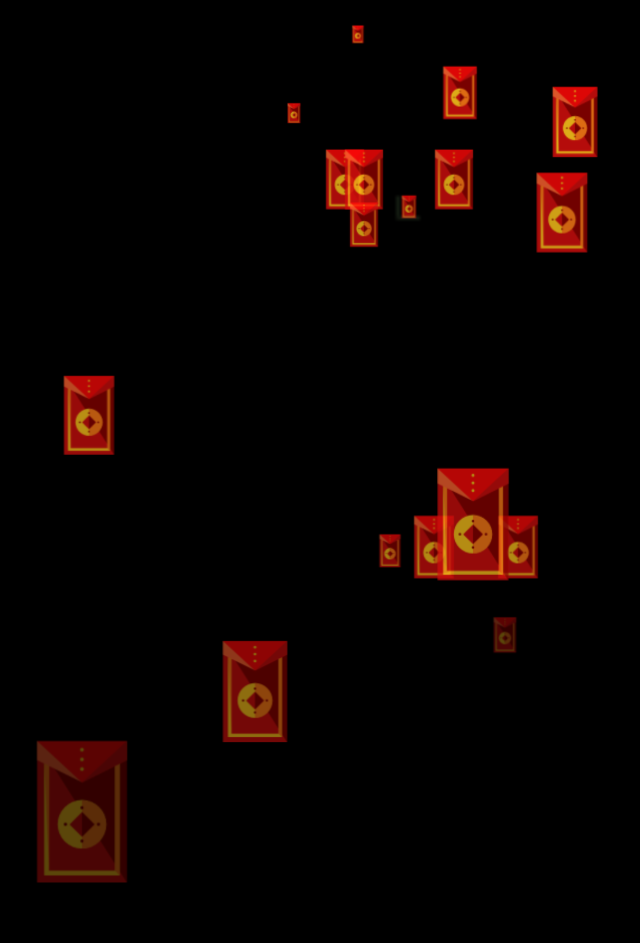
<template>
<view class="page">
<view
v-for="(item, index) in Array(18).fill()"
:key="index"
class="animation span"
:data-animation-position="position()"
:data-animation-delay="delay()"
:data-animation-timing="timing()"
:data-animation-duration="duration()"
:data-animation-name="name()"
><image class="image" src="../../static/bonus.png"
/></view>
</view>
</template>
<script>
export default {
data() {
return {};
},
onReady: function() {},
methods: {
random(min, max) {
return Math.floor(Math.random() * (max - min + 1) + min);
},
position() {
return this.random(1, 100);
},
delay() {
return this.random(1, 4);
},
duration() {
return this.random(4, 8);
},
name() {
return this.random(1, 4);
},
timing() {
return ["linear", "ease", "ease-in", "ease-out", "ease-in-out"][
this.random(0, 4)
];
}
}
};
</script>
<style lang="scss" scoped>
html,
body,
#app {
height: 100%;
background: #000;
}
.page {
height: 100vh;
overflow: hidden;
background: #000;
position: relative;
color: #fff;
.span {
width: 30upx;
height: 30upx;
position: absolute;
top: -100upx;
animation-iteration-count: infinite;
transform-origin: center -30upx;
transform: translate3d(0, 0, 0);
}
.image {
width: 30upx;
height: 30upx;
}
}
// 横向起始位置
@for $i from 1 through 100 {
.animation[data-animation-position="#{$i}"] {
left: #{$i}vw;
}
}
// 降落曲线
$timing: (
linear: linear,
ease: ease,
ease-in: ease-in,
ease-out: ease-out,
ease-in-out: ease-in-out
);
@each $key, $value in $timing {
.animation[data-animation-timing="#{$key}"] {
transition-timing-function: $value;
}
}
// 延时时间
@for $i from 1 through 4 {
.animation[data-animation-delay="#{$i}"] {
animation-delay: #{$i}s;
}
}
// 持续时间
@for $i from 4 through 8 {
.animation[data-animation-duration="#{$i}"] {
animation-duration: #{$i}s;
}
}
// 动画
@for $i from 1 through 4 {
.animation[data-animation-name="#{$i}"] {
animation-name: fall, swing#{$i}, scaleing#{$i};
}
}
// 下落
@keyframes fall {
0% {
top: -80upx;
opacity: 1;
}
50% {
opacity: 0.7;
}
100% {
top: 55vh;
color: red;
opacity: 0.3;
}
}
// 左右摇摆
@for $i from 1 through 4 {
@keyframes swing#{$i} {
25% {
transform: translateX(-#{$i * 20}px);
}
50% {
transform: translateX(#{$i * 20}px);
}
75% {
transform: translateX(-#{$i * 20}px);
}
100% {
transform: translateX(#{$i * 20}px);
}
}
}
// 透视
@for $i from 1 through 4 {
@keyframes scaleing#{$i} {
from {
transform: scale($i*0.6);
}
to {
transform: scale($i * 1.5);
}
}
}
</style>
Note
- 主要是css属性选择器配合自定义data-属性的使用
- css没有随机数,用scss循环生成多个类,用属性选择器去匹配
参考:
https://juejin.im/post/5c4525ab6fb9a049bb7ca45c