单链表添加节点
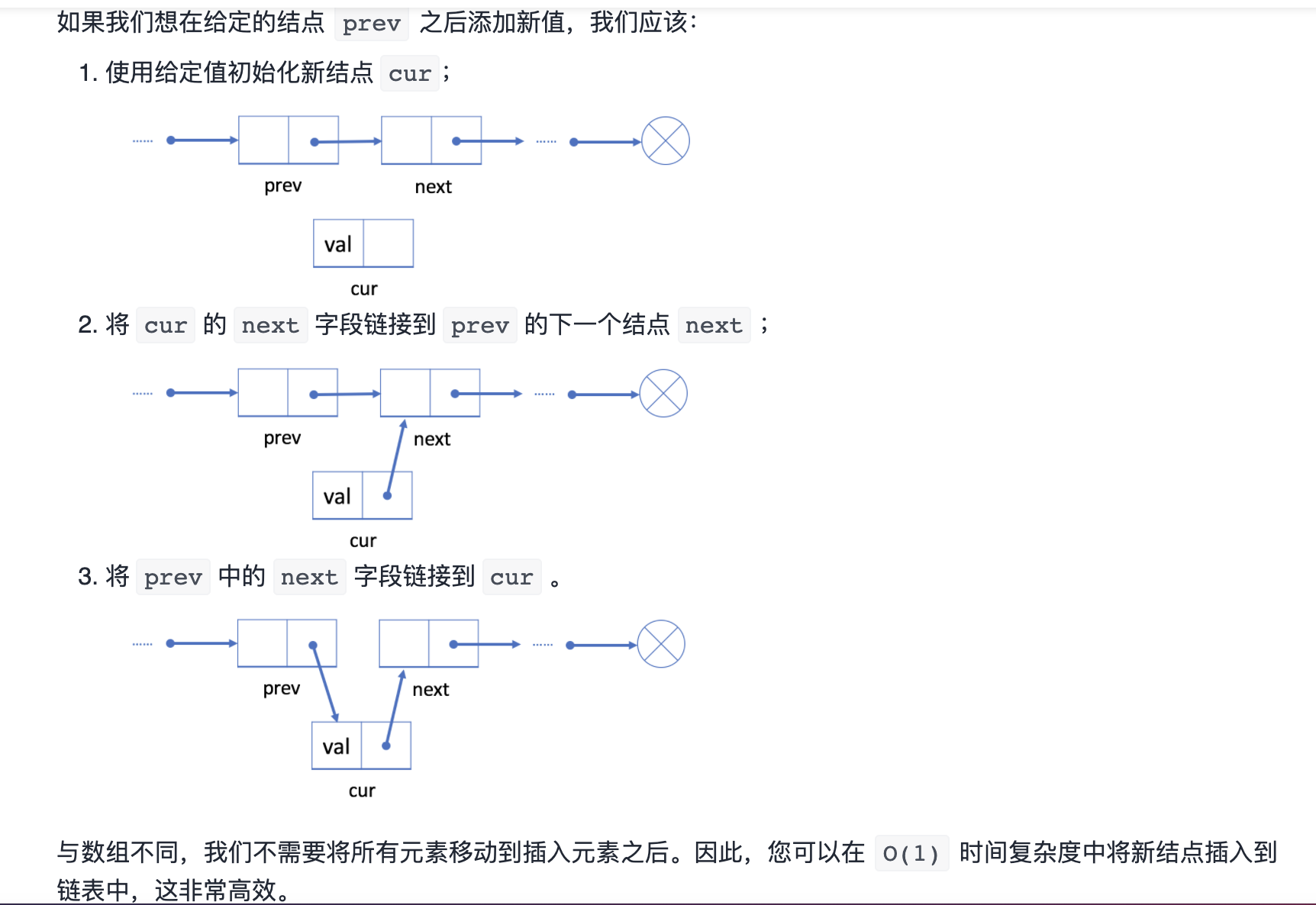
function insert(head,val){
const newNode = listNode(9)
const cur = head
while(head.next && !cur === val){
cur = head.next
}
const next = cur.next
newNode.next = next
cur.next = newNode
return head
}
在链表头部添加
const newNode = listNode(0)
newNode.next = head
head = newNode
return head
在链表尾部添加
while(head.next){
// 移动 head 指针
head = head.next;
}
// 新节点插入尾部
head.next = newNode;
单链表删除节点
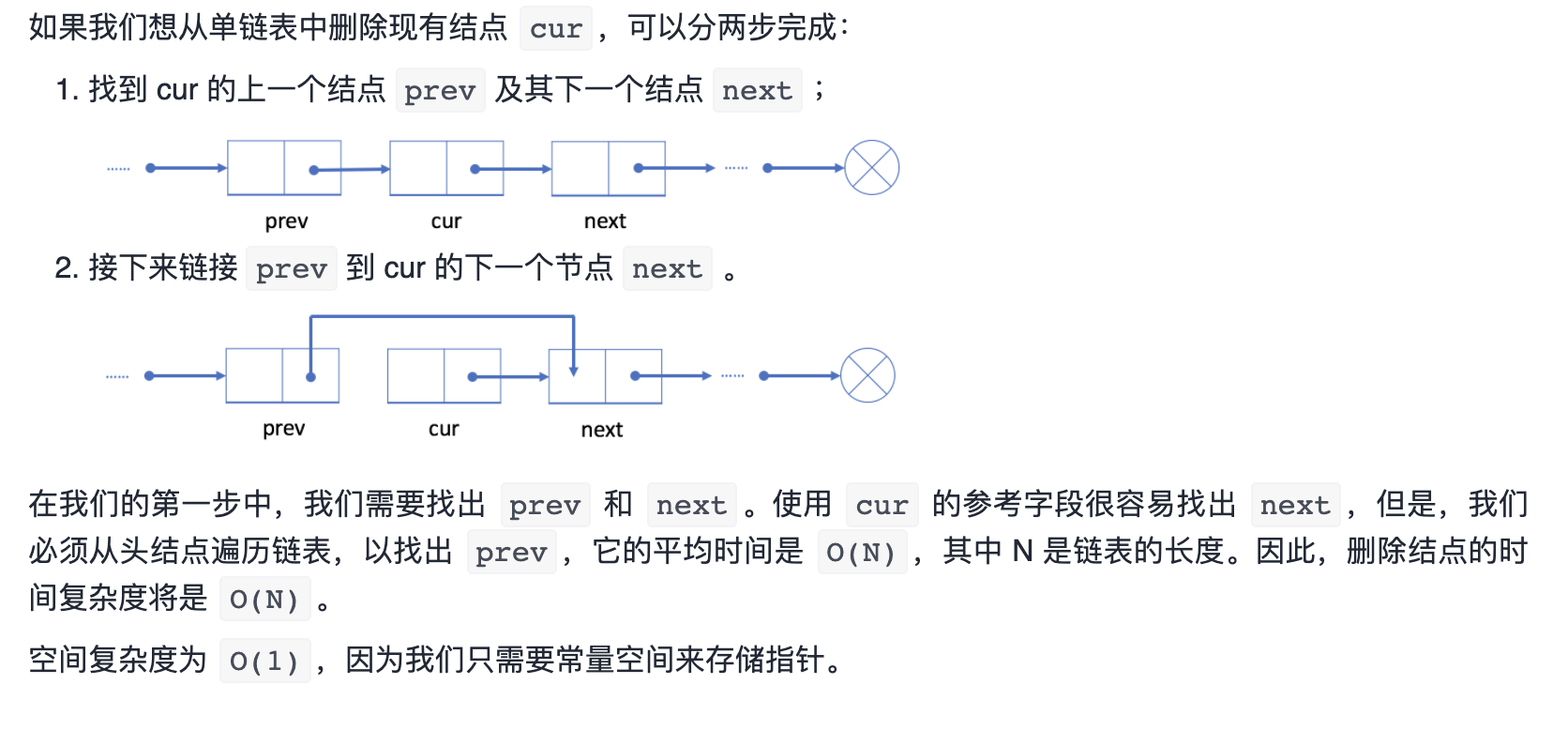
function deleteNode(head, val){
if(head === null) return
let prev = null
let cur = head
while(head.next && cur !== val){
prev = cur
cur = head.next
}
prev.next = cur.next
return head
}