键盘事件
// document.onkeydown=function(){
// console.log('keydown');
// //不抬起按键会一直触发
// }
// document.onkeyup=function() {
// console.log('keyup')
// }
// document.onkeypress=function() {
// console.log('keypress');
// }
//keydown->keypress->keyup
//所有按键都可以触发
document.onkeydown=function(e){
console.log(e);
console.log(e.charCode)//0 无数字
console.log(e.keyCode)//键盘顺位码
//keyCode有上下左右
}
//只能触发字符类按键,上下左右不行
document.onkeypress=function(e) {
console.log(e);
console.log(e.charCode)//1 有数字 ascii码
console.log(e.keyCode);//一样 有
var str=String.fromCharCode(e.charCode)
console.log(str);
//能得到大写的G、H、L,keyCode不行
//只能触发字符类,别的不行,shift、上下左右
//keyCode没有上下左右
}
keyCode表
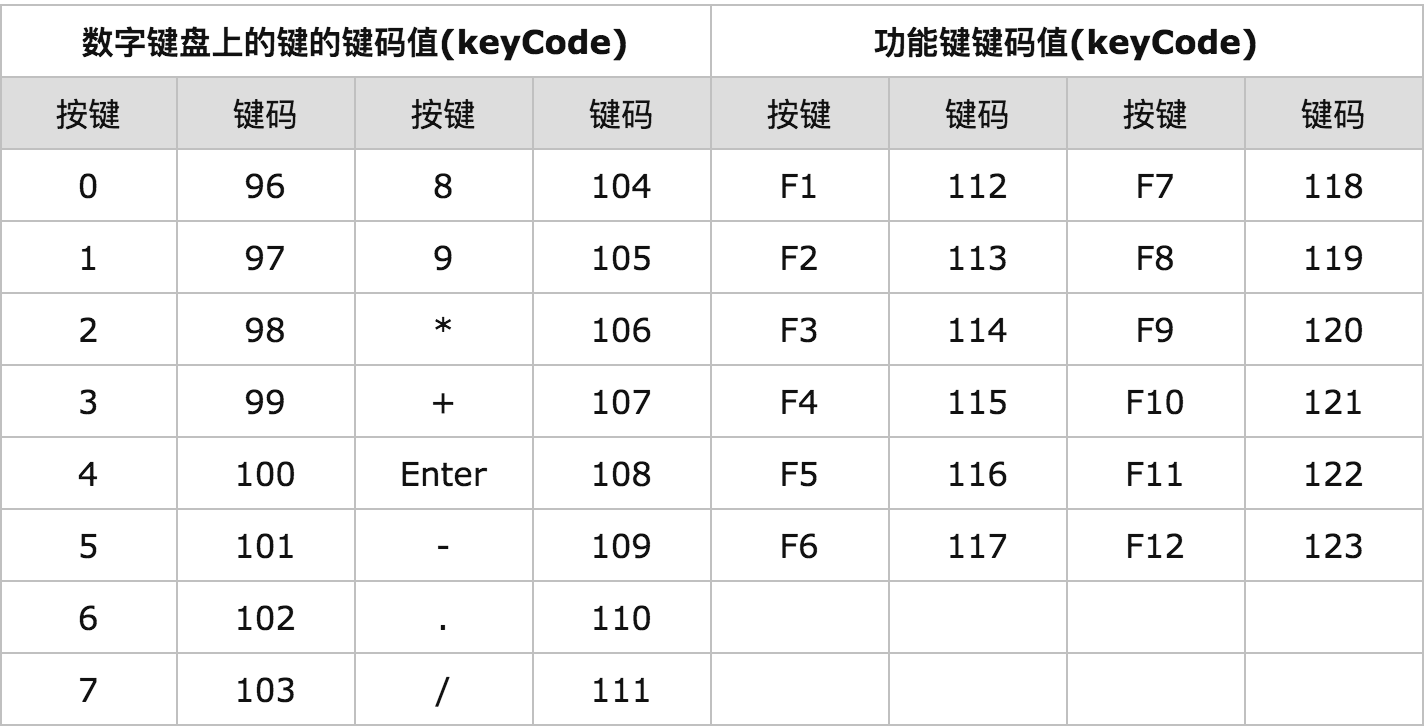
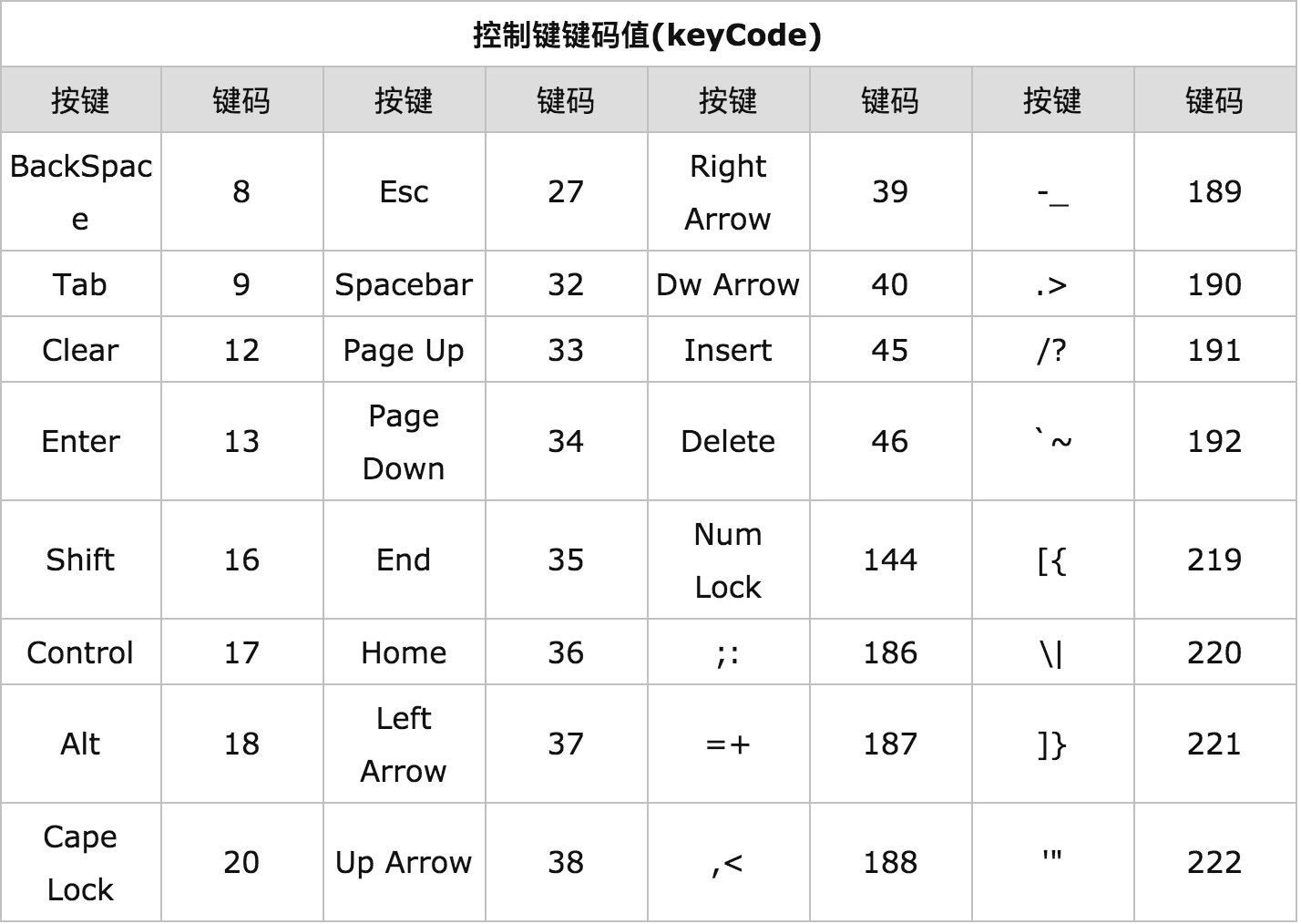
例子
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title></title>
<style>
.box {
position: absolute;
top: 0;
left: 0;
width: 100px;
height: 100px;
background-color: green;
}
</style>
</head>
<body>
<div class="box"></div>
<script src="./js/utils.js"></script>
<script>
var box = document.getElementsByClassName('box')[0];
document.onkeydown = function (e) {
var e = e || window.event,
code = e.keyCode,
bLeft = getStyles(box, 'left'),
bTop = getStyles(box, 'top');
switch (code) {
case 37:
box.style.left = bLeft - 5 + 'px';
break;
case 39:
box.style.left = bLeft + 5 + 'px';
break;
case 38:
box.style.top = bTop -5 + 'px';
break;
case 40:
box.style.top = bTop + 5 + 'px';
break;
default:
break;
}
}
</script>
</body>
</html>
贪吃蛇
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
.wrap{
position: relative;
width: 500px;
height: 500px;
margin: 50px auto;
background-color: #000;
overflow: hidden;
}
.round{
display: block;
position: absolute;
width: 20px;
height: 20px;
border-radius: 50%;
background-color: green;
z-index: 1;
}
.round.head{
background-color: red;
}
.food{
display: block;
position: absolute;
width: 20px;
height: 20px;
border-radius: 50%;
background-color: yellow;
z-index: 2;
}
</style>
</head>
<body>
<div class="wrap"></div>
<script src="./js/untils.js"></script>
<script src="./js/index.js"></script>
</body>
</html>
window.onload = function () {
init();
}
function init() {
initGame();
}
var initGame = (function () {
var wrap = document.getElementsByClassName('wrap')[0],
wrapW = getStyles(wrap, 'width'),
wrapH = getStyles(wrap, 'height'),
t = null;
var Snake = function () {
this.bodyArr = [{
x: 0,
y: 0
},
{
x: 0,
y: 20
},
{
x: 0,
y: 40
},
{
x: 0,
y: 60
},
{
x: 0,
y: 80
},
{
x: 0,
y: 100
}
];
this.dir = 'DOWN'
}
Snake.prototype = {
init: function () {
this.bindEvent();
this.createFood();
this.initSnake();
this.run();
},
bindEvent: function () {
var _self = this;
addEvent(document, 'keydown', function () {
_self.changeDir();
});
},
initSnake: function () {
var arr = this.bodyArr,
len = arr.length,
frag = document.createDocumentFragment(),
item;
for (var i = 0; i < len; i++) {
item = arr[i];
var round = document.createElement('i'); //i标签
// console.log(round);
// 此处i是循坏中的i 查找数组中最后一项
round.className = i === len - 1 ? 'round head' : 'round';
round.style.left = item.x + 'px';
round.style.top = item.y + 'px';
frag.appendChild(round);
}
wrap.appendChild(frag);
},
run: function () {
var _self = this
t = setInterval(function () {
_self.move();
}, 500);
},
move: function () {
var arr = this.bodyArr,
len = arr.length;
for (var i = 0; i < len; i++) {
if (i === len - 1) {
this.setHeadXY(arr);
} else {
arr[i].x = arr[i + 1].x;
arr[i].y = arr[i + 1].y;
}
}
this.eatFood(arr);
this.removeSnake();
this.initSnake();
this.headInBody(arr);
},
setHeadXY: function (arr) {
var head = arr[arr.length - 1];
switch (this.dir) {
case 'LEFT':
if (head.x <= 0) {
head.x = wrapW - 20;
} else {
head.x -= 20;
}
break;
case 'RIGHT':
if (head.x >= wrapW - 20) {
head.x = 0;
} else {
head.x += 20;
}
break;
case 'UP':
if (head.y <= 0) {
head.y = wrapH - 20;
} else {
head.y -= 20;
}
break;
case 'DOWN':
if (head.y >= wrapH - 20) {
head.y = 0;
} else {
head.y += 20;
}
break;
default:
break;
}
},
headInBody: function (arr) {
var headX = arr[arr.length - 1].x,
headY = arr[arr.length - 1].y;
for (var i = 0; i < arr.length - 2; i++) {
item = arr[i];
if (headX === item.x && headY === item.y) {
var _self = this;
setTimeout(function () {
alert('游戏结束');
clearInterval(t);
_self.removeSnake();
_self.removeFood();
}, 100)
}
}
},
removeSnake: function () {
var bodys = document.getElementsByClassName('round');
while (bodys.length > 0) {
bodys[0].remove();
}
},
changeDir: function () {
var e = e || window.event;
code = e.keyCode;
this.setDir(code);
},
setDir: function () {
switch (code) {
case 37:
if (this.dir !== 'RIGHT' && this.dir !== 'LEFT') {
this.dir = 'LEFT';
}
break;
case 39:
if (this.dir !== 'RIGHT' && this.dir !== 'LEFT') {
this.dir = 'RIGHT';
}
break;
case 38:
if (this.dir !== 'UP' && this.dir !== 'DOWN') {
this.dir = 'UP';
}
break;
case 40:
if (this.dir !== 'UP' && this.dir !== 'DOWN') {
this.dir = 'DOWN';
}
break;
default:
break;
}
},
createFood: function () {
var food = document.createElement('i');
food.className = 'food';
food.style.left = this.setRandomPos(wrapW) * 20 + 'px';
food.style.top = this.setRandomPos(wrapH) * 20 + 'px';
wrap.appendChild(food);
},
setRandomPos: function (size) {
return Math.floor(Math.random() * (size / 20))
},
eatFood: function (arr) {
var food = document.getElementsByClassName('food')[0],
foodX = getStyles(food, 'left'),
foodY = getStyles(food, 'top'),
headX = arr[arr.length - 1].x,
headY = arr[arr.length - 1].y,
x,
y;
if (headX === foodX && headY === foodY) {
this.removeFood();
this.createFood();
if (arr[0].x == arr[1].x) {
x = arr[0].x;
if (arr[0].y > arr[1].y) {
y = arr[0].y + 20;
} else if (arr[0].y < arr[1].y) {
y = arr[0].y - 20;
}
} else if (arr[0].y === arr[1].y) {
y = arr[0].y;
if (arr[0].x > arr[1].x) {
x = arr[0].x + 20;
} else if (arr[0].x < arr[1].x) {
x = arr[0].x - 20;
}
}
arr.unshift({
x,
y
})
}
},
removeFood: function () {
var food = document.getElementsByClassName('food')[0];
food.remove();
}
}
return new Snake().init();
});