002.001 C#也能开发Android应用
准备工作
打开 Visual Studio Installer 安装移动开发套件使用.net的移动开发。
安装好套件后打开 VS 的 Tools - Android SDK Manager 确认 SDK Platform 和 Emulator 安装成功
(第一次使用时要进入Android Device Manager设置机型初始化安卓Emulator)
体验 Android 模拟器
Android SDK 更新完毕后,可以在 Tools - Android SDK Manager - Android Device Manager 直接体验 Android 模拟器(一瞥 8.1)。
补充: 建议选用X86_64 核心减少调试卡顿
开发一个简单的计算器 App
开发安卓应用可以通过创建移动端跨平台项目,也可以通过创建单纯的安卓项目。考虑到 IOS 和 UWP 项目较难调试和发布视频里就只创建了 Android XAML App(Xamarin.Forms) 项目。
下面开发一个简单的加法应用。
VS2019新建项目(与视频内VS2017界面略微不同)
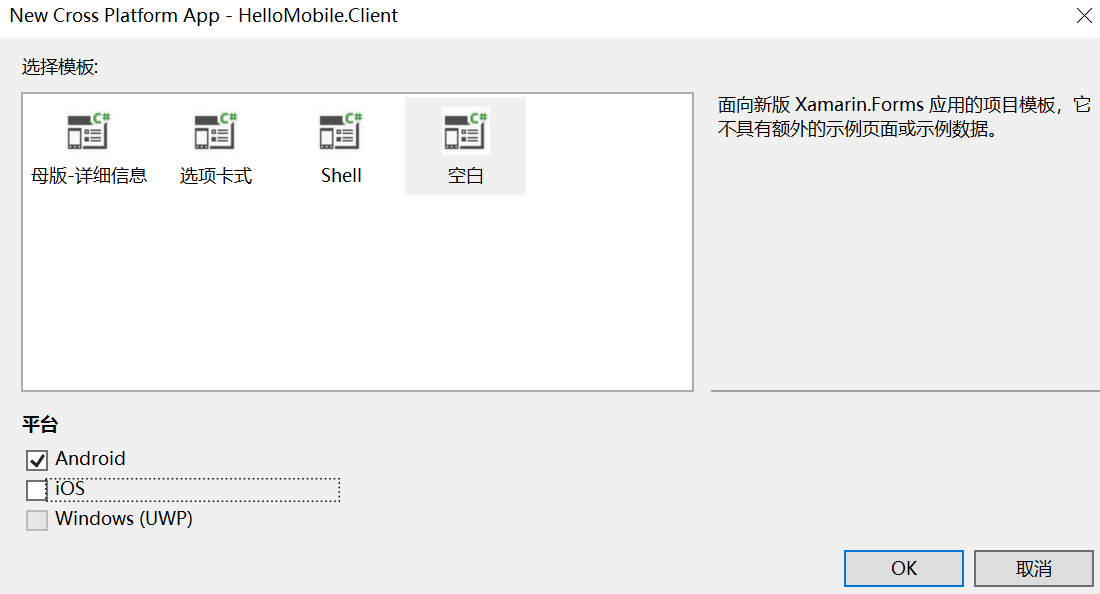
事例代码:
前端 XAML:
<?xml version="1.0" encoding="utf-8" ?>
<ContentPage xmlns="http://xamarin.com/schemas/2014/forms"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
xmlns:local="clr-namespace:HelloMobile.Client"
x:Class="HelloMobile.Client.MainPage">
<Grid>
<Grid.RowDefinitions>
<RowDefinition Height="Auto"/>
<RowDefinition Height="Auto"/>
<RowDefinition Height="Auto"/>
<RowDefinition Height="Auto"/>
</Grid.RowDefinitions>
<Editor x:Name="Tb1" Grid.Row="0"/>
<Editor x:Name="Tb2" Grid.Row="1"/>
<Editor x:Name="Tb3" Grid.Row="2"/>
<Grid Grid.Row="3">
<Grid.ColumnDefinitions>
<ColumnDefinition Width="*"/>
<ColumnDefinition Width="*"/>
<ColumnDefinition Width="*"/>
<ColumnDefinition Width="*"/>
</Grid.ColumnDefinitions>
<Button x:Name="Btn1" Grid.Column="0" Text="Add" Clicked="Doadd"/>
<Button x:Name="Btn2" Grid.Column="1" Text="Sub" Clicked="Dosub"/>
<Button x:Name="Btn3" Grid.Column="2" Text="Mul" Clicked="Domul"/>
<Button x:Name="Btn4" Grid.Column="3" Text="Div" Clicked="Dodiv"/>
</Grid>
</Grid>
</ContentPage>
Ctrl+E+V将当前语句复制到下一行(每次都得按先ctrl再e 然后v的顺序按)
**
后端 C#:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Xamarin.Forms;
namespace HelloMobile.Client
{
// Learn more about making custom code visible in the Xamarin.Forms previewer
// by visiting https://aka.ms/xamarinforms-previewer
[DesignTimeVisible(true)]
public partial class MainPage : ContentPage
{
public MainPage()
{
InitializeComponent();
}
private void Doadd(object sender, EventArgs e)
{
double.TryParse(Tb1.Text, out double x);//TryParse从TB1.Text从字符串转换为双精度浮点型,赋值给x
double.TryParse(Tb2.Text, out double y);
var z = x + y;
Tb3.Text = z.ToString();
}
private void Dosub(object sender, EventArgs e)
{
double.TryParse(Tb1.Text, out double x);
double.TryParse(Tb2.Text, out double y);
var z = x - y;
Tb3.Text = z.ToString();
}
private void Domul(object sender, EventArgs e)
{
double.TryParse(Tb1.Text, out double x);
double.TryParse(Tb2.Text, out double y);
var z = x * y;
Tb3.Text = z.ToString();
}
private void Dodiv(object sender, EventArgs e)
{
double.TryParse(Tb1.Text, out double x);
double.TryParse(Tb2.Text, out double y);
var z = x / y;
Tb3.Text = z.ToString();
}
}
}
注:最好先 Build生成项目,再进行调试
源码工程文件:HelloMobile.zip
打包成 APK
In your toolbar change the project from debug mode to release mode
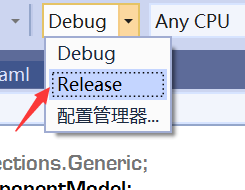
Right-click on your Project and Select Archive(存档)…
右键
- Click on the generated archive and below on the Right side you will find two options Open folder and Distribute. (Select Distribute)
- Then on the Pop-up that appears Select AD-HOC
- Click on the Green plus icon to add signing identity where you need to provide the identity of the signing person or company
- After creating the signing identity click on that identity to select it and then click on save as to save your APK.
- A pop-up will appear asking password for the signing identity which you will produce in step 5.
(输入上一步自己设置的密码)
打包成 APK,安装到手机上的效果:
打包好的apk文件:jisuanqi.zip
其它问题
Android 设计器不工作(VS2019实操未遇到纯备档)
再 XAML 切换到设计器时提示:System.ComponentModel.Composition.ImportCardinalityMismatchException: No exports were found that match the constraint: ...
解决方法:
- 保证 SDK 都更新到了最新版
- 清除 C:\Users\UserName\AppData\Local\Microsoft\VisualStudio\15.0*\ComponentModelCache 里面的缓存文件
推荐扩展读物
深入浅出WPF完整版.pdf
WPF语法与Xamarin有很大一致性,该书也是刘老师所写推荐阅读