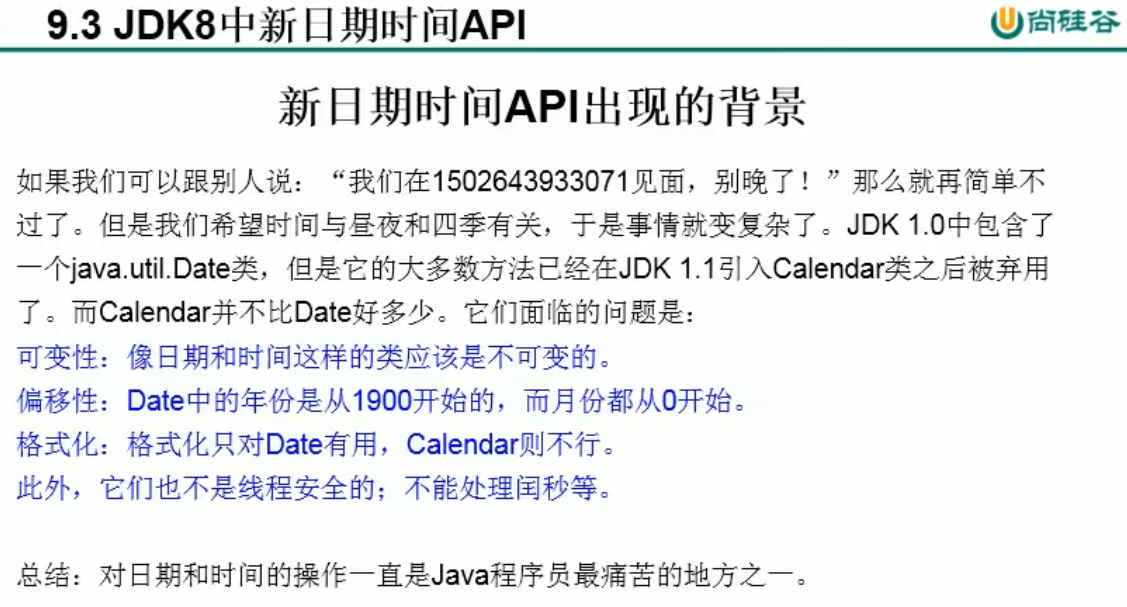

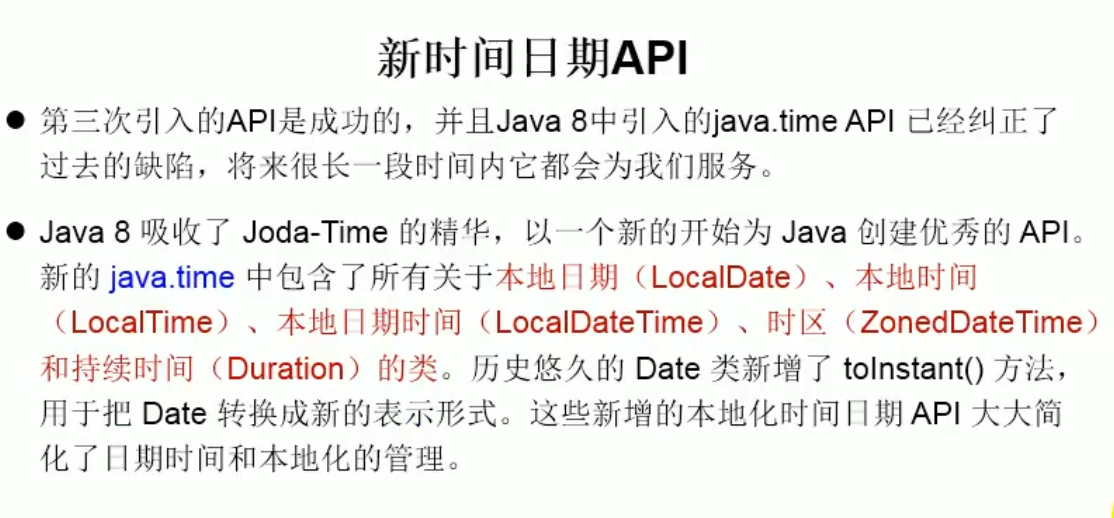
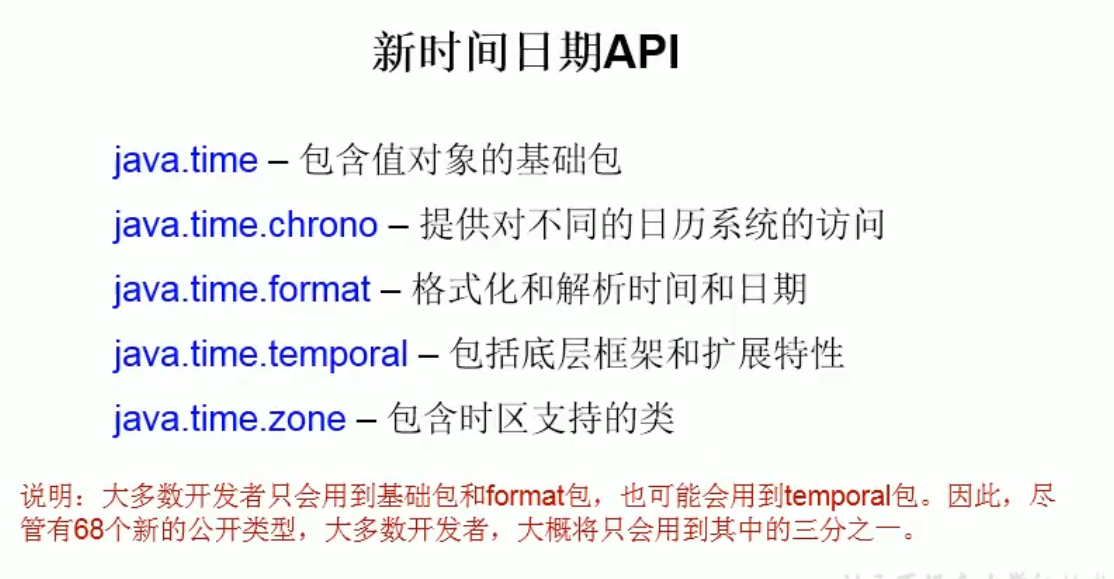
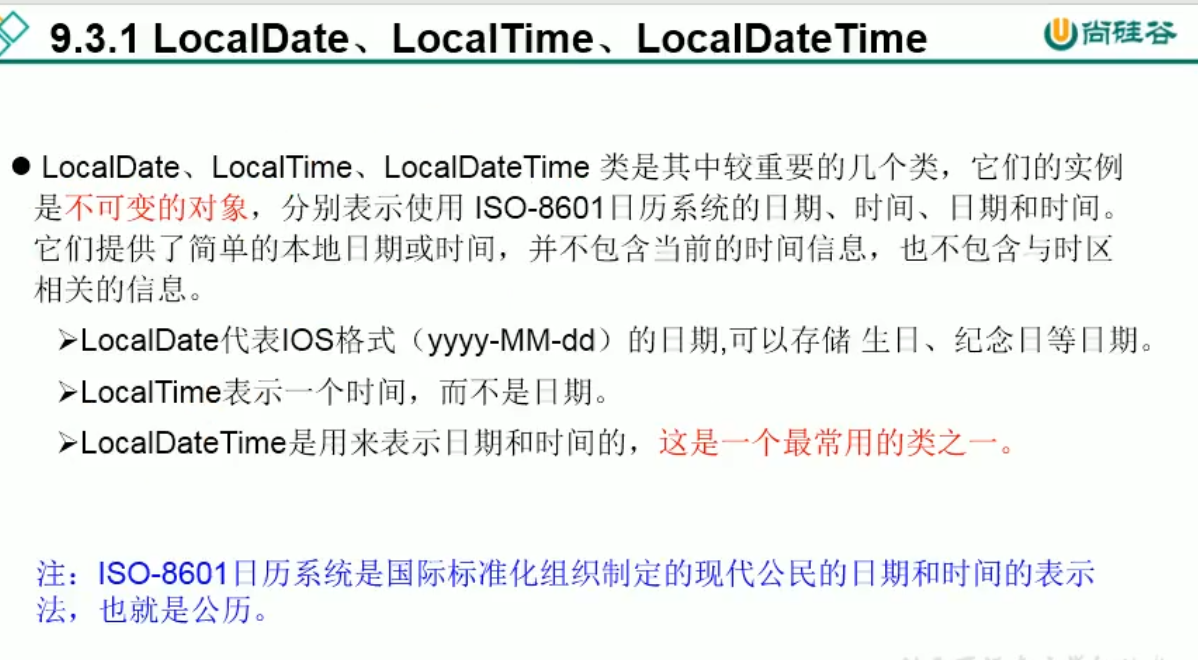
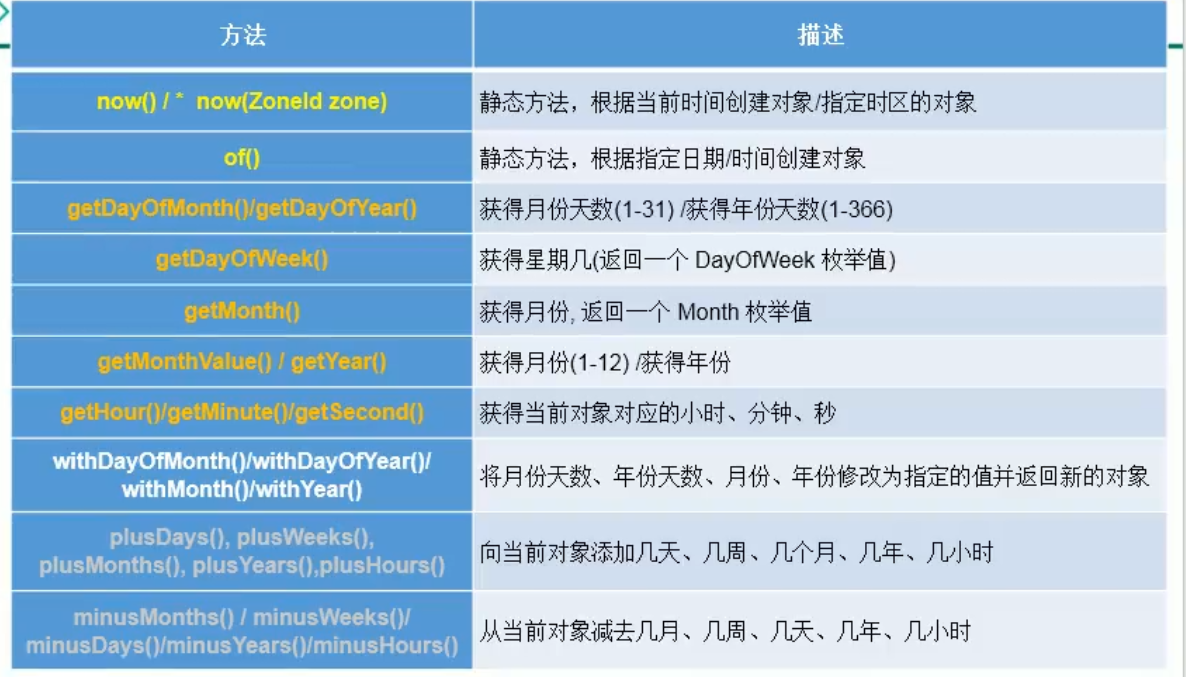
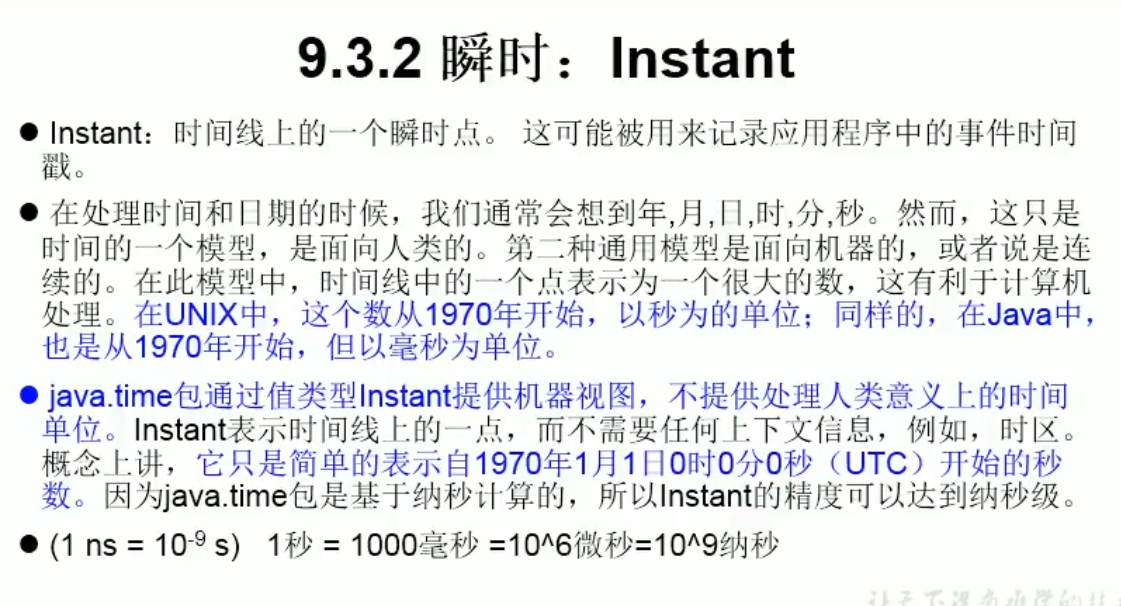
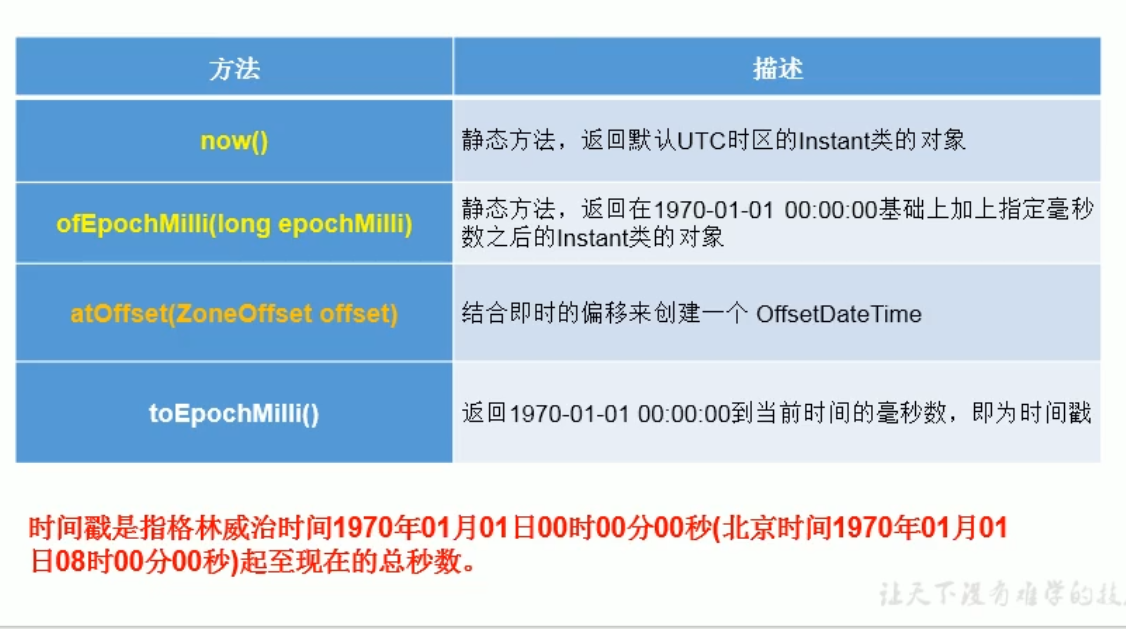
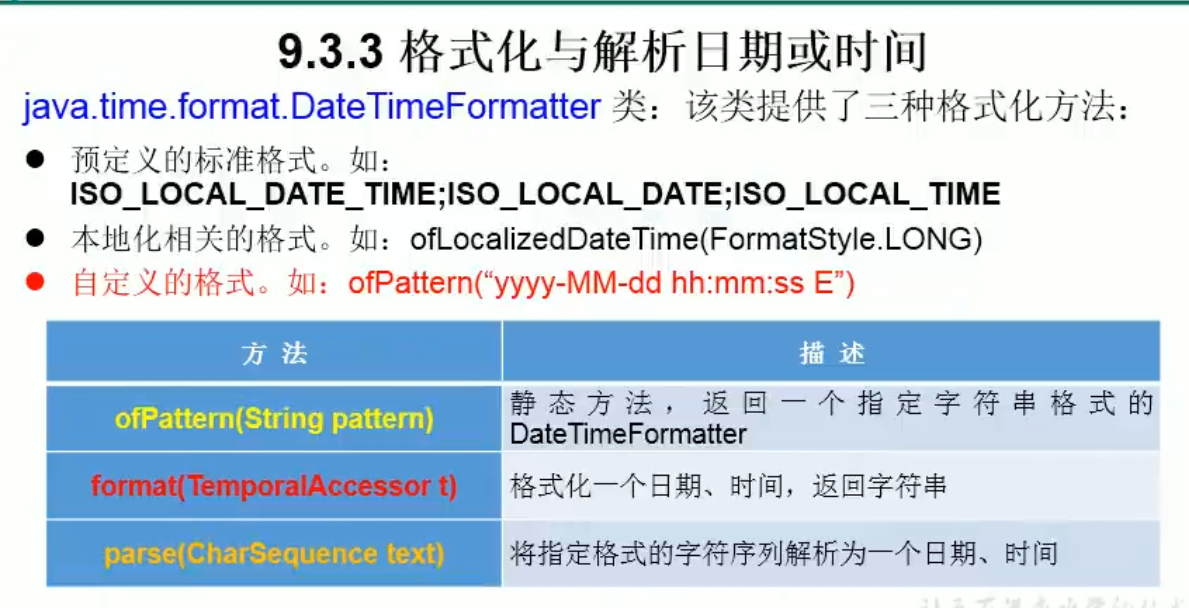
package com.atguigu.java;
import org.junit.Test;
import java.time.*;
import java.time.format.DateTimeFormatter;
import java.time.format.FormatStyle;
import java.time.temporal.TemporalAccessor;
import java.util.Date;
/**
* @author Dxkstart
* @create 2021-05-12 19:52
*/
public class JDK8_DateTimeTest {
@Test
public void testDate(){
//偏移量的问题
// Date date = new Date(2021,05,12);
// System.out.println(date);//Sun Jun 12 00:00:00 CST 3921
//年份不正确的原因是,有1900的偏移量,且月份是从0开始的
Date date = new Date(2021-1900,5-1,12);
System.out.println(date);//Wed May 12 00:00:00 CST 2021
}
/*
LocalDate、 LocalTime、 LocalDateTime 的使用
说明:
1.LocalDateTime相较于其他两个来说,使用频率更高一些
2.类似于Calendar
*/
@Test
public void Test(){
//new():获取当前的日期、时间、日期+时间
LocalDate localeDate = LocalDate.now();
LocalTime localTime = LocalTime.now();
LocalDateTime localDateTime = LocalDateTime.now();
System.out.println(localeDate);
System.out.println(localTime);
System.out.println(localDateTime);
//of():设置指定的年、月、日、时、分、秒。没有偏移量
LocalDateTime localDateTime1 = LocalDateTime.of(2021, 6, 7, 12, 00, 59);
System.out.println(localDateTime1);
//getXxx():获取相关的属性
System.out.println(localDateTime.getDayOfMonth());
System.out.println(localDateTime.getDayOfWeek());
System.out.println(localDateTime.getDayOfYear());
//体现不可变性
//withXxx():设置相关的属性
LocalDateTime localDateTime2 = localDateTime.withDayOfMonth(22);
System.out.println(localDateTime);//12日
System.out.println(localDateTime2);//22日
//不变性,+加操作
LocalDateTime localDateTime3 = localDateTime.plusMonths(5);
System.out.println(localDateTime);//5月
System.out.println(localDateTime3);//10月
//减操作,—
LocalDateTime localDateTime4 = localDateTime.minusMonths(3);
System.out.println(localDateTime);//5月
System.out.println(localDateTime4);//2月
}
/*
Instant的使用
*/
@Test
public void test2(){
//now():获取本初子午线对应的标准时间
Instant instant = Instant.now();
System.out.println(instant);//2021-05-12T12:49:24.778Z,这是伦敦时间,1时区
//添加偏移量
OffsetDateTime offsetDateTime = instant.atOffset(ZoneOffset.ofHours(8));
System.out.println(offsetDateTime);
//toEpochMilli():获取自1970年1月1日0时0分0秒(UTC)开始的毫秒数 --->Date类的getTime()
long toEpochMilli = instant.toEpochMilli();
System.out.println(toEpochMilli);
//toEpochMilli():通过给定的毫秒数,获取Instant实例 --->Date(long millis)
Instant instant1 = Instant.ofEpochMilli(1520824202970L);
System.out.println(instant1);
}
/*
DateTimeFormatter:格式化或解析日期、时间
类似于SimpleDateFormat
*/
@Test
public void test3(){
//方式一:预定义的标准格式。如:ISO_LOCAL_DATE_TIME;ISO_LOCAL_DATE;ISO_LOCAL_TIME
DateTimeFormatter formatter = DateTimeFormatter.ISO_LOCAL_DATE_TIME;
//格式化:日期 -->字符串
LocalDateTime localDateTime = LocalDateTime.now();
String str1 = formatter.format(localDateTime);
System.out.println(localDateTime);
System.out.println(str1);//2021-05-12T21:31:17.246
//解析:字符串 -->日期
TemporalAccessor parse = formatter.parse("2021-05-12T21:31:17.246");
System.out.println(parse);
//方式二:
// 本地化相关的格式。如:ofLocalizedDateTime(FormatStyle.LONG)
// FormatStyle.LONG / FormatStyle.MEDIUM / FormatStyle.SHORT :适用于LocalDateTime
DateTimeFormatter formatter1 = DateTimeFormatter.ofLocalizedDateTime(FormatStyle.SHORT);
//格式化
String str2 = formatter1.format(localDateTime);
System.out.println(str2);//21-5-12 下午9:42
//本地化相关的格式。如:ofLocalizedDate()
// FormatStyle.FULL / FormatStyle.LONG / FormatStyle.MEDIUM / FormatStyle.SHORT:
//适用于:LocalDate
DateTimeFormatter formatter2 = DateTimeFormatter.ofLocalizedDate(FormatStyle.FULL);
String str3 = formatter2.format(LocalDate.now());
System.out.println(str3);//2021年5月12日 星期三
//重点:
//方式三:自定义的格式。如:ofPattern("yyyy-MM-dd hh:mm:ss ")
DateTimeFormatter formatter3 = DateTimeFormatter.ofPattern("yyyy-MM-dd hh:mm:ss");
//格式化
String str4 = formatter3.format(LocalDateTime.now());
System.out.println(str4);//2021-05-12 10:00:27
//解析
TemporalAccessor parse1 = formatter3.parse("2021-05-12 10:00:27");
System.out.println(parse1);
}
}
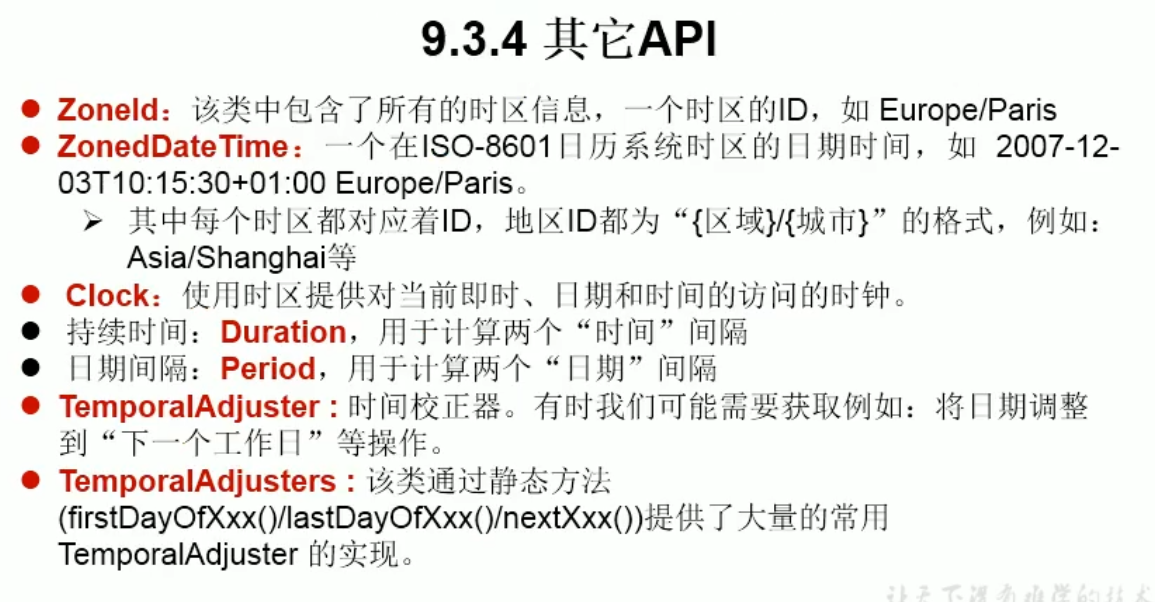