data.json
一、基于 ztree 插件实现
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>zTree</title>
<!-- IMPORT CSS -->
<link rel="stylesheet" href="css/reset.min.css">
<link rel="stylesheet" href="css/zTreeStyle.css">
</head>
<body>
<ul class="ztree" id="ztree1"></ul>
<!-- IMPORT JS -->
<script src="js/jquery.min.js"></script>
<script src="js/jquery.ztree.all.min.js"></script>
<script>
// $.fn.zTree.init([容器],[配置项],[数据]);
let $ztree1 = $('#ztree1'),
options = {};
$.ajax({
url: 'data.json',
method: 'GET',
success: result => {
$.fn.zTree.init($ztree1, options, result);
}
})
</script>
</body>
</html>
二、基于 jQuery 的 ztree 插件
~ function ($) {
function ztree(data) {
let count = 0,
$this = $(this);
// 数据绑定
let bindHTML = function (result) {
let str = ``;
result.forEach(item => {
count++;
let {
name,
open,
children
} = item;
str += `<li>
<a href="" class="title">${name}</a>
${children?`<em class="icon ${open?'open':''}"></em>
<ul class="level level${count}"
style="display:${open?'block':'none'}">
${bindHTML(children)}
</ul>`:``}
</li>`;
count--;
});
return str;
};
$this.html(bindHTML(data));
// 基于事件委托实现点击操作
$this.click(function (ev) {
let target = ev.target,
$target = $(target),
$next = $target.next('ul');
if (target.tagName === 'EM') {
$target.toggleClass('open');
$next.stop().slideToggle(100);
}
});
}
$.fn.extend({
ztree: ztree
});
}(jQuery);
三、自己模拟一个ztree
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>zTree树形结构菜单</title>
<!-- import css -->
<link rel="stylesheet" href="css/reset.min.css">
<style>
.container {
box-sizing: border-box;
margin: 20px auto;
padding: 10px;
width: 600px;
border: 1px dashed #AAA;
-webkit-user-select: none;
}
.level {
display: none;
font-size: 14px;
margin-left: 10px;
}
.level.level0 {
display: block;
margin-left: 0;
}
.level li {
position: relative;
padding-left: 15px;
line-height: 30px;
}
.level li .icon {
position: absolute;
left: 0;
top: 9px;
box-sizing: border-box;
width: 12px;
height: 12px;
line-height: 8px;
text-align: center;
border: 1px solid #AAA;
background: #EEE;
cursor: pointer;
}
.level li .icon:after {
display: block;
content: "+";
font-size: 12px;
font-style: normal;
}
.level li .icon.open:after {
content: "-";
}
.level li .title {
color: #000;
}
</style>
</head>
<body>
<div class="container">
<ul class="level level0" id="tree1"></ul>
</div>
<div class="container">
<ul class="level level0" id="tree2"></ul>
</div>
<!-- import js -->
<script src="js/jquery.min.js"></script>
<script src="js/ztree-plugin.js"></script>
<script>
$.ajax({
url: 'data.json',
method: 'get',
success: result => {
$('#tree1').ztree(result);
$('#tree2').ztree(result);
}
});
</script>
<script>
/* let treeModule = (function () {
let $level0 = $('.level0'),
count = 0;
// 获取数据
let queryData = function (callBack) {
$.ajax({
url: 'data.json',
method: 'get',
success: callBack
// success: result => {
// callBack(result);
// }
});
};
// 数据绑定
let bindHTML = function (result) {
let str = ``;
result.forEach(item => {
count++;
let {
name,
open,
children
} = item;
str += `<li>
<a href="" class="title">${name}</a>
${children?`<em class="icon ${open?'open':''}"></em>
<ul class="level level${count}"
style="display:${open?'block':'none'}">
${bindHTML(children)}
</ul>`:``}
</li>`;
count--;
});
return str;
};
return {
init() {
// 基于事件委托实现点击操作
$level0.click(function (ev) {
let target = ev.target,
$target = $(target),
$next = $target.next('ul');
if (target.tagName === 'EM') {
// 加减号的切换
$target.toggleClass('open');
// 控制子集的显示隐藏
$next.stop().slideToggle(100);
}
});
queryData(function anonymous(result) {
// 获取数据后完成相关的事项
$level0.html(bindHTML(result));
});
}
}
})();
treeModule.init();
</script>
</body>
</html>
四、基于原生 js 实现鼠标拖拽
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>DRAG-拖拽</title>
<!-- IMPORT CSS -->
<link rel="stylesheet" href="css/reset.min.css">
<style>
html,
body {
height: 100%;
overflow: hidden;
}
.box {
position: absolute;
top: 0;
left: 0;
width: 100px;
height: 100px;
background: red;
cursor: move;
}
</style>
</head>
<body>
<div class="box" id="box"></div>
<script>
box.addEventListener('mousedown', down);
function down(ev) {
this.startX = ev.pageX;
this.startY = ev.pageY;
this.startL = this.offsetLeft;
this.startT = this.offsetTop;
// 把执行 BIND 处理后的函数存储到盒子的自定义属性上,绑定的时候绑定存储的这个方法,移除的时候也基于自定义属性获取到这个方法移除
this._MOVE = move.bind(this);
this._UP = up.bind(this);
document.addEventListener('mousemove', this._MOVE);
document.addEventListener('mouseup', this._UP);
}
function move(ev) {
let curL = ev.pageX - this.startX + this.startL,
curT = ev.pageY - this.startY + this.startT;
let minL = 0,
minT = 0,
maxL = document.documentElement.clientWidth - this.offsetWidth,
maxT = document.documentElement.clientHeight - this.offsetHeight;
curL = curL < minL ? minL : (curL > maxL ? maxL : curL);
curT = curT < minT ? minT : (curT > maxT ? maxT : curT);
this.style.left = curL + 'px';
this.style.top = curT + 'px';
}
function up(ev) {
document.removeEventListener('mousemove', this._MOVE);
document.removeEventListener('mouseup', this._UP);
}
</script>
<script>
/* box.onmousedown = down;
function down(ev) {
// 把鼠标起始位置信息和盒子起始位置信息存储到盒子的自定义属性上
this.startX = ev.pageX;
this.startY = ev.pageY;
this.startL = this.offsetLeft;
this.startT = this.offsetTop;
// 按下来在给盒子绑定 MOVE 方法
// 谷歌浏览器中解决鼠标焦点丢失的问题(别绑定给盒子了,绑定给 document),但是要注意 move 中的 this 已经是 document 了,而不是之前的 box,我们需要处理一下
document.onmousemove = move.bind(this);
document.onmouseup = up.bind(this);
}
function move(ev) {
// 随时获取当前鼠标的信息,计算盒子最新的位置
let curL = ev.pageX - this.startX + this.startL,
curT = ev.pageY - this.startY + this.startT;
// 边界判断
let minL = 0,
minT = 0,
maxL = document.documentElement.clientWidth - this.offsetWidth,
maxT = document.documentElement.clientHeight - this.offsetHeight;
curL = curL < minL ? minL : (curL > maxL ? maxL : curL);
curT = curT < minT ? minT : (curT > maxT ? maxT : curT);
this.style.left = curL + 'px';
this.style.top = curT + 'px';
}
function up(ev) {
// 鼠标抬起,把 MOVE 移除掉
document.onmousemove = null;
document.onmouseup = null;
}
*/
/*
* // 把鼠标和当前盒子拿绳子捆在一起
this.setCapture();
// 把鼠标和盒子解绑
this.releaseCapture();
谷歌不支持
*/
</script>
</body>
</html>
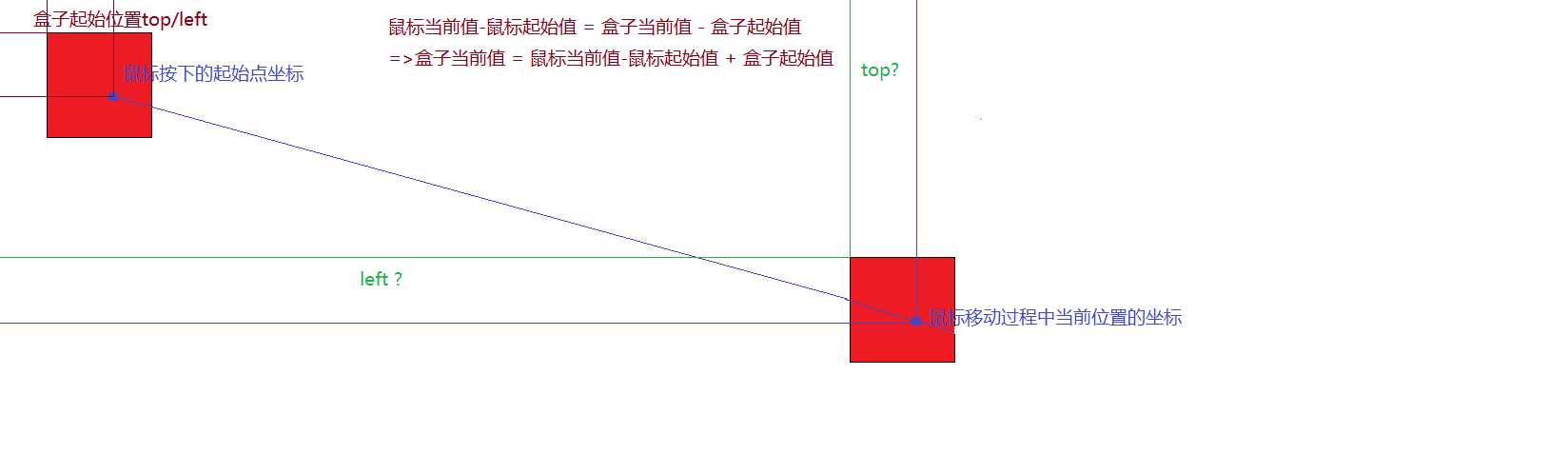