面向对象编程模式
对象:独立的存在 或 作为目标的事物
独立性:对象都存在清晰的边界,重点在于划分边界
功能性:对象都能表现出一些功能、操作或行为
交互性:对象之间存在交互,如:运算和继承
万物皆对象
面向对象编程思想
OOP本质是把问题解决抽象为以对象为中心的计算机程序
把对象当作程序的基本单元,对象包含数据和操作数据的函数
面向对象编程 重点在于,高抽象的复用代码
是一种编程方式,并非解决问题的高级方法
面向过程VS面向对象
面向对象三个特征
Python面向对象术语
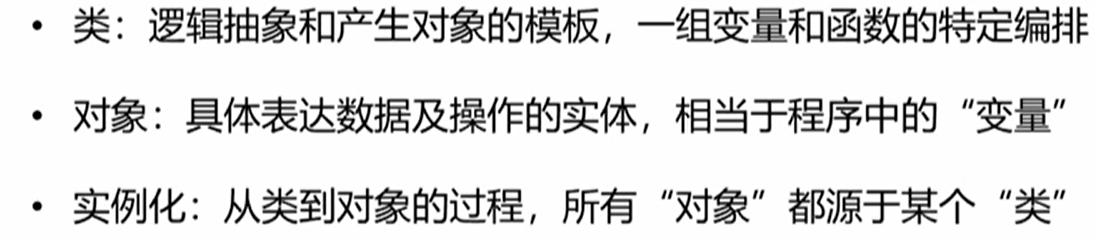<br /> 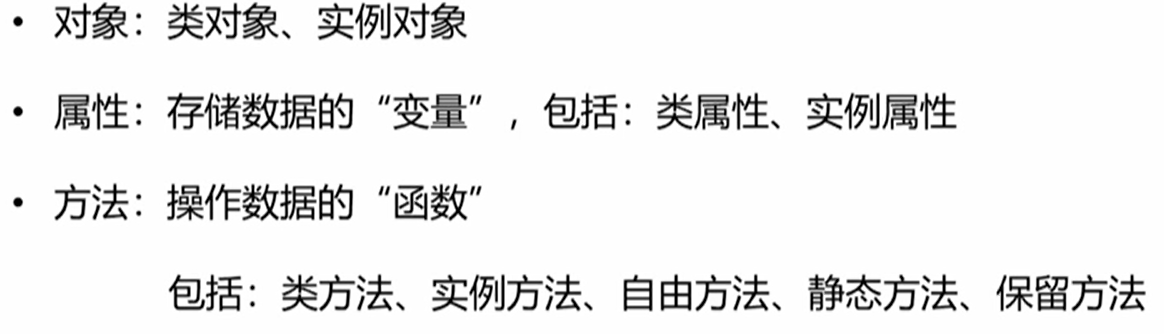<br />类对象VS实例对象:<br />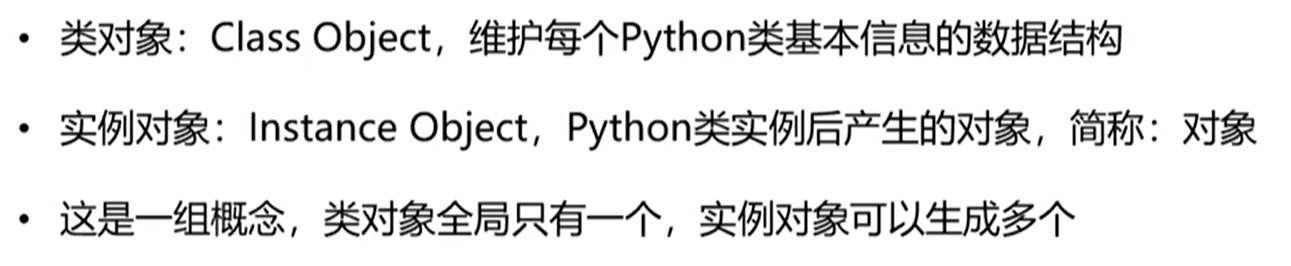<br />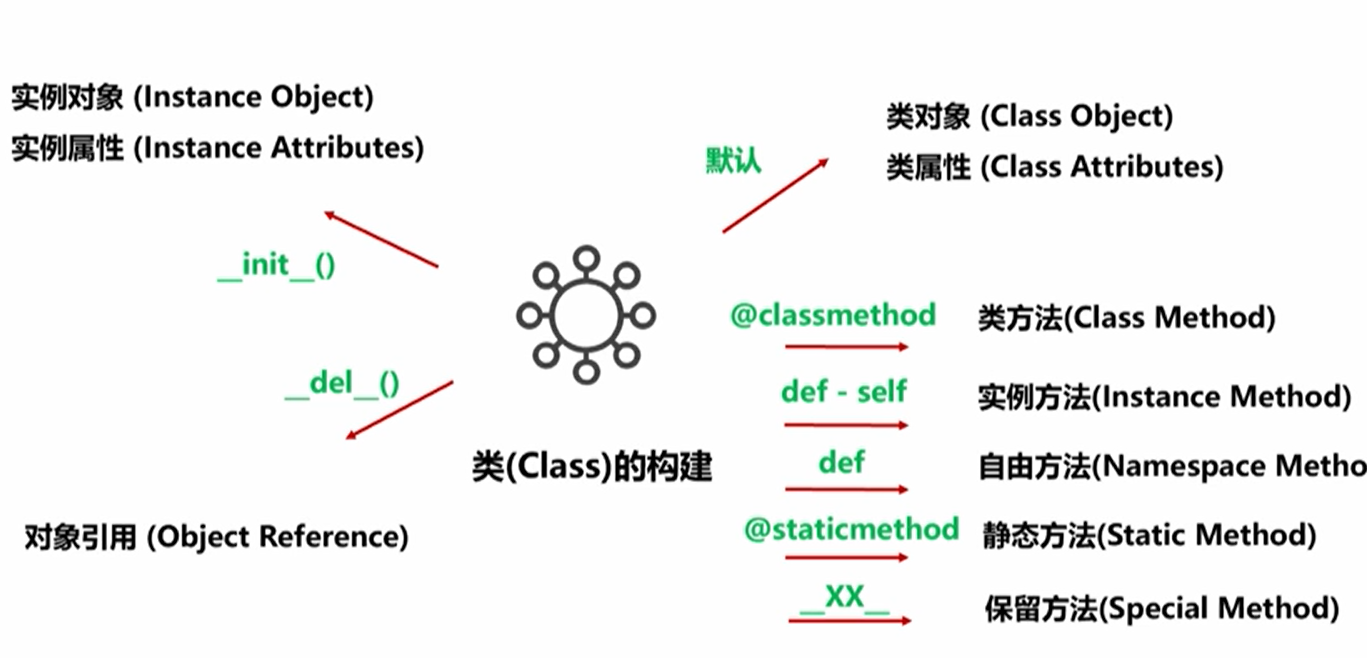
实例
class Product:
def __init__(self,name):
self.name = name
self.label_price = 0
self.real_price = 0
c = Product("电脑")
d = Product("打印机")
e = Product("投影仪")
c.label_price,c.real_price = 10000,8000
d.label_price,d.real_price = 2000,1500
e.label_price,e.real_price = 1500,900
s1,s2 = 0,0
for i in [c,d,e]:
s1 += i.label_price
s2 += i.real_price
print(s1,s2)
Python类的构建
(1)类的基本构建
类对象:
类对象内直接包含的语句会被执行,因此,一般不直接在类定义中直接包含语句
实例对象:
(2)类的构造函数
(3)类的属性
类属性:类对象的属性,由所有实例对象所共享
实例属性:实例对象的属性,由各实例对象所共享
(4)类的方法
- 实例方法:类内部定义的函数,与实例对象相关
- 类方法:与类对象相关的函数,由所有的实例对象共享
- 自由方法:定义在类命名空间中的普通函数
4. 静态方法:定义在类中的普通方法,但可以被类中的所有实例对象共享
#自由方法,静态方法
class DemoClass:
count = 0
def __init__(self,name):
self.name = name
DemoClass.count += 1
@staticmethod
def foo():
DemoClass.count *= 100
return DemoClass.count
dc1 = DemoClass("老王")
print(DemoClass.foo())
print(dc1.foo())
- 保留方法:双下划线开始和结束的方法,保留使用
(5)类的析构函数
实例1
Python类的封装
大概念封装:属性、方法形成类
小概念封装:私有公开对应的私有属性、公开属性、私有方法、公开方法
封装:属性和方法的抽象
属性的抽象:隐藏属性的内在机理
方法的抽象:隐藏方法的内部逻辑
私有属性、公开属性
公开类属性:即类属性
似有类属性:仅供当前类访问,子类不能访问
- 外部不能访问,仅可在类内部被调用
私有实例属性:仅供在当前类内部访问的实例属性,子类亦不能访问
- 只能在类内部被方法所访问
```python
私有类属性、私有实例属性
class DemoClass: count = 0 def init(self,name): self.name = name DemoClass.count += 1 def getName(self): return self.name @classmethod def getCount(cls): return DemoClass.__count
dc1 = DemoClass(“老王”) dc2 = DemoClass(“小诸葛”)
print(DemoClass.count) #不能通过外部直接访问
print(DemoClass.getCount()) #仅可通过特定函数访问 print(dc1.getName(),dc2.getName())#通过方法调用
print(dc1.name,dc2.name)#通过方法调用,直接返回会报错
print(dc1._DemoClass__name)#转换形式强行访问
<a name="xHaCS"></a>
### 私有方法、公开方法
类内部使用的方法<br /> 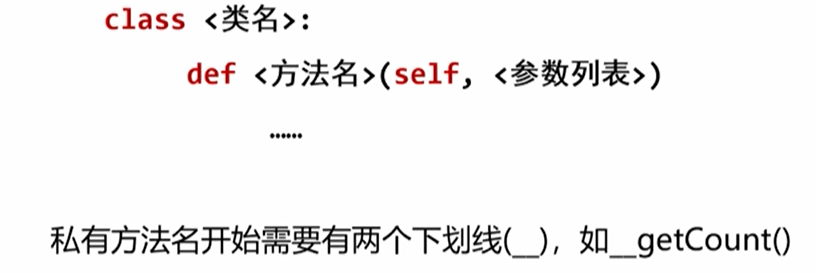
```python
#私有方法、公开方法
class DemoClass:
def __init__(self,name):
self.__name = name
def __getName(self):#变成私有方法
if self.__name != "":
return self.__name
else:
return "老张"
def printName(self):
return "{}同志".format(self.__getName())
dc1 = DemoClass("老王")
dc2 = DemoClass("")
print(dc1.printName(),dc2.printName())
保留属性
保留方法
Python类的继承
(1)继承
代码复用的高级抽象
实现以类为单位的代码抽象
只是一些定义的名字
多继承
(2)类继承的构建
与继承关系判断有关的内置函数
约束
(3)python最基础类
基础类有关的内置函数:
(4)类的属性重载
(5)类的方法重载
(6)类的多继承
- 深度优先:将第一个类及其父类、超类继承完之后
- 从左到右:再继承括号内的第二个类,依次从左到右
```python
函数运行有问题?? — 函数名拼写错误
类的多继承
class DemoClass: def init(self,name): self.name = name def printName(self): return self.name
class NameClass: def init(self,title): self.nick = title def printName(self): return self.nick + “同志同志”
class HumanNameClass(NameClass,DemoClass):
class HumanNameClass(DemoClass,NameClass): pass
dc1 = HumanNameClass(“老王”)
dc1 = NameClass(“老张”)
print(dc1.printName())
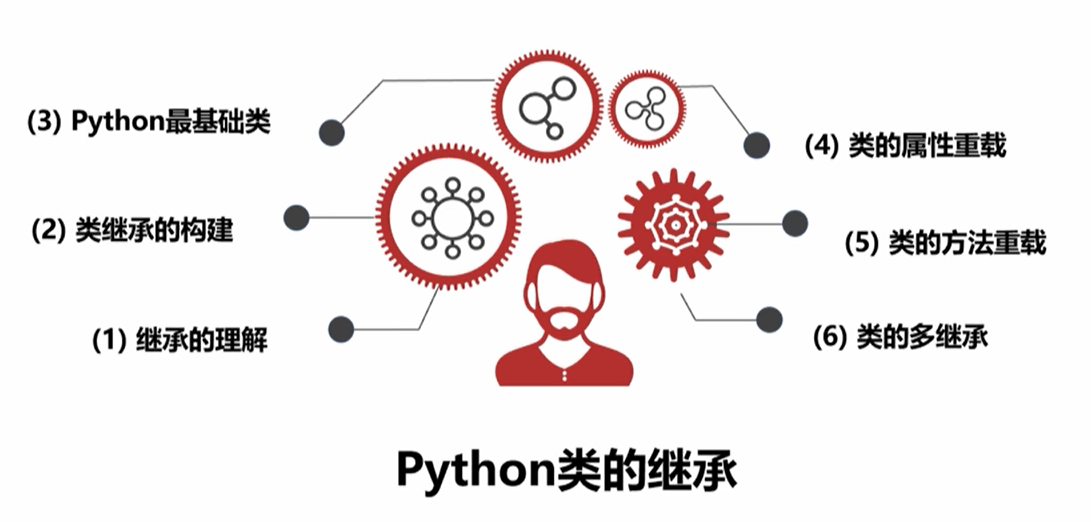
<a name="aXha0"></a>
## Python类运算
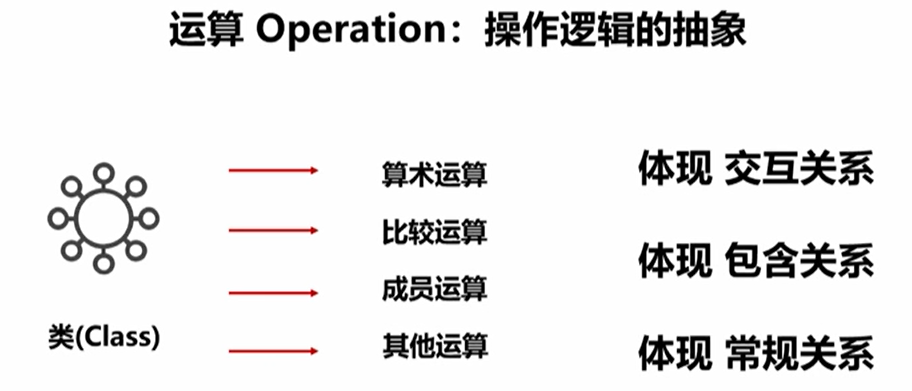<br />**重载限制**:妥协灵活性与功能性<br />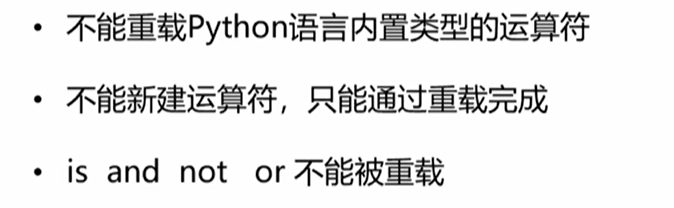
<a name="USpcT"></a>
#### 算数运算重载
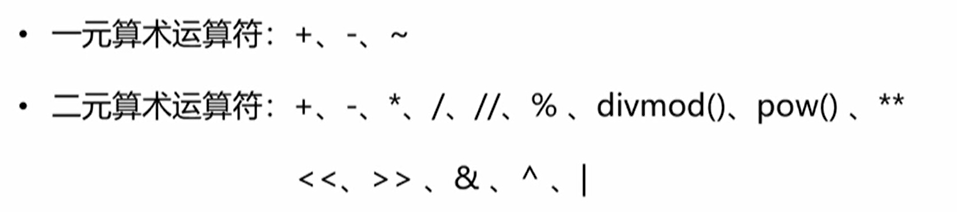
一元运算符重载<br />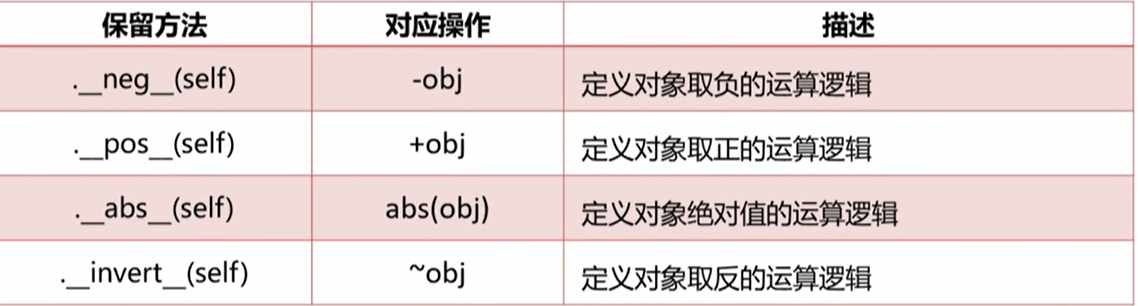<br />二元运算符重载<br />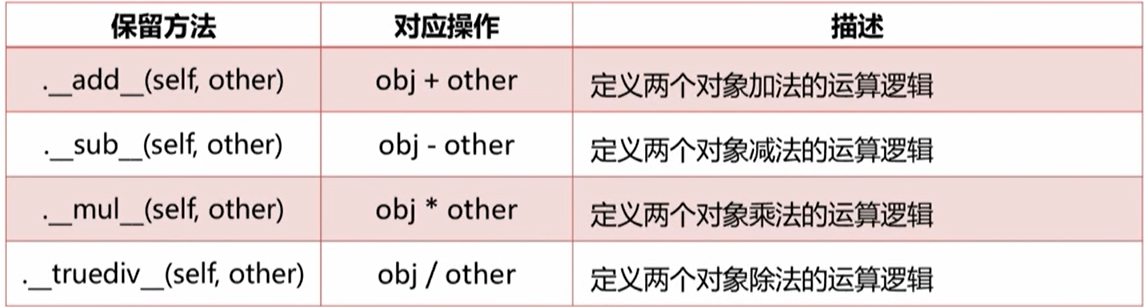<br />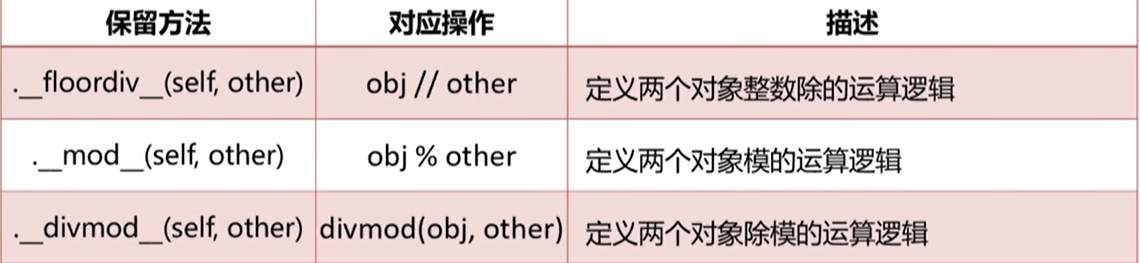<br />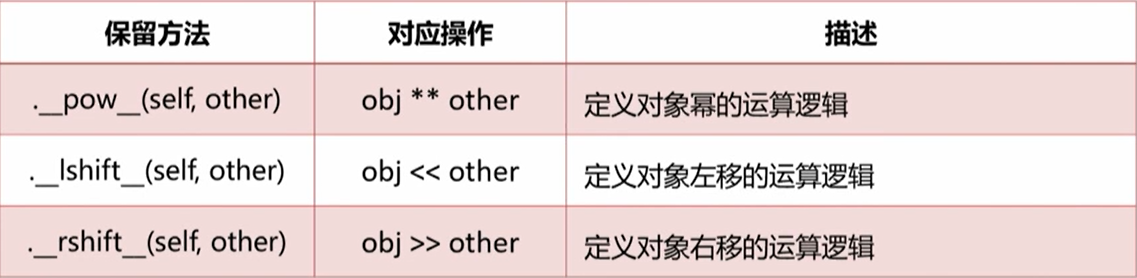<br />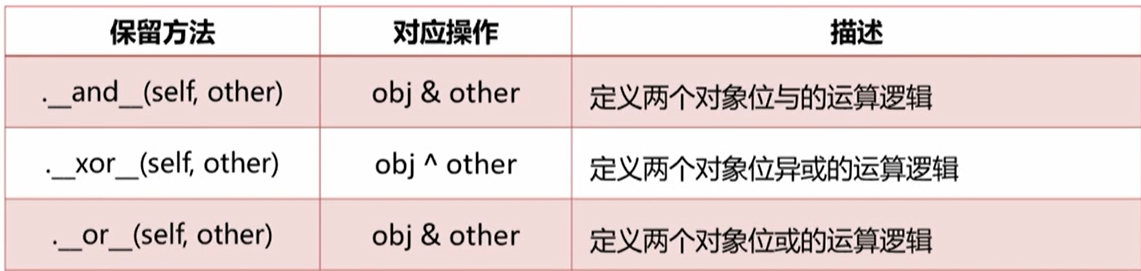
<a name="id3oI"></a>
#### 比较运算重载
<br />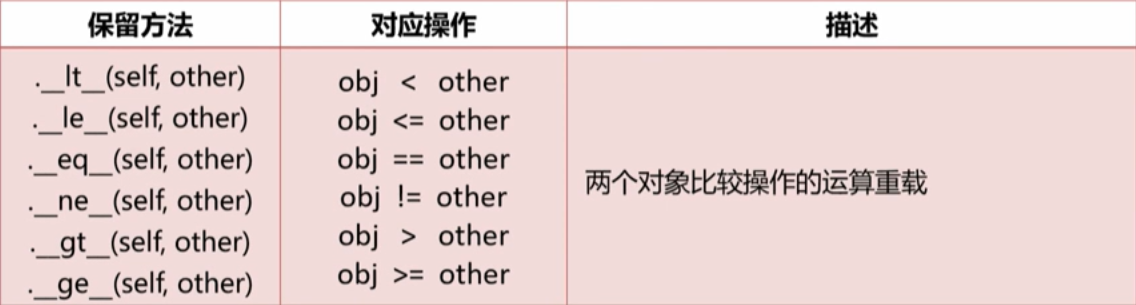
<a name="RFN5w"></a>
#### 成员运算重载
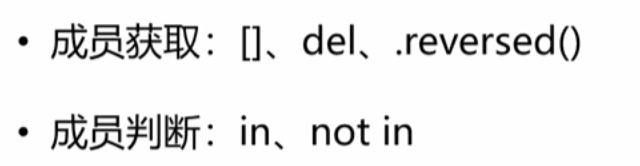<br />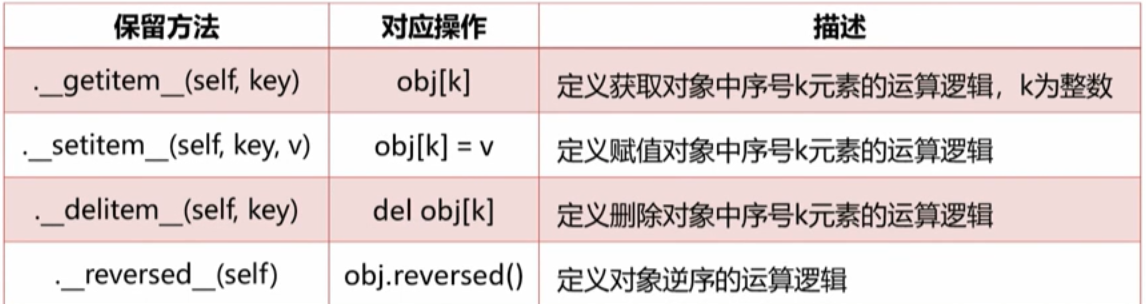<br />
<a name="tkS22"></a>
#### 其他运算重载
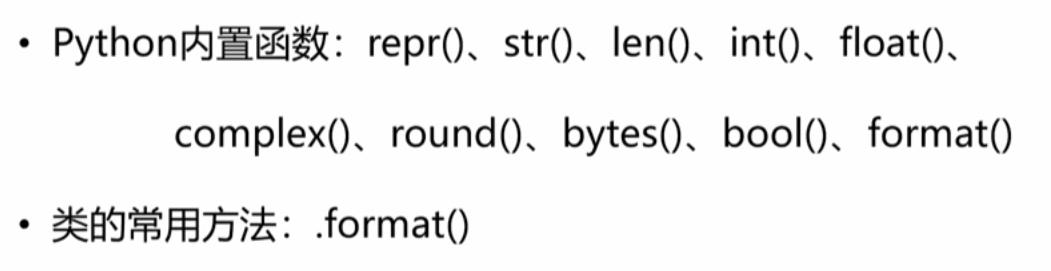<br /> 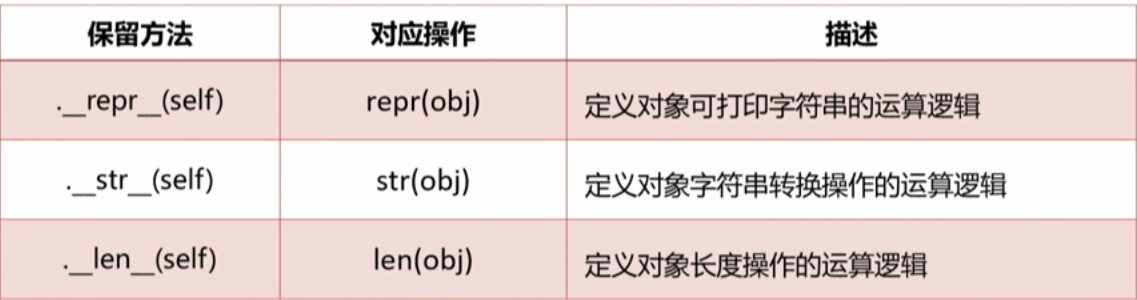<br /> 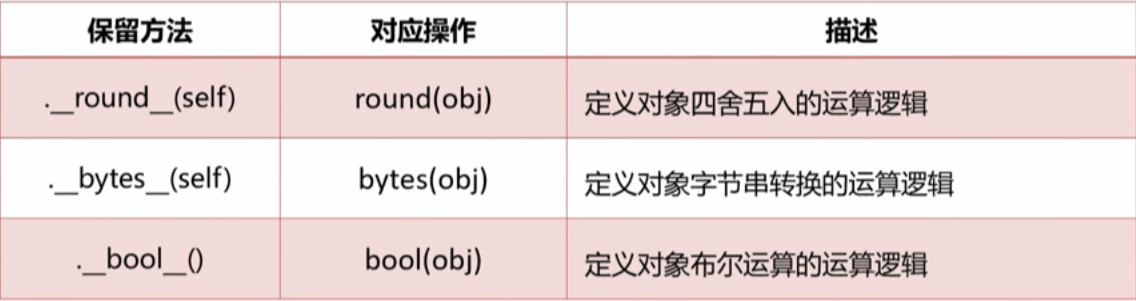<br /> 
<a name="W86Li"></a>
#### code
```python
#算术运算符重载
class NewList(list):
#相加重载
def __add__(self,other):
result = []
for i in range(len(self)):
try:
result.append(self[i] + other[i])
except:
result.append(self[i])
return result
#小于比较重载
def __lt__(self,other):
s,t = 0,0
for c in self:
s += c
for c in other:
t += c
return True if s < t else False
#in判断 重载 继承之前特性并且添加和的值是否在其中的判断
def __contains__(self,item):
s = 0
for c in self:
s += c
if super().__contains__(item) or item == s:
return True
else:
return False
#format重载
def __format__(self,format_spec):
t = []
for c in self:
if type(c) == type("字符串"):
t.append(c)
else:
t.append(str(c))
return ", ".join(t)
ls = NewList([6,2,3,4,5,6])
lt = NewList([1,2,3,99])
print(ls + lt)#加号重载 将各对应位置元素相加
print(ls < lt)#小于比较重载 比较列表元素相加之和的大小
ls = NewList([6,1,2,3])
print(6 in ls,12 in ls)#成员判断重载
print(format([6,1,2,3]))
print(format(ls))#格式化重载
Python类多态
参数类型的多态,参数形式的多态
多态的理解:仅针对方法,方法灵活性的抽象
参数类型的多态:一个方法能够处理多个类型
参数形式的多态:一个方法能够接收多个参数的能力
多态是OPP的一个传统概念,Python天生支持多态(弱类型编程语言?),不需要特殊语法
#多态 参数类型和参数形式的多态
class DemoClass:
def __init__(self,name):
self.name = name
def __id__(self):
return len(self.name)
def lucky(self,salt = 0,more = 9):
s = 0
for c in self.name:
s += (ord(c) + id(salt) + more) % 100
return s
dc1 = DemoClass("老王")
dc2 = DemoClass("老李")
print(dc1.lucky())
print(dc1.lucky(10))
print(dc1.lucky("10"))
print(dc1.lucky(10,100))
print(dc1.lucky(dc2))