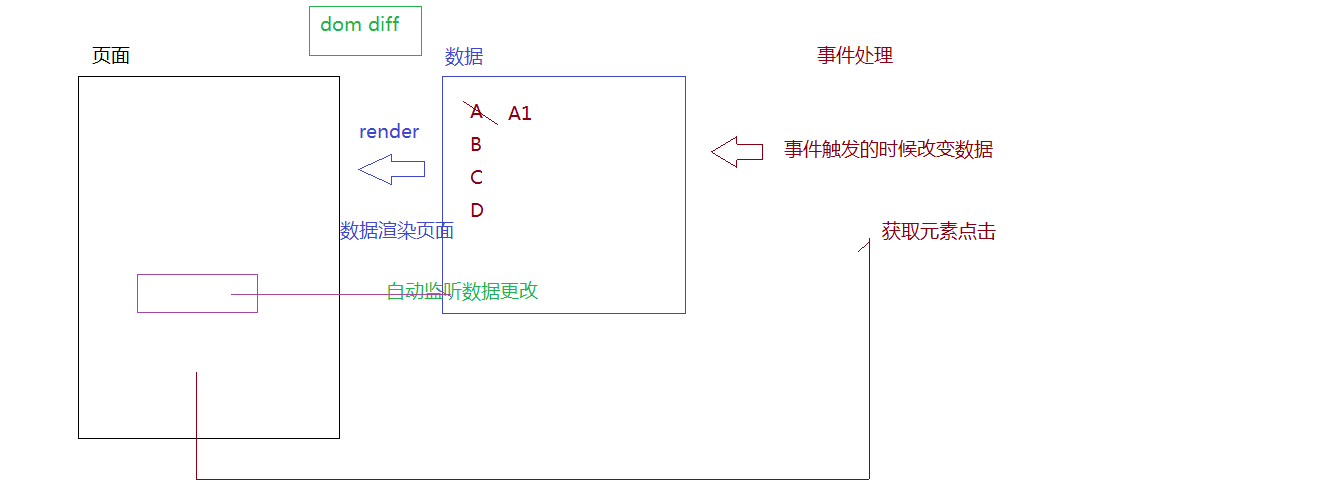
js:操作数据模式
let cartModule = (function ($) {
let $list = $('.list'),
$info = $('.info'),
$btns = null;
// 准备数据模型(页面就是按照数据模型渲染出来的)
let _DATA = [{
id: 1,
count: 0,
price: 12.5,
total: 0
}, {
id: 2,
count: 0,
price: 10.5,
total: 0
}, {
id: 3,
count: 0,
price: 8.5,
total: 0
}, {
id: 4,
count: 0,
price: 8,
total: 0
}, {
id: 5,
count: 0,
price: 14.5,
total: 0
}];
// render:按照数据模型渲染视图
function render() {
// 渲染操作区域视图
let str = ``;
$.each(_DATA, (index, item) => {
let {count, price, total, id} = item;
str += `<li>
<i group="${id}"></i>
<em>${count}</em>
<i group="${id}"></i>
<span>
单价:<strong>${price}元</strong>
小计:<strong>${total}元</strong>
</span>
</li>`;
});
$list.html(str);
// 渲染总计信息区视图
let counts = 0,
totals = 0,
maxprice = 0;
_DATA.forEach(item => {
counts += item.count;
totals += item.total;
// 购买才进入最高价格的计算
if (item.count > 0) {
maxprice < item.price ? maxprice = item.price : null;
}
});
$info.html(`<span>商品公合计:<em>${counts}</em>件</span>
<span>共花费了:<em>${totals}</em>元</span>
<span>其中最贵的商品单价是:<em>${maxprice}</em>元</span>`);
// 执行事件绑定
handle();
}
// handle:点击按钮操作(不操作 DOM,只改变 _DATA 的数据)
function handle() {
$btns = $list.find('i');
$btns.click(function () {
let $this = $(this),
n = $this.index(),
group = parseFloat($this.attr('group'));
// 修改数据
_DATA = _DATA.map(item => {
if (item.id === group) {
if (n === 0) {
item.count--;
item.count < 0 ? item.count = 0 : null;
} else {
item.count++;
item.count > 10 ? item.count = 10 : null;
}
item.total = item.price * item.count;
}
return item;
});
// 重新渲染
render();
});
}
return {
init() {
render();
}
}
})(jQuery);
cartModule.init();
图片资源